Question
Fix code errors when running juketester.java Juketester.java public class JukeTester { public static void main(String[] args) { Record testRecord; Jukebox myJukebox = new Jukebox(); myJukebox.insert(new
Fix code errors when running juketester.java
Juketester.java
public class JukeTester { public static void main(String[] args) {
Record testRecord;
Jukebox myJukebox = new Jukebox();
myJukebox.insert(new Record()); myJukebox.insert(new Record("Kokomo", "The Beach Boys")); myJukebox.insert(new Record("Wooly Bully", "Sam the Sham & the Pharaohs")); myJukebox.insert(new Record("Mustang Sally", "Wilson Pickett")); myJukebox.insert(1, new Record( "Great Balls of Fire", "Jerry Lee Lewis")); myJukebox.insert(0, new Record("I Feel Good", "James Brown"));
System.out.println("Jukebox now contains: " + myJukebox);
System.out.println(" A random Record is: " + myJukebox.random()); System.out.println("A random Record is: " + myJukebox.random()); System.out.println("A random Record is: " + myJukebox.random()); System.out.println("A random Record is: " + myJukebox.random()); System.out.println("A random Record is: " + myJukebox.random());
testRecord = new Record("Kokomo", "The Beach Boys"); System.out.println(" Does it contain " + testRecord + "?: " + myJukebox.contains(testRecord));
testRecord = new Record("Mustang Sally", "The Commitments"); System.out.println("Does it contain " + testRecord + "?: " + myJukebox.contains(testRecord));
testRecord = new Record("Its Too Late", "Wilson Pickett"); System.out.println("Does it contain " + testRecord + "?: " + myJukebox.contains(testRecord));
testRecord = new Record("Great Balls of Fire", "Jerry Lee Lewis"); System.out.println(" What slot is " + testRecord + " in?: " + myJukebox.findSlot(testRecord));
testRecord = new Record("The Great Pretender", "The Platters"); System.out.println("What slot is " + testRecord + " in?: " + myJukebox.findSlot(testRecord));
testRecord = new Record("Wooly Bully", "Sam the Sham & the Pharaohs"); System.out.println(" Did it successfully remove " + testRecord + "?: "+ myJukebox.remove(testRecord));
testRecord = new Record("Heartbreak Hotel", "Elvis Presley"); System.out.println("Did it successfully remove " + testRecord + "?: " +myJukebox.remove(testRecord));
System.out.println(" Jukebox now contains: " + myJukebox); } }
JukeInterface.java
public interface JukeInterface {
public void insert(Record aRecord);
public void insert(int slot, Record aRecord);
public boolean remove(Record aRecord);
public boolean contains(Record aRecord);
public int findSlot(Record aRecord); (int)(Math.random()*theSize) public Record random();
public String toString(); }
Record.java
public class Record { private String title; private String artist;
public Record( String theTitle, String theArtist ) { this.title = theTitle; this.artist = theArtist; }
public boolean equals( Object currentOb ) { if ( this.getClass() != currentOb.getClass() ) return false; if ( currentOb == null ) return false; Record aRecord2 = (Record)currentOb; // Must typecast the object
return ( this.title.equals( aRecord2.title ) && this.artist.equals( aRecord2.artist )); }
public String toString() { return "( " + this.title + ", " + this.artist + " )"; } }
JukeBox.java
======================================
Class: Jukebox
======================================
import java.util.ArrayList; import java.util.Random;
//Class: Jukebox implemeting the interface JukeInterface public class Jukebox implements JukeInterface { //private instance field records, which is an arraylist of Record private ArrayList
@Override //insert aRecord into the records arraylist at the given slot public void insert(int slot, Record aRecord) { records.add(slot, aRecord);//calling arraylist's add method to add element at given index. //subsequent element will be shifted one position to the right }
@Override //remove aRecord from records //return true if removal is successful, false otherwise public boolean remove(Record aRecord) { return records.remove(aRecord); }
@Override //this method will check whether aRecord exists in the records arraylist or not //return true if contains, false otherwise public boolean contains(Record aRecord) { return records.contains(aRecord); }
@Override //find index of given aRecord in the records array list and return it public int findSlot(Record aRecord) { return records.indexOf(aRecord); }
@Override //return a random Record from the array list public Record random() { Random r = new Random(); //this will give any index from 0 to records.size()-1 int randomIndex = r.nextInt(records.size()); return records.get(randomIndex);//get the record at randomIndex }
@Override //String representation of the Jukebox public String toString() { return records.toString(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
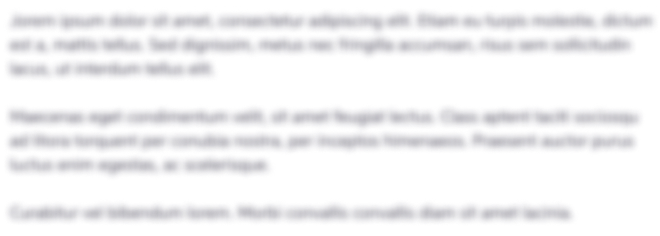
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started