Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Fix evaluateExceptionWhenExprContainsTooFewOperands I am working to write an Evaluates the given postfix expression with help of stack. I am nearly done but one of my
Fix evaluateExceptionWhenExprContainsTooFewOperands
I am working to write an Evaluates the given postfix expression with help of stack. I am nearly done but one of my test does not go through. Can someone help me? BTW i cant add new methods. The rest of the code is : https://www.chegg.com/homework-help/questions-and-answers/help-understand-top-method-work-stacktestjava-get-java-cannot-find-symbol-symbol-method-to-q91574583
import java.util.Stack; /** * The Postfix class implements an evaluator for integer postfix expressions. * * Postfix notation is a simple way to define and write arithmetic expressions * without the need for parentheses or priority rules. For example, the postfix * expression "1 2 - 3 4 + *" corresponds to the ordinary infix expression * "(1 - 2) * (3 + 4)". The expressions may contain decimal 32-bit integer * operands and the four operators +, -, *, and /. Operators and operands must * be separated by whitespace. */ public class Postfix { public static class ExpressionException extends Exception { public ExpressionException(String message) { super(message); } } /** * Evaluates the given postfix expression. * * @param expr Arithmetic expression in postfix notation * @return The value of the evaluated expression * @throws ExpressionException if the expression is wrong */ public static int evaluate(String expr) throws ExpressionException { expr = expr.trim(); //Split string into tokens. String[] tokens = expr.split("\\s+"); if(expr.matches("(\\+)|(\\-)|(\\*)|(\\/)")){ throw new ExpressionException(""); } Stackstack = new Stack (); for(String token: tokens) { if(isInteger(token)) { int number = Integer.parseInt(token); stack.push(number); } else if(isOperator(token)) { String operator = token; int b = stack.pop(); int a = stack.pop(); switch(operator) { case "+": stack.push(a+b); break; case "-": stack.push(a-b); break; case "*": stack.push(a*b); break; case "/": if(b == 0){ throw new ExpressionException(""); } stack.push(a/b); break; default: throw new ExpressionException(""); } } else { throw new ExpressionException(""); } } int result = stack.pop(); if(!stack.isEmpty()) { throw new ExpressionException(""); } return result; } /** * Returns true if s is an operator. * * A word of caution on using the String.matches method: it returns true * if and only if the whole given string matches the regex. Therefore * using the regex "[0-9]" is equivalent to "^[0-9]$". * * An operator is one of '+', '-', '*', '/'. */ private static boolean isOperator(String s) { return s.matches("[-+*/]"); } /** * Returns true if s is an integer. * * A word of caution on using the String.matches method: it returns true * if and only if the whole given string matches the regex. Therefore * using the regex "[0-9]" is equivalent to "^[0-9]$". * * We accept two types of integers: * * - the first type consists of an optional '-' * followed by a non-zero digit * followed by zero or more digits, * * - the second type consists of an optional '-' * followed by a single '0'. */ private static boolean isInteger(String s) { return s.matches("(((\\-)*?[1-9](\\d+)*)|(\\-)*?0)"); } }
The testclass is:
import org.junit.Test; import org.junit.Before; import org.junit.Rule; import org.junit.rules.Timeout; import static org.junit.Assert.*; import static org.hamcrest.MatcherAssert.assertThat; import static org.hamcrest.CoreMatchers.*; import java.util.Arrays; /** * Test class for Postfix * */ public class PostfixTest { @Rule public Timeout globalTimeout = Timeout.seconds(5); @Test public void evaluateIsCorrectWhenExpressionContainsManyOperandsInARow() throws Exception { String expression = "1 2 3 4 -0 + * - +"; int expected = -9; int actual = Postfix.evaluate(expression); assertThat(actual, equalTo(expected)); } @Test public void evaluateIsCorrectIntWhenExprContainsOnlyAPositiveInt() throws Exception { int evalZero = Postfix.evaluate("0"); int largeValue = 1234567890; int evalLarge = Postfix.evaluate(Integer.toString(largeValue)); assertThat(evalZero, equalTo(0)); assertThat(evalLarge, equalTo(largeValue)); } @Test public void evaluateIsCorrectIntWhenExprContainsOnlyANegativeInt() throws Exception { int evalMinusZero = Postfix.evaluate("-0"); int smallValue = -1234567890; int evalSmall = Postfix.evaluate(Integer.toString(smallValue)); assertThat(evalMinusZero, equalTo(-0)); assertThat(evalSmall, equalTo(smallValue)); } @Test public void evaluateIsCorrectWhenExprIsAdditionOfPositiveInts() throws Exception { int expected = 1 + 23; int actual = Postfix.evaluate("1 23 +"); assertThat(actual, equalTo(expected)); } @Test