Question
Fix this code so it passes the JUNIT Test for hasConsecutive. IN JAVA!!! public class PA2b { /** * Error to supply if input is
Fix this code so it passes the JUNIT Test for hasConsecutive. IN JAVA!!!
public class PA2b {
/**
* Error to supply if input is not positive
*/
public static final String ERR_VALUES = "Number of values must be positive.";
/**
* Returns true if the supplied array has a sequence of k consecutive values
*/
public static boolean hasConsecutive(int[] values, int k) {
// if (values.length == 1 && k == 1) {
// return true;
// }
// int count = 1;
//
// for (int i = 0; i < values.length; i++) {
// if (i > 0 && values[i] == values[i - 1]) {
// count++;
// if (count >= k - 1) {
// return true;
// }
// } else {
// count = 1;
// }
// }
// return false;
//
// }
for (int i = 0; i < values.length - k + 1; i++) {
boolean isConsecutive = true;
for (int j = i; j < i + k - 1; j++) {
if (values[j + 1] - values[j] != 1 || values[j] < 0) {
isConsecutive = false;
break;
}
}
if (isConsecutive) {
return true;
}
}
return false;
}
/**
* Returns the length of the longest consecutive sequence in the supplied array
*/
public static int maxConsecutive(int[] values) {
if (values.length == 0) {
return 0;
} else if (values.length == 1) {
return 1;
}
int max_count = 1;
int curr_count = 1;
int previous = values[0];
for (int i = 1; i < values.length; i++) {
if (values[i] == previous) {
curr_count++;
max_count = Math.max(curr_count, max_count);
} else {
curr_count = 1;
}
previous = values[i];
}
return max_count;
}
/**
* Inputs an array of numbers and outputs the longest consecutive sequence of
* values
*/
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
/*
* Asks the user for the number of values
*/
System.out.print("Enter the number of values: ");
int n = scanner.nextInt();
/*
* Checks to see if the users input is a positive. If the input is not, then the
* user will get the error code ERR_VALUES. Then exits the program.
*/
if (n <= 0) {
System.out.println(ERR_VALUES);
System.exit(0);
}
int arr[] = new int[n];
/*
* Asks the user to enter the values of the array.
*/
System.out.print("Enter the values: ");
for (int i = 0; i < n; i++) {
arr[i] = scanner.nextInt();
}
scanner.close();
/*
* After doing the calculations, if it passed the ERR_VALUES, then the user will
* get the output back. Which is the largest consecutive number.
*/
System.out.printf("The maximum length of consecutive values is %s.%n", maxConsecutive(arr));
}
}
Here is the code for the JUNIT TEST.
public void testHasConsecutive() { _testHasConsecutive(new int[] {}, -1, false); _testHasConsecutive(new int[] {}, 0, false); _testHasConsecutive(new int[] {}, 1, false); _testHasConsecutive(new int[] {}, 2, false); _testHasConsecutive(new int[] {}, 3, false); _testHasConsecutive(new int[] {}, 4, false); _testHasConsecutive(new int[] {1}, -1, false); _testHasConsecutive(new int[] {1}, 0, false); _testHasConsecutive(new int[] {1}, 1, true); _testHasConsecutive(new int[] {1}, 2, false); _testHasConsecutive(new int[] {1}, 3, false); _testHasConsecutive(new int[] {1}, 3, false); _testHasConsecutive(new int[] {1, 2}, -1, false); _testHasConsecutive(new int[] {1, 2}, 0, false); _testHasConsecutive(new int[] {1, 2}, 1, true); _testHasConsecutive(new int[] {1, 2}, 2, false); _testHasConsecutive(new int[] {1, 2}, 3, false); _testHasConsecutive(new int[] {1, 2}, 4, false); _testHasConsecutive(new int[] {2, 2}, -1, false); _testHasConsecutive(new int[] {2, 2}, 0, false); _testHasConsecutive(new int[] {2, 2}, 1, true); _testHasConsecutive(new int[] {2, 2}, 2, true); _testHasConsecutive(new int[] {2, 2}, 3, false); _testHasConsecutive(new int[] {2, 2}, 4, false); _testHasConsecutive(new int[] {1, 2, 1}, -1, false); _testHasConsecutive(new int[] {1, 2, 1}, 0, false); _testHasConsecutive(new int[] {1, 2, 1}, 1, true); _testHasConsecutive(new int[] {1, 2, 1}, 2, false); _testHasConsecutive(new int[] {1, 2, 1}, 3, false); _testHasConsecutive(new int[] {1, 2, 1}, 3, false); _testHasConsecutive(new int[] {3, 3, 5, 5, 5, 5, 4}, -1, false); _testHasConsecutive(new int[] {3, 3, 5, 5, 5, 5, 4}, 0, false); _testHasConsecutive(new int[] {3, 3, 5, 5, 5, 5, 4}, 1, true); _testHasConsecutive(new int[] {3, 3, 5, 5, 5, 5, 4}, 2, true); _testHasConsecutive(new int[] {3, 3, 5, 5, 5, 5, 4}, 3, true); _testHasConsecutive(new int[] {3, 3, 5, 5, 5, 5, 4}, 4, true); _testHasConsecutive(new int[] {3, 3, 5, 5, 5, 5, 4}, 5, false); _testHasConsecutive(new int[] {3, 3, 5, 5, 5, 5, 4}, 6, false); _testHasConsecutive(new int[] {3, 3, 5, 5, 5, 5, 4}, 7, false); _testHasConsecutive(new int[] {3, 3, 5, 5, 5, 5, 4}, 8, false); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
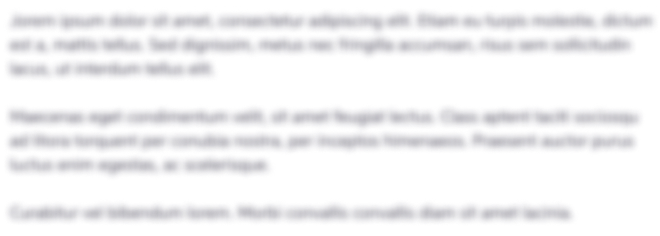
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started