Question
for my School Manager System assignment I stuck at Number 4 of this below table which is registration. I put my all codes plus my
for my School Manager System assignment I stuck at Number 4 of this below table which is registration. I put my all codes plus my code for this table please help me to pass this
Services (Implementation)
You might have some helper methods of your own in the services if necessary.
make a package in the src/main/java folder named jpa.service, create two classes as StudentService and CourseService which implements the respective DAOs. These classes are going to be used to interact with the respective tables in your database instance.
No. | Return Type | Class Name | Method Name | Input Parameters |
1 | List | StudentService | getAllStudents -This method reads the student table in your database and returns the data as a List | None |
2 | Student | StudentService | getStudentByEmail -This method takes a Student's email as a String and parses the student list for a Student with that email and returns a Student Object. | String sEmail |
3 | boolean | StudentService | validateStudent -This method takes two parameters: the first one is the user email and the second one is the password from the user input. Return whether or not a student was found. | String sEmail, String sPassword |
4 | void | StudentService | registerStudentToCourse -After a successful student validation, this method takes a Student's email and a Course ID. It checks in the join table (i.e. Student_Course) generated by JPA to find if a Student with that Email is currently attending a Course with that ID. If the Student is not attending that Course, register the student for that course; otherwise not. | String sEmail, int cId |
5 | List | StudentService | getStudentCourses -This method takes a Student's Email as a parameter and would find all the courses a student is registered for. | String sEmail |
6 | List | CourseService | getAllCourses -This method takes no parameter and returns every Course in the table. |
Models:
package jpa.entitymodels;import java.io.Serializable;import java.util.List;import javax.persistence.CascadeType;import javax.persistence.Column;import javax.persistence.Entity;import javax.persistence.GeneratedValue;import javax.persistence.GenerationType;import javax.persistence.Id;import javax.persistence.JoinColumn;import javax.persistence.ManyToMany;import javax.persistence.ManyToOne;import javax.persistence.NamedQueries;import javax.persistence.NamedQuery;import javax.persistence.OneToOne;import javax.persistence.Table;@Entity@Table@NamedQueries({ @NamedQuery( name="Select_All_Courses", query="from Course"),})public class Course implements Serializable { private static final long serialVersionUID = 1L; //Select_All_Courses //class variables @Id @Column(name = "id", nullable = false) private int cId; @Column(name = "name", nullable = false, length = 50) private String cName; @Column(name = "instructor", nullable = false, length = 50) private String cInstructorName; public Course(int cId, String cName, String cInstructorName) { this.cId = cId; this.cName = cName; this.cInstructorName = cInstructorName; } //default constructor public Course() {} //generate getters setters public int getcId() { return cId; } public void setcId(int cId) { this.cId = cId; } public String getcName() { return cName; } public void setcName(String cName) { this.cName = cName; } public String getcInstructorName() { return cInstructorName; } public void setcInstructorName(String cInstructorName) { this.cInstructorName = cInstructorName; }}
