Question
Fix the code so it pass the tests. (Header.h) #ifndef BINARY_TREE_H #define BINARY_TREE_H #include #include #include #include #include using namespace std; template struct Node {
Fix the code so it pass the tests.
(Header.h)
#ifndef BINARY_TREE_H #define BINARY_TREE_H #include
using namespace std;
template
template
protected: Node
private: void destroy(Node
int height(int level, Node
template
template
template
{
destroy(curr->left);
destroy(curr->right);
delete curr;
curr = nullptr; } }
template
insert(item, root);
}
template
Node
Node
Node
newNode = new Node
assert(newNode != nullptr);
newNode->item = item;
newNode->left = nullptr;
newNode->right = nullptr;
if (root == nullptr)
{
root = newNode;
} else
{
current = curr;
while (current != nullptr)
{
trailCurrent = current;
if (current->item == item)
{
cerr << "The insert item is already in the list-";
cerr << "duplicates are not allowed." << endl;
return; }
else if (current->item > item)
current = current->left;
else
current = current->right;
} // end while
if (trailCurrent->item > item)
trailCurrent->left = newNode;
else
trailCurrent->right = newNode; }
}
template
if (p != nullptr)
{
cout << p->item << " ";
preorder(p->left);
preorder(p->right); } }
template
template
{
inOrder(p->left);
cout << p->item << " ";
inOrder(p->right); } }
template
{
cout << p->item << " ";
postOrder(p->left);
postOrder(p->right); } }
template
template
// recursive call to left child and right child and // add the result of these with 1 ( 1 for counting the root) return 1 + countNode(root->left) + countNode(root->right); }
template
template
if (curr == nullptr) return 0; if (curr->left == nullptr && curr->right == nullptr) return 1; else return leavesCount(curr->left) + leavesCount(curr->right); }
template
template
bool found = false;
if (root == nullptr)
return nullptr;
else
{
current = root;
while (current != nullptr && !found)
{
if (current->item == searchItem) { return current; }
else if (current->item > searchItem)
current = current->left;
else
current = current->right;
} // end while
} // end else
return nullptr; }
template
if (root == nullptr) { return nullptr; } if (root->right == nullptr) { return root; } return findRightMostNode(root->right);
}
template
{
Node
Node
Node
if (p == nullptr)
cerr << "Error: The node to be deleted is nullptr." << endl;
else if (p->left == nullptr && p->right == nullptr)
{
temp = p;
p = nullptr;
delete temp; }
else if (p->left == nullptr)
{
temp = p;
p = temp->right;
delete temp; }
else if (p->right == nullptr)
{
temp = p;
p = temp->left;
delete temp; }
else
{
current = p->left;
trailCurrent = nullptr;
while (current->right != nullptr)
{
trailCurrent = current;
current = current->right;
} // end while
p->item = current->item;
if (trailCurrent == nullptr) // current did not move; current == p->left; adjust p
p->left = current->left;
else
trailCurrent->right = current->left;
delete current;
} // end else
} // end deleteFromTree
template
template
Node
bool found = false;
if (root == nullptr)
cout << "Cannot delete from the empty tree." << endl;
else
{
current = root;
trailCurrent = root;
while (current != nullptr && !found)
{
if (current->item == deleteItem)
found = true;
else
{
trailCurrent = current;
if (current->item > deleteItem)
current = current->left;
else
current = current->right; }
} // end while
if (current == nullptr)
cout << "The delete item is not in the tree." << endl;
else if (found)
{
if (current == root)
deleteFromTree(root);
else if (trailCurrent->item > deleteItem)
deleteFromTree(trailCurrent->left);
else
deleteFromTree(trailCurrent->right);
} // end if } return root; }
template
/* use the larger one */ if (lDepth > rDepth) return(lDepth + 1); else return(rDepth + 1); } }
#endif // BINARY_TREE_H
(Tester.cpp)
#include
using namespace std; bool checkTest(string testName, bool condition); bool checkTest(string testName, int whatItShouldBe, int whatItIs); void testFindMethod(); void testRightMostNodeSearch(); void deleteTest(); void testHeight(); void testLeavesCount();
BinaryTree
int main() { BinaryTree
BinaryTree
for(int i = 0; i < size; i++){ myTree.insert(values[i]); }
return myTree; }
void testFindMethod( ){ BinaryTree
void testRightMostNodeSearch(){ BinaryTree
checkTest("Test 3: RightNodeTest(49)", 67, ptrchild->item); }
void deleteTest(){ BinaryTree
myTree.remove(67); ptr = myTree.find(67); if(!checkTest("Test 5: Delete Node with Left Child: 67", ptr == nullptr)){ cout << "In Order: "; myTree.inOrder(); cout << endl; }
myTree.remove(42); ptr = myTree.find(42); if(!checkTest("Test 6: Delete Node with Right Child: 42", ptr == nullptr)){ cout << "In Order: "; myTree.inOrder(); cout << endl; }
myTree.remove(73); ptr = myTree.find(73); if(!checkTest("Test 7: Delete Node with two children: 73", ptr == nullptr)){ cout << "In Order: "; myTree.inOrder(); cout << endl; }
myTree.remove(37); ptr = myTree.find(37); if(!checkTest("Test 8: Delete root: 37", ptr == nullptr)){ cout << "In Order: "; myTree.inOrder(); cout << endl; } }
void testHeight(){ BinaryTree
void testLeavesCount(){ BinaryTree
//Helps with Testing bool checkTest(string testName, int whatItShouldBe, int whatItIs) {
if (whatItShouldBe == whatItIs) { cout << "Passed " << testName << endl; return true; } else { cout << "***Failed test " << testName << " *** " << endl << " Output was " << whatItIs << endl << " Output should have been " << whatItShouldBe << endl; return false; } } //Helps with Testing bool checkTest(string testName, bool condition) {
if (condition) { cout << "Passed " << testName << endl; return true; } else { cout << "***Failed test " << testName << " *** " << endl; return false; }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
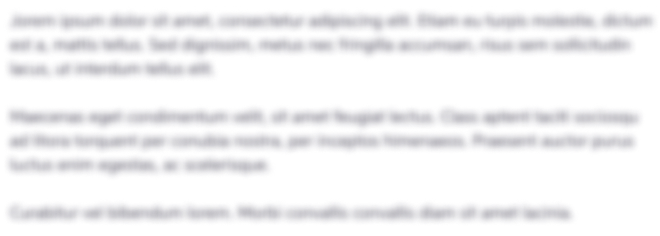
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started