Question
Fix the todo in Point.java Ball.java package hw3; import java.awt.Color; import java.awt.Point; import edu.princeton.cs.algs4.StdDraw; /** * A class that models a bounding ball */ public
Fix the todo in Point.java
Ball.java
package hw3;
import java.awt.Color; import java.awt.Point;
import edu.princeton.cs.algs4.StdDraw;
/** * A class that models a bounding ball */ public class Ball { // Minimum and maximum x and y values for the screen private static double minX; private static double minY; private static double maxX; private static double maxY;
private Point center; private double radius; // Every time the ball moves, its x position changes by vX; private double vX; // Every time the ball moves, its y position changes by vY; private double vY; private Color color;
/** * Sets the minimum and maximum x and y values of the screen on which the balls appear. * The bottom left of the screen is at (minX
, minY
) while the * top right is at (maxX
, maxY
). * @param minX the leftmost x value * @param minY the bottommost y value * @param maxX the rightmost x value * @param maxY the topmost y value */ public static void setScreen(double minX, double minY, double maxX, double maxY) { Ball.minX = minX; Ball.minY = minY; Ball.maxX = maxX; Ball.maxY = maxY; } /** * Constructs a ball. * @param centerX the x coordinate of the center of the ball * @param centerY the y coordinate of the center of the ball * @param r the radius of the ball * @param vX the velocity of the ball along the x-axis * @param vY the velocity of the ball along the y-axis * @param col the color of the ball */ public Ball(double centerX, double centerY, double r, double vX, double vY, Color col) { center = new Point(centerX, centerY); radius = r; this.vX = vX; this.vY = vY; color = col; } /** * Moves the ball (changes its position) by vX along the x-axis * and by vY along the y-axis. Additionally, if the ball has * reached one of the edges of the screen it changes the velocity * to reflect that the ball has bounced off the edge. */ public void move() { center.moveTo (center.getX()+this.vX, center.getY()+this.vY); if (center.getX()+this.radius >= this.maxX) this.vX -= 1 if (center.getX()-this.radius <= this.minX) this.vX += 1 if (center.getY()+this.radius >= this.maxY) this.vY -= 1 if (center.getY()-this.radius <= this.minY) this.vY += 1 } /** * Determines if this
has collided with b2
. * @param b2 the other Ball
* @return ture
if this
has collided with * b2
. */ public boolean collision(Ball b2) { //TODO - This code is wrong and needs to be fixed return false; } /** * Draws the Ball
on the screen. */ public void draw() { StdDraw.setPenColor(color); StdDraw.filledCircle(center.getX(), center.getY(), radius); } } BouncingBalls.java
package hw3;
import java.awt.Color; import edu.princeton.cs.algs4.StdDraw;
public class BouncingBalls { // Number of balls private static final int BALL_COUNT = 4; // Window runs from (-WINDOW_MAX,-WINDOW_MAX) in bottom left to // (WINDOW_MAX, WINDOW_MAX) in the top right private static final int WINDOW_MAX = 400; // The maximum ball radius private static final int MAX_BALL_RADIUS = 40; // The maximam velocity in both the x direction and the y direction. private static final double MAX_VELOCITY = 1.0;
public static void main(String[] args) throws Exception { // Initialize StdDraw StdDraw.enableDoubleBuffering(); int windowSize = 2 * WINDOW_MAX + 1; StdDraw.setCanvasSize(windowSize, windowSize); StdDraw.setXscale(-WINDOW_MAX, WINDOW_MAX); StdDraw.setYscale(-WINDOW_MAX, WINDOW_MAX); StdDraw.clear(Color.BLACK); // Let the Ball class know the min and max coordinates of the window Ball.setScreen(-WINDOW_MAX, -WINDOW_MAX, WINDOW_MAX, WINDOW_MAX); // Initialize Ball objects in animation Ball[] ball = new Ball[BALL_COUNT]; for(int i = 0; i < ball.length; i++) { ball[i] = createRandomBall(); } while(true) { // clear the screen StdDraw.clear(Color.BLACK); // move and draw the balls in window buffer for(Ball b : ball) { b.move(); b.draw(); } // draw window buffer on screen and pause StdDraw.show(); StdDraw.pause(5); // If there is a collision pause and then // start over with random balls. if (checkCollisions(ball)) { StdDraw.pause(2000); for(int i = 0; i < ball.length; i++) ball[i] = createRandomBall(); } } } /** * Checks if any of the Ball
s in b
* have collided. * @param b an array of Ball
objects to check * @return true
if any of the Ball
* objects in b
have collided and * false
otherwise. */ private static boolean checkCollisions(Ball[] b) { // TODO - this code is wrong and needs to be fixed. return false; } /** * Creates a new Ball
that is randomly placed on the screen * and given a random size (radius), a random velocity, and a random color. * @return a new randomly generated Ball
object. */ private static Ball createRandomBall() { double minPos = -WINDOW_MAX + MAX_BALL_RADIUS; double maxPos = WINDOW_MAX - MAX_BALL_RADIUS; double x = HW3Utils.randomDouble(minPos, maxPos); double y = HW3Utils.randomDouble(minPos, maxPos); double r = HW3Utils.randomDouble(0.5 * MAX_BALL_RADIUS, MAX_BALL_RADIUS); double vX = HW3Utils.randomDouble(-MAX_VELOCITY, MAX_VELOCITY); double vY = HW3Utils.randomDouble(-MAX_VELOCITY, MAX_VELOCITY); Color c = HW3Utils.randomColor(); Ball b = new Ball(x, y, r, vX, vY, c); return b; } }
Point.java
package hw3;
/** * A class to that models a 2D point. */ public class Point { private double x; private double y;
/** * Construct the point (x
, y
). * @param x the Point
's x coordinate * @param y the Point
's y coordinate */ public Point(double x, double y) { this.x = x; this.y = y; } /** * Move the point to (newX
, newY
). * @param newX the new x coordinate for this Point
. * @param newY the new y coordinate for this Point
. */ public void moveTo(double newX, double newY) { x = newX; y = newY; }
/** * Returns the point's x coordinate * @return the point's x coordinate */ public double getX() { return x; }
/** * Returns the point's y coordinate * @return the point's y coordinate */ public double getY() { return y; } /** * Returns the distance between this Point
and the Point p2
* @param p2 the other Point
* @return the distance between this Point
and the Point p2
*/ public double distance(Point p2) { double distance = Math.sqrt(Math.pow(this.x-p2.x,2) + Math.pow(this.y-p2.y,2)); return distance; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
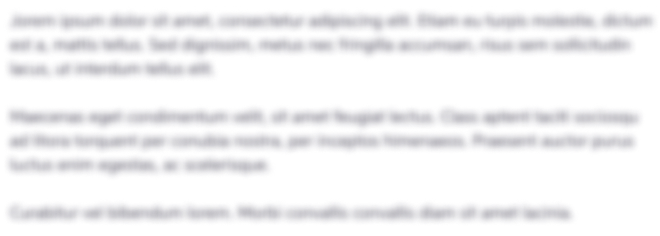
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started