package jpa.entitymodels;import java.io.Serializable;import java.util.ArrayList;import java.util.List;import javax.persistence.CascadeType;import javax.persistence.Column;import javax.persistence.Entity;import javax.persistence.FetchType;import javax.persistence.GeneratedValue;import javax.persistence.GenerationType;import javax.persistence.Id;import javax.persistence.JoinColumn;import javax.persistence.ManyToMany;import javax.persistence.ManyToOne;import javax.persistence.NamedQueries;import javax.persistence.NamedQuery;import javax.persistence.OneToOne;import javax.persistence.Table;@Entity@Table(name = "student")@NamedQueries({ @NamedQuery( name="Select_All_Students", query="from Student"), @NamedQuery( name="Select_Student_By_Email", query="Select c from Student c where c.sEmail = :email")})public class Student implements Serializable{ private static final long serialVersionUID = 1L; //class variables @Id @Column(name = "email", nullable = false, length = 50) private String sEmail; @Column(name = "name", nullable = false, length = 50) private String sName; @Column(name = "password", nullable = false, length = 50) private String sPass; //relationship @ManyToMany(targetEntity=Course.class, cascade = {CascadeType.ALL}, fetch = FetchType.EAGER) private List sCourses = new ArrayList(); public Student(String sEmail, String sName, String sPass, List sCourses) { this.sEmail = sEmail; this.sName = sName; this.sPass = sPass; this.sCourses = sCourses; } //default constructor public Student(){} //getters setters public String getsEmail() { return sEmail; } public void setsEmail(String sEmail) { this.sEmail = sEmail; } public String getsName() { return sName; } public void setsName(String sName) { this.sName = sName; } public String getsPass() { return sPass; } public void setsPass(String sPass) { this.sPass = sPass; } public List getsCourses() { return sCourses; } public void setsCourses(List sCourses) { this.sCourses = sCourses; } public static long getSerialversionuid() { return serialVersionUID; }}
package jpa.entitymodels;import java.io.Serializable;import javax.persistence.Column;import javax.persistence.Entity;import javax.persistence.Id;import javax.persistence.NamedQueries;import javax.persistence.NamedQuery;import javax.persistence.Table;@Entity@Table(name ="student_course")@NamedQueries({ @NamedQuery( name="Select_All_Registered_Courses", query="from RegisteredCourse"), @NamedQuery( name="Select_Registered_Courses_by_Student", query="Select c from RegisteredCourse c where c.Student_Student_Email = :email"),})public class RegisteredCourse implements Serializable { private static final long serialVersionUID = 1L; //class variables @Id @Column(name = "Student_Student_Email", nullable = false) private String Student_Student_Email; @Id @Column(name = "sCourses_Course_Id", nullable = false) private int sCourses_Course_Id; public RegisteredCourse(String student_Student_Email, Integer sCourses_Course_Id) { this.Student_Student_Email = student_Student_Email; this.sCourses_Course_Id = sCourses_Course_Id; } //default constructor public RegisteredCourse() {} //Getters Setters public String getStudent_Student_Email() { return Student_Student_Email; } public void setStudent_Student_Email(String student_Student_Email) { Student_Student_Email = student_Student_Email; } public Integer getsCourses_Course_Id() { return sCourses_Course_Id; } public void setsCourses_Course_Id(Integer sCourses_Course_Id) { this.sCourses_Course_Id = sCourses_Course_Id; } public static long getSerialversionuid() { return serialVersionUID; } @Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + sCourses_Course_Id; result = prime * result + ((Student_Student_Email == null) ? 0 : Student_Student_Email.hashCode()); return result; } /* * (non-Javadoc) * * @see java.lang.Object#equals(java.lang.Object) */ @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (getClass() != obj.getClass()) return false; RegisteredCourse other = (RegisteredCourse) obj; if (sCourses_Course_Id != other.sCourses_Course_Id) return false; if (Student_Student_Email == null) { if (other.Student_Student_Email != null) return false; } else if (!Student_Student_Email.equals(other.Student_Student_Email)) return false; return true; } }
package jpa.dao;import java.util.List;import jpa.entitymodels.Course;public interface CourseDao { List getAllCourses(); Course GetCourseById(int courseId);}
package jpa.dao;import java.util.List;import jpa.entitymodels.Course;public interface StudentCourseServiceDao { List getAllStudentCourses(String studentEmail);}
package jpa.dao;import java.util.List;import jpa.entitymodels.Course;import jpa.entitymodels.Student;public interface StudentDao { List getAllStudents(); Student getStudentByEmail(String studentEmail); boolean validateStudent(String studentEmail, String studentPassword); void registerStudentToCourse(String studentEmail, int courseId); List getStudentCourses(String sEmail);}
