Question
Following is a Code (in C#) for a Customer-Supplier Relationship Management System. There are Customers, Suppliers, Products, Categories, Quantities and. Prices *** Modify the Code
Following is a Code (in C#) for a Customer-Supplier Relationship Management System.
There are Customers, Suppliers, Products, Categories, Quantities and. Prices
***Modify the Code below so that Data Entered in this system can be Saved and Read from a Text File and If Microsoft Visual Studio is used, where would this text file would be located***
Customer's: ===========
Back End: ---------------
public class Customers { public int customer_id, pincode; public string address, name;
public string read(){}
public int Customer_id { get{ return customer_id; } set{ customer_id = value; } }
public int Pincode { get{ return pincode; } set{ pincode = value; } }
public string Address { get{ return address; } set{ address = value; } }
public string Name { get{ return name; } set{ name = value; } }
}
Front End: ---------------
//Drag and drop 4 labels and 4 textboxes and a button from the toolbar for better nomenclature.
//Inside the Form_load insert the following code:
Label1.Text = "Customer_id";
Label2.Text = "Pincode";
Label3.Text = "Customer Name";
Label4.Text = "Address";
Textbox1.ReadOnly = False;
Textbox2.ReadOnly = False;
Textbox3.ReadOnly = False;
Textbox4.ReadOnly = False;
Textbox4.MultiLine = True; // Because address can be long and it requires more than a single line
Textbox1.Width = 200;
Textbox1.Height = 50;
Textbox2.Width = 200;
Textbox2.Height = 50;
Textbox3.Width = 200;
Textbox3.Height = 50;
Textbox4.Width = 200;
Textbox4.Height = 50;
Button1.Text = "Submit"
Supplier: =========
Back End: ---------
public class Suppliers { public int supplier_id, phone; public string name; public Customers *c; public Products *p;
public Suppliers(Customers *c, Products *P) { this.c = c; this.p = p; }
public int Supplier_id { get{ return supplier_id; } set{ supplier_id = value; } }
public int phone { get{ return phone; } set{ phone = value; } }
public string Name { get{ return name; } set{ name = value; } }
public string create_inv(){} public string read(){} }
Front End: ---------------
//Drag and drop 3 labels, 3 textboxes and a button for a better gui.
//Inside the form_load of the products project put the following code:
Label1.Text = "Suppliers_id";
Label2.Text = "Phone no.";
Label1.Text= "Supplier's Name";
Textbox1.ReadOnly = False;
Textbox2.ReadOnly = False;
Textbox3.ReadOnly = False;
Textbox1.Width = 200;
Textbox1.Height = 50;
Textbox2.Width = 200;
Textbox2.Height = 50;
Textbox3.Width = 200;
Textbox3.Height = 50;
Button1.Text = "Submit" // In-order to send the supplier's data into the database
Product: ========
Back End: ---------
public class Products { public int product_id; public string product_name; public double discount;
public int Product_id { get{ return product_id; } set{ product_id = value; } }
public string Product_name { get{ return product_name; } set{ product_name = value; } }
public double Discount { get{ return discount; } set{ discount = value; } }
public void create(){} public void delete(){} public void update(){} }
Front End: ---------------
//Drag and drop 3 labels, 3 textbox and a button in order to make a better gui
//Inside the form_load part of the products class enter the following code:
Label1.Text = "Product id";
Label2.Text = "Product name";
Label3.Text = "Discount";
Textbox1.ReadOnly = False;
Textbox2.ReadOnly = False;
Textbox3.ReadOnly = False;
Textbox1.Width = 200;
Textbox1.Height = 50;
Textbox2.Width = 200;
Textbox2.Height = 50;
Textbox3.Width = 200;
Textbox3.Height = 50;
Button1.Text = "Submit" // In-order to send the supplier's data into the database
Category ========
Back End: --------
public class Categories : Products { public int category_id; public string product_category;
public int Category_id { get{ return category_id; } set{ category_id = value; } }
public string Product_category { get{ return product_category; } set{ product_category = value; } }
public void create(){} public void delete(){} public void update(){} }
Front End: ----------
//Drag and drop 2 labels, 1 textbox , 1 combobox and 1 button control from the toolbar for better gui
//Inside the form_load section of the category form enter the following code:
Label1.Text = "Category id";
Label2.Text = "Select Category";
Textbox1.Width = 200;
Textbox1.Height = 50;
Button1.Text = "Submit"
Price: ======
Back End: ---------
public class Price : Products { public int price_id; public string product_price;
public int Price_id { get{ return price_id; } set{ price_id = value; } }
public string Product_price { get{ return product_price; } set{ product_price = value; } }
public void create(){} public void delete(){} public void update(){} }
Front End: ----------
//Drag and drop 2 labels, 2 textbox and 1 button control from the toolbar in order to generate a better gui
//Inside the form_load section of the PRICE form enter the following code:
Label1.Text = "Price id"
Label2.Text = "Price";
Textbox1.ReadOnly = False;
Textbox2.ReadOnly = False;
Textbox1.Width = 200;
Textbox1.Height = 50;
Textbox2.Width = 200;
Textbox2.Height = 50;
Button1.Text = "Submit"
Quantity: =========
Back End: --------
public class Quantity : Products { public int quantity_id, product_quantity;
public int Quantity_id { get{ return quantity_id; } set{ quantity_id = value; } }
public int Product_quantity { get{ return product_quantity; } set{ product_quantity = value; } }
public void create(){} public void delete(){} public void update(){} }
Front End: ---------- //Drag and drop 2 labels, 2 textbox and 1 button control from the toolbar in order to generate a better gui
//Inside the form_load section of the QUANTITY form enter the following code:
Label1.Text = "Quantity id"
Label2.Text = "Quantity";
Textbox1.ReadOnly = False;
Textbox2.ReadOnly = False;
Textbox1.Width = 200;
Textbox1.Height = 50;
Textbox2.Width = 200;
Textbox2.Height = 50;
Button1.Text = "Submit"
Step by Step Solution
There are 3 Steps involved in it
Step: 1
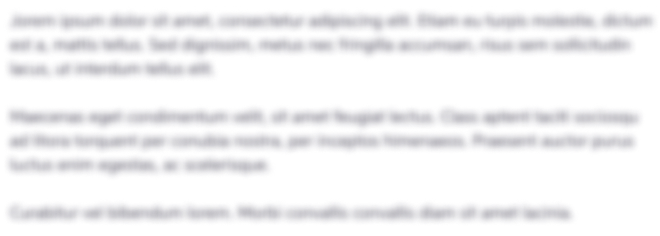
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started