Question
Finishing java code. Program should read from infix text file and convert the numbers from infix to postfix notation and then give its value. The
Finishing java code. Program should read from infix text file and convert the numbers from infix to postfix notation and then give its value. The program should read from the infix text file and output the results to another text file called csis.txt. This last part is where im having difficulty coding please help with updated and working java program. The infix.txt should have the following numbers to be converted and return its value.
8 + 4 * 2 - 6
7 * 2 - 4 * 3 + 2 * 5
2 * 3 * 4 - 8 + 9 / 3 / 3
5 + 7 * 4 - 6
4 * ( 3 + 2 * 4 ) - 7
( 5 + 7 ) / ( 9 - 5 )
3 * ( 5 * ( 5 - 2 ) ) - 9
( ( 5 * ( 4 + 2 ) - ( 8 + 8 ) / 2 ) - 9 ) ^ 3
( ( 5 + 5 * ( 6 - 2 ) + 4 ^ 2 ) * 8 )
( ( ( 3 ^ 4 ) ) )
The code i have is the folowing:
// Driver class
import java.io.*;
import java.util.*;
public class Driver {
public static void main(String[]args) throws IOException
{
Scanner fileScan = new Scanner(new File("infix.txt"));
InfixToPostfix infixToPostfix = new InfixToPostfix();
EvalPostfix evalPostfix = new EvalPostfix();
{
try {
File file = new File("infix.txt");
if (file.createNewFile()){
System.out.println("File is created!");
}else{
System.out.println("File already exists.");
}
} catch (IOException e) {
e.printStackTrace();
}
}
while(fileScan.hasNextLine())
{
String infix = fileScan.nextLine();
String postfix = infixToPostfix.convertToPostfix(infix);
String result = evalPostfix.evalPostfix(postfix);
System.out.println("Infix: "+ infix);
System.out.println("Postfix: "+ postfix);
System.out.println("Result: "+ result);
System.out.println();
}
}
}
// Object Stack
public class ObjectStack implements ObjectStackInterface {
private Object[] item;
private int top;
public ObjectStack()
{
item = new Object[1];
top = -1;
}
public boolean isEmpty()
{
return top == -1;
}
public boolean isFull()
{
return top == item.length -1;
}
public void clear()
{
item = new Object[1];
top = -1;
}
public void push(Object o)
{
if(isFull())
resize(2 * item.length);
item[++top] = o;
}
private void resize(int size)
{
Object[] temp = new Object[size];
for(int i = 0;i <=top;i++)
temp[i] = item[i];
item = temp;
}
public Object pop()
{
if(isEmpty())
{
System.out.println("Stack Underflow");
System.exit(1);
}
Object temp = item[top--];
if(top == item.length/4)
resize(item.length/2);
return temp;
}
public Object top()
{
if(isEmpty())
{
System.out.println("Stack Underflow");
System.exit(1);
}
return item[top];
}
}
// ObjectStack Interface
import java.io.File;
import java.io.IOException;
public interface ObjectStackInterface {
public boolean isEmpty();
public boolean isFull();
public void clear();
public void push(Object o);
public Object pop();
public Object top();
}
// EvalPostfix
public class EvalPostfix {
private ObjectStack objStack;
public EvalPostfix()
{
objStack = new ObjectStack();
}
public String evalPostfix(String postfix)
{
int op1 = 0, op2 = 0;
int result = 0;
String str = null;
String[] tokens = postfix.split(" ");
for(int i = 0; i < tokens.length; i++)
{
str = tokens[i];
if(!("+".equals(str) || "-".equals(str) || "*".equals(str) || "/".equals(str) || "^".equals(str)))
{
objStack.push(str);
}
else
{
op1 = Integer.valueOf((String) objStack.pop());
op2 = Integer.valueOf((String) objStack.pop());
switch(str)
{
case "+":
result = (op2 + op1);
break;
case "-":
result = (op2 - op1);
break;
case "*":
result = (op2 * op1);
break;
case "/":
result = (op2 / op1);
break;
case "^":
result = (int) Math.pow(op2, op1);
break;
}
objStack.push("" + result);
}
}
String val = (String) objStack.pop();
return val;
}
}
// Infix to Postfix
public class InfixToPostfix {
private ObjectStack objStack;
public InfixToPostfix()
{
objStack = new ObjectStack();
}
public String convertToPostfix(String infix)
{
String[] tokens = infix.split(" ");
String postfix = "";
for(int i = 0; i < tokens.length; i++)
{
char ch = tokens[i].charAt(0);
if(ch <= '9' && ch >= '0')
{
postfix += ch + " ";
}
else if(ch == '^' || ch == '/' || ch == '*' || ch == '+' || ch == '-' || ch == '(')
{
while(!objStack.isEmpty() && (getPriority(ch) <= getPriority((Character) objStack.top())) && (ch != '('))
{
postfix += objStack.pop() + " ";
}
objStack.push(ch);
}
else if(ch == ')')
{
while((!(objStack.top().equals('('))))
{
postfix += objStack.pop() + " ";
}
objStack.pop();
}
}
while(!objStack.isEmpty())
{
postfix += objStack.pop() + " ";
}
return postfix;
}
private int getPriority(char ch)
{
switch(ch)
{
case '^':
return 3;
case '/':
case '*':
return 2;
case '+':
case '-':
return 1;
default:
return 0;
}
}
}
Step by Step Solution
3.41 Rating (148 Votes )
There are 3 Steps involved in it
Step: 1
Program code Driverjava import javaio import javautil import javaioFile import javaioIOException imp...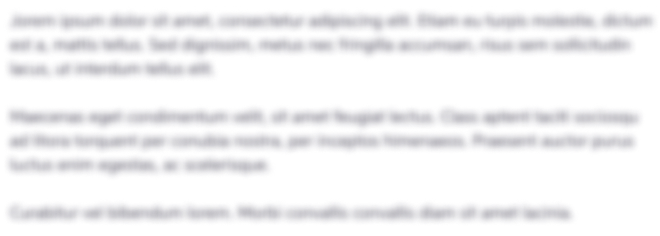
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started