jpa.service
package jpa.service;import java.util.List;import javax.persistence.TypedQuery;import org.hibernate.Session;import org.hibernate.SessionFactory;import org.hibernate.cfg.Configuration;import jpa.dao.CourseDao;import jpa.entitymodels.Course;import jpa.entitymodels.Student;public class CourseService implements CourseDao { //This method takes no parameter and returns every Course in the table. public List getAllCourses() { // TODO Auto-generated method stub SessionFactory factory = new Configuration().configure().buildSessionFactory(); Session session = factory.openSession(); TypedQuery query = session.getNamedQuery("Select_All_Courses"); List courses = query.getResultList(); //testing for(Course o: courses){ System.out.println("Course Id: " + o.getcId() +" | Course instructor : "+ o.getcInstructorName()+" | course name: "+ o.getcName()); } factory.close(); session.close(); return courses; } //gets a course by Id public Course GetCourseById(int courseId) { // TODO Auto-generated method stub SessionFactory factory = new Configuration().configure().buildSessionFactory(); Session session = factory.openSession(); Course course = (Course) session.get(Course.class,new Integer(courseId)); //testing System.out.println("***courseName***"); System.out.println(course.getcName()); //get all courses //find the one specific course and return it return course; }}
package jpa.service;import java.util.ArrayList;import java.util.List;import javax.persistence.TypedQuery;import org.hibernate.Session;import org.hibernate.SessionFactory;import org.hibernate.cfg.Configuration;import jpa.dao.StudentCourseServiceDao;import jpa.entitymodels.Course;import jpa.entitymodels.RegisteredCourse;import jpa.entitymodels.Student;public class StudentCourseService implements StudentCourseServiceDao { //return all student courses public List getAllStudentCourses(String studentEmail) { List result = new ArrayList(); // TODO Auto-generated method stub SessionFactory factory = new Configuration().configure().buildSessionFactory(); Session session = factory.openSession(); TypedQuery query = session.createNamedQuery("curseTaken",RegisteredCourse.class); query.setParameter("email", studentEmail); List enrolled = query.getResultList(); CourseService cs = new CourseService(); for (RegisteredCourse sc : enrolled) { result.add(cs.GetCourseById(sc.getsCourses_Course_Id())); } factory.close(); session.close(); //return all courses of a particular student return result; }}
package jpa.service;import java.util.Iterator;import java.util.List;import javax.persistence.TypedQuery;import org.hibernate.Session;import org.hibernate.SessionFactory;import org.hibernate.Transaction;import org.hibernate.cfg.Configuration;import jpa.dao.StudentDao;import jpa.entitymodels.Course;import jpa.entitymodels.Student;public class StudentService implements StudentDao { //This method reads the student table in your database and returns the data as a List public List getAllStudents() { // TODO Auto-generated method stub SessionFactory factory = new Configuration().configure().buildSessionFactory(); Session session = factory.openSession(); TypedQuery query = session.getNamedQuery("Select_All_Courses"); List students = query.getResultList(); //testing Iterator itr = students.iterator(); for (Student u : students) { System.out.println("Email: " +u.getsEmail() + "|" + " Full name:" + u.getsName() +"|"+ "password: " + u.getsPass() +"|"); } factory.close(); session.close(); return students; } //This method takes a Student's email as a String and parses the student list for a Student with that email and returns a Student Object. public Student getStudentByEmail(String studentEmail) { // TODO Auto-generated method stub SessionFactory factory = new Configuration().configure().buildSessionFactory(); Session session = factory.openSession(); Student student = session.find(Student.class, studentEmail); // Student student = (Student) session.get(Student.class,new String(studentEmail));// // //testing// // System.out.println("email : "+student.getsEmail());// System.out.println("Name : "+student.getsName());// System.out.println("pass : "+student.getsPass()); //Close resources factory.close(); session.close(); return student; } //---------------------------------Validate Credentials-------------------- //This method takes two parameters: the first one is the user email and the second one is the password from the user input. Return whether or not a student was found. public boolean validateStudent(String studentEmail, String studentPassword) { // TODO Auto-generated method stub //Only students with the right credentials can log in. Otherwise, a message is displayed stating: "Wrong Credentials". //Valid students are able to see the courses they are registered for. //Valid students are able to register for any course in the system as long as they are not already registered. SessionFactory factory = new Configuration().configure().buildSessionFactory(); Session session = factory.openSession(); //Create Employee object using session.get() Student student = (Student) session.get(Student.class,new String(studentEmail)); String validPassword = student.getsPass(); if(studentPassword.equalsIgnoreCase(validPassword)) { /eed to add try catch blocks to close resources factory.close(); session.close(); return true; }else { System.out.println("Wrong Credentials"); factory.close(); session.close(); } //how are you going to validate a student? //i am going to be provided a student password //i need to grab from the database my student //i need to save the password attribute to a local variable //i need to run a comparison between the input and the local variable, //return true if correct //return an error message if false return false; } //---------------------------------registering a course to a student-------------------- //After a successful student validation, this method takes a Student's email and a Course ID. //It checks in the join table (i.e. Student_Course) generated by JPA to find if a Student with that Email is currently attending a Course with that ID. //If the Student is not attending that Course, register the student for that course; otherwise not. public void registerStudentToCourse(String studentEmail, int courseId) { // TODO Auto-generated method stub boolean temp = false; //testing System.out.println(studentEmail); System.out.println(courseId); //open session factory SessionFactory factory = new Configuration().configure().buildSessionFactory(); Session session2 = factory.openSession(); Transaction t = session2.beginTransaction(); //Create student object using session.get() Student student = (Student) session2.get(Student.class,new String(studentEmail)); //testing System.out.println("*** Student Details ***"); System.out.println("email : "+student.getsEmail()); System.out.println("Name : "+student.getsName()); System.out.println("pass : "+student.getsPass()); //create course list List coursesBeingTaken = student.getsCourses(); //test// Iterator q = coursesBeingTaken.iterator();// for (Course u : coursesBeingTaken) {// System.out.println("course being taken:" + u.getcId());// System.out.println("total courses:" + coursesBeingTaken.size());// int id = u.getcId();// } if(coursesBeingTaken.size() >= 1) { //compare each course in the course list System.out.println("stop here0"); Iterator itr = coursesBeingTaken.iterator(); for (Course u : coursesBeingTaken) { int id = u.getcId(); if(id == courseId) { System.out.println("course already being taken"); temp = true; } } if(temp == false) { //create the course object Course course = (Course) session2.get(Course.class,new Integer(courseId)); //insert the course object coursesBeingTaken.add(course); //set attribute student.setsCourses(coursesBeingTaken); session2.merge(student); session2.getTransaction().commit(); } } temp = false; t.commit(); factory.close(); session2.close(); } //----------------------------------------gets the courses the student is registered to------------------------------------------------- //This method takes a Student's Email as a parameter and would find all the courses a student is registered for. //should this pull the students course attribute or reference studentCourse table public List getStudentCourses(String studentEmail) { // TODO Auto-generated method stub SessionFactory factory = new Configuration().configure().buildSessionFactory(); Session session = factory.openSession(); //Create Employee object using session.get() Student student = (Student) session.get(Student.class,new String(studentEmail)); List studentClassList = student.getsCourses(); //how are you going to get student courses of a student? //being provided an email //generate that object from database // return its course attribute factory.close(); session.close(); return studentClassList; }}
Main
/* * Filename: SMSRunner.java* Author: Stefanski* 02/25/2020 */package jpa.mainrunner;import static java.lang.System.out;import java.util.List;import java.util.Scanner;import jpa.entitymodels.Course;import jpa.entitymodels.Student;import jpa.entitymodels.RegisteredCourse;import jpa.service.CourseService;import jpa.service.StudentCourseService;import jpa.service.StudentService;/**1 * * @author Harry * */public class SMSRunner { private Scanner sin; private StringBuilder sb; private CourseService courseService; private StudentService studentService; private Student currentStudent; public SMSRunner() { sin = new Scanner(System.in); sb = new StringBuilder(); courseService = new CourseService(); studentService = new StudentService(); } /** * @param args */ public static void main(String[] args) { SMSRunner sms = new SMSRunner(); sms.run(); } private void run() { // Login or quit switch (menu1()) { case 1: if (studentLogin()) { registerMenu(); } break; case 2: out.println("Goodbye!"); break; default: } } private int menu1() { System.out.println("Are you a(n)"); sb.append(" 1.Student 2. Quit Please, enter 1 or 2."); out.print(sb.toString()); sb.delete(0, sb.length()); return sin.nextInt(); } private boolean studentLogin() { boolean retValue = false; out.print("Enter your email address: "); String email = sin.next(); out.print("Enter your password: "); String password = sin.next(); Student student = studentService.getStudentByEmail(email); if (student != null) { currentStudent = student; } //here we reference the relationship table and that session is closed if (currentStudent != null && currentStudent.getsPass().equals(password)) { List courses = studentService.getStudentCourses(email); out.println("MyClasses"); if(courses.isEmpty()) { System.out.println("no registered courses"); retValue = false; } for (Course course : courses) { out.println("Course Id: " + course.getcId() +" | Course instructor : "+ course.getcInstructorName()+" | course name: "+ course.getcName()); } retValue = true; } else { out.println("User Validation failed. GoodBye!"); } return retValue; } private void registerMenu() { sb.append(" 1.Register a class 2. Logout Please Enter Selection: "); out.print(sb.toString()); sb.delete(0, sb.length()); switch (sin.nextInt()) { case 1: List allCourses = courseService.getAllCourses(); List studentCourses = studentService.getStudentCourses(currentStudent.getsEmail()); allCourses.removeAll(studentCourses); //out.printf("%5s%15S%15s ", "ID", "Course", "Instructor"); for (Course course : allCourses) { //out.println(course); out.println("Course Id: " + course.getcId() +" | Course instructor : "+ course.getcInstructorName()+" | course name: "+ course.getcName()); } out.println(); out.print("Enter Course Number: "); int number = sin.nextInt(); Course newCourse = courseService.GetCourseById(number); if (newCourse != null) { System.out.println("this prints0"); //test studentService.registerStudentToCourse(currentStudent.getsEmail(), newCourse.getcId()); Student temp = studentService.getStudentByEmail(currentStudent.getsEmail()); StudentCourseService scService = new StudentCourseService(); List sCourses = scService.getAllStudentCourses(temp.getsEmail()); out.println("MyClasses"); for (Course course : sCourses) { out.println("Course Id: " + course.getcId() +" | Course instructor : "+ course.getcInstructorName()+" | course name: "+ course.getcName()); } } break; case 2: default: out.println("Goodbye!"); } }}
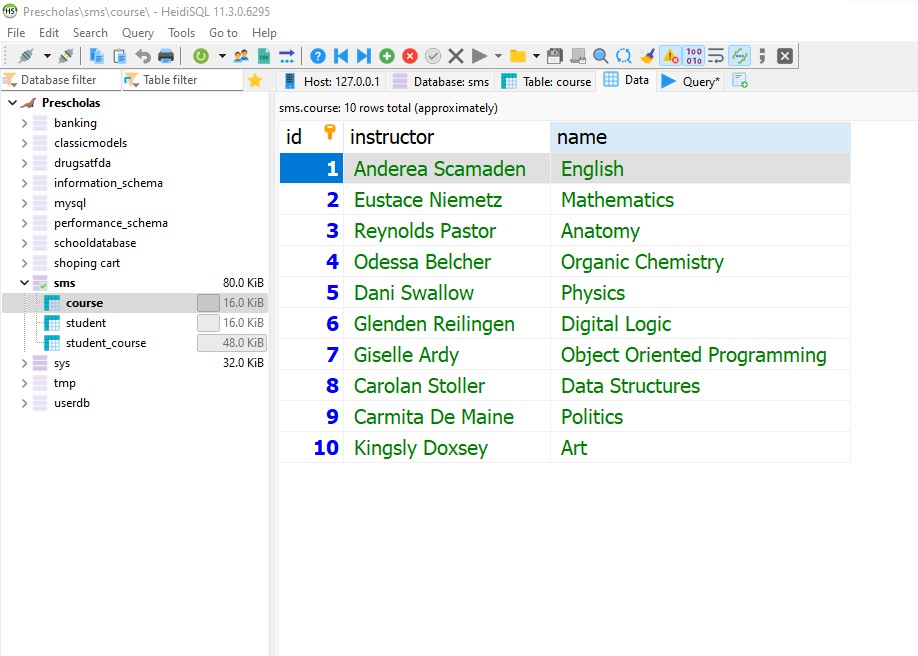