public void evaluateIsCorrectWhenExprIsAdditionOfMixedInts() throws Exception { int expected = 1 + 23 + -432; int actual = Postfix.evaluate("1 23 + -432 +"); assertThat(actual, equalTo(expected)); } @Test public void evaluateIsCorrectWhenExprWhitespaceIsTabs() throws Exception { int expected = 1 + 23; int actual = Postfix.evaluate("1 23 +"); assertThat(actual, equalTo(expected)); } @Test public void evaluateIsCorrectWhenExprWithespaceIsTabsAndSpaces() throws Exception { int expected = 1 + 23; int actual = Postfix.evaluate(" 1 23 +"); assertThat(actual, equalTo(expected)); } @Test public void evaluateIsCorrectWhenExprContainsLeadingAndTrailingWhitespace() throws Exception { int expected = 1 + 23; int actual = Postfix.evaluate(" 1 23 + "); assertThat(actual, equalTo(expected)); } @Test public void evaluateIsCorrectWhenExprIsSubtractionOfPositiveInts() throws Exception { int expected = 1 - 23; int actual = Postfix.evaluate("1 23 -"); assertThat(actual, equalTo(expected)); } @Test public void evaluateIsCorrectWhenExprIsSubtractionOfMixedInts() throws Exception { int expected = 1 - -23; int actual = Postfix.evaluate("1 -23 -"); assertThat(actual, equalTo(expected)); } @Test public void evaluateIsCorrectWhenExprIsMultiplication() throws Exception { int expected = 34 * 123; int actual = Postfix.evaluate("34 123 *"); assertThat(actual, equalTo(expected)); } @Test public void evaluateIsCorrectWhenExprIsDivision() throws Exception { int expected = 342 / 5; int actual = Postfix.evaluate("342 5 /"); assertThat(actual, equalTo(expected)); } @Test public void evaluateIsZeroWhenExprIsDivisionAndNumeratorIsZero() throws Exception { int expected = 0 / 1; int actual = Postfix.evaluate("0 1 /"); assertThat(actual, equalTo(expected)); } @Test public void evaluateIsCorrectWhenExprIsMixOfOperators() throws Exception { int expectedMinusMultPlus = (12 - 34) * (56 + -78); String minusMultPlusExpr = "12 34 - 56 -78 + *"; int expectedPlusDivMinus = (32 + 5) / 2 - 3; String plusDivMinusExpr = "32 5 + 2 / 3 -"; int expectedAllOperators = (((1 + 2) * 3) - 4) / 5; String allOperatorsExpr = "1 2 + 3 * 4 - 5 /"; int actualMinusMultPlus = Postfix.evaluate(minusMultPlusExpr); int actualPlusDivMinus = Postfix.evaluate(plusDivMinusExpr); int actualAllOperators = Postfix.evaluate(allOperatorsExpr); assertThat(actualMinusMultPlus, equalTo(expectedMinusMultPlus)); assertThat(actualPlusDivMinus, equalTo(expectedPlusDivMinus)); assertThat(actualAllOperators, equalTo(expectedAllOperators)); } @Test(expected = Postfix.ExpressionException.class) public void evaluateExceptionWhenExprIsEmptyString() throws Exception { Postfix.evaluate(""); } @Test public void evaluateExceptionWhenExprContainsOnlyAnOperator() { String[] expressions = {"+", "-", "/", "*"}; assertExpressionExceptionOnAll(expressions); } @Test public void evaluateExceptionWhenExprContainsIntsConnectedByDash() { String[] expressions = {"-1-0", "-0-1", "1-3"}; assertExpressionExceptionOnAll(expressions); } @Test public void evaluateExceptionWhenExprContainsTooFewOperands() { String[] expressions = {"1 +", " 1 2 + +"}; assertExpressionExceptionOnAll(expressions); } @Test(expected = Postfix.ExpressionException.class) public void evaluateExceptionWhenExprContainsTooManyOperands() throws Exception { Postfix.evaluate("1 2 3 +"); } @Test public void evaluateExceptionWhenExprContainsIncorrectlyPlacedOperators() { String[] expressions = {"1 2+", "1 2) 3 +*", "1 2+"}; assertExpressionExceptionOnAll(expressions); } @Test public void evaluateExceptionWhenExprContainsIllegalCharacters() { String[] expressions = {"1 2 ,", "1 2 .", "0x17", "x", "1234L"}; assertExpressionExceptionOnAll(expressions); } @Test public void evaluateExceptionWhenExprContainsPositiveIntWithLeadingZero() { String[] expressions = {"03", "017"}; assertExpressionExceptionOnAll(expressions); } @Test public void evaluateExceptionWhenExprContainsNegativeIntWithLeadingZero() { String[] expressions = {"-03", "-017"}; assertExpressionExceptionOnAll(expressions); } @Test(expected = Postfix.ExpressionException.class) public void evaluateExceptionOnDivideByZero() throws Exception { Postfix.evaluate("1 0 /"); } /** * Assert that every expression in expressions causes Postfix.evaluate to * throw an ExpressionException. * * @param expressions An array of expressions. */ private static void assertExpressionExceptionOnAll(String[] expressions) { for (String expr : expressions) { try { // Act Postfix.evaluate(expr); fail("Expected ExpressionException on input: " + expr); } catch (Postfix.ExpressionException e) { // Exception thrown, all good! } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
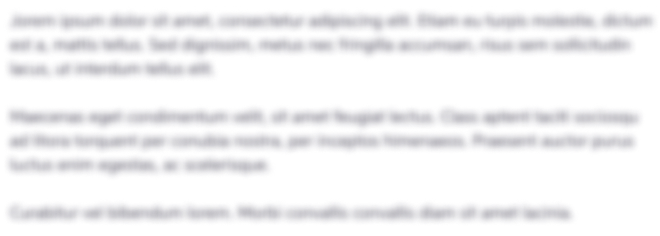
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started