Answered step by step
Verified Expert Solution
Question
1 Approved Answer
FOR JAVA: Summary: Revising Registrar program to ensure input data is logical. Solution should be names Rev_Registrar.java Currently, the program accepts month, day and year
FOR JAVA:
Summary: Revising Registrar program to ensure input data is logical.
- Solution should be names Rev_Registrar.java
- Currently, the program accepts month, day and year of enrollment.
- Modify program to do a range check on the month (1-12), and a range check on the day, based on the month. (You have the option to accommodate for leap years)
- Modify program to automatically populate year variable with current year.
- Document all changes.
- Run your program until it works and the output looks nice
The output/input can be whatever you'd like just as long as it follows the guidelines above. We are only testing to see if the program works as given by the instructions. There is no one right output answer.
Here is the original Registrar program for this assignment:
=========================================================================== import java.text.SimpleDateFormat; import java.util.GregorianCalendar; public class CollegeStudent { private String firstName; private String lastName; private GregorianCalendar enrollmentDate; private GregorianCalendar graduationDate; public CollegeStudent(String firstName, String lastName, int month, int day, int year) { this.firstName = firstName; this.lastName = lastName; this.enrollmentDate = new GregorianCalendar(year, month-1, day); this.graduationDate = new GregorianCalendar(year + 4, month-1, day); } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public GregorianCalendar getEnrollmentDate() { return enrollmentDate; } public void setEnrollmentDate(int month, int day, int year) { this.enrollmentDate = new GregorianCalendar(year, month-1, day); } public GregorianCalendar getGraduationDate() { return graduationDate; } public void setGraduationDate(int month, int day, int year) { this.graduationDate = new GregorianCalendar(year, month-1, day); } @Override public String toString() { SimpleDateFormat format = new SimpleDateFormat("dd-MMM-yyyy"); return "Student Name: " + getFirstName() + ", " + getLastName() + ", Enrollment Date: " + format.format(enrollmentDate.getTime()) + ", Graduation Date: " + format.format(graduationDate.getTime()); } }
==========================================================
import java.util.Scanner; public class Registrar { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Student 1"); System.out.print("Enter first name: "); String fName = scanner.nextLine(); System.out.print("Enter last name: "); String lName = scanner.nextLine(); System.out.print("Enter enrollment day: "); int day = scanner.nextInt(); System.out.print("Enter enrollment month: "); int month = scanner.nextInt(); System.out.print("Enter enrollment year: "); int year = scanner.nextInt(); scanner.nextLine(); CollegeStudent studentOne = new CollegeStudent(fName, lName, month, day, year); System.out.println("Student 2"); System.out.print("Enter first name: "); fName = scanner.nextLine(); System.out.print("Enter last name: "); lName = scanner.nextLine(); System.out.print("Enter enrollment day: "); day = scanner.nextInt(); System.out.print("Enter enrollment month: "); month = scanner.nextInt(); System.out.print("Enter enrollment year: "); year = scanner.nextInt(); CollegeStudent studentTwo = new CollegeStudent(fName, lName, month, day, year); System.out.println(studentOne); System.out.println(studentTwo); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
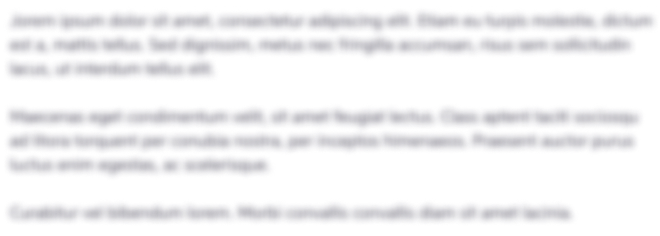
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started