Question
Find n-th prime number in MIPS Hi, I was given this C++ program and I need to finish the MIPS function FindNthPrime and is_prime below.
Find n-th prime number in MIPS
Hi, I was given this C++ program and I need to finish the MIPS function FindNthPrime and is_prime below. I have tried by myself, but I always confuse on function calling and registers on MIPS. Would you help me to solve this problem or give me some hints to do this, thank you
#------- Data Segment ----------
.data
# Define the string messages and the primes array
mesg1: .asciiz "Please enter an integer number(range: 1~100, enter 0 to end the program):"
mesg2: .asciiz "-th prime is:"
mesg3: .asciiz "Sorry, input range should be 1~100. "
newline: .asciiz " "
primes: .word 0:100
#------- Text Segment ----------
.text
.globl main
main:
# Put 2 as the 1st element in primes array
addi $t1, $zero, 2
la $t2, primes
sw $t1, 0($t2)
ask_input:
# Print starting message to ask input
la $a0 mesg1
li $v0 4
syscall
# $s0 stores the input integer
li $v0, 5
syscall
addi $s0, $v0, 0
# if input integer is 0, then end the program
beq $s0, $zero, endmain
# if input integer is larger than 100, then ask another input
addi $t1, $zero, 101
slt $t2, $s0, $t1
bne $t2, $zero, start
la $a0 mesg3
li $v0 4
syscall
j ask_input
start:
# Otherwise, call nth_prime procedure to calculate n-th prime, $a0=n
addi $a0, $s0, 0
jal FindNthPrime # FindNthPrime is what you should implement
# $t0 stores the calculated n-th prime
addi $t0, $v0, 0
# Print result message and result of n-th prime
addi $a0, $s0, 0
li $v0, 1
syscall
la $a0, mesg2
li $v0, 4
syscall
addi $a0, $t0, 0
li $v0, 1
syscall
la $a0, newline
li $v0, 4
syscall
# Another loop
j ask_input
# Terminate the program
endmain:
li $v0, 10
syscall
# Implement function FindNthPrime
# What FindNthPrime should do: calculate n-th prime, $a0=n, return n-th prime in $v0
FindNthPrime:
# TODO below T$T2
# TODO above
# Implement function is_prime, which would be used in FindNthPrime function
# What is_prime should do: Given a value in $a0, check whether it is a prime
# if $a0 is prime, then return $v0=1, otherwise, return $v0=0
is_prime:
# TODO below
# TODO above
# Given value in $a1 and $a2, this function calculate the remainder of $a1 / $a2
# return remainder($a1 % $a2) in $v1
Remainder:
addi $sp, $sp, -4
sw $ra, 0($sp)
div $a1, $a2
mfhi $v1
lw $ra, 0($sp)
addi $sp, $sp, 4
jr $ra
Question 2: Find n-th prime (20 marks) The following C++ program is to get an input value n, then output the n-th prime. Translate FindNthPrime() function into a MIPS procedure. The translation doesn't need to be 100% identical but should follow the same algorithm as described The program keeps the prime numbers in the array primes calculated by the previous call (s) to the function FindNthPrime in the array primes. Given an input value n, the program first checks the n-th position of primes array, if n-th prime has already been stored in it (i.e. that cell has a non-zero value), the prime will be outputted directly. Otherwise, the program call FindNthPrime function to find next prime until the n-th prime is found. All the newly found primes will be stored in array primes for future use (now primes contains the first n prime numbers). For simplicity, the input value would be in range 0, 100] A prime number (or a prime) is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. To check whether a given value n is prime, the program adopts the most intuitive method, which divides n by each integer from 2 to + 1. If the remainders of every division aren't zeros, then n is a prime. The following MIPS instructions are used by Remainder Function in MIPS program. Refer to NthPrime.asm for more details. Read comments in skeleton and follow its instructions to implement n-th prime finding algorithm div $s, $t #Divides $s by $t and stores the quotient in $1.0 and the remainder in $HI mfhi $d #The contents of register HI are moved to the specified register You should submit the solution using the original name NthPrime.asm, do not change the file name and do not change the code other than adding the TODOs. #includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
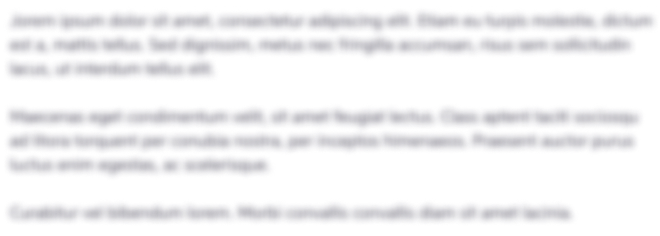
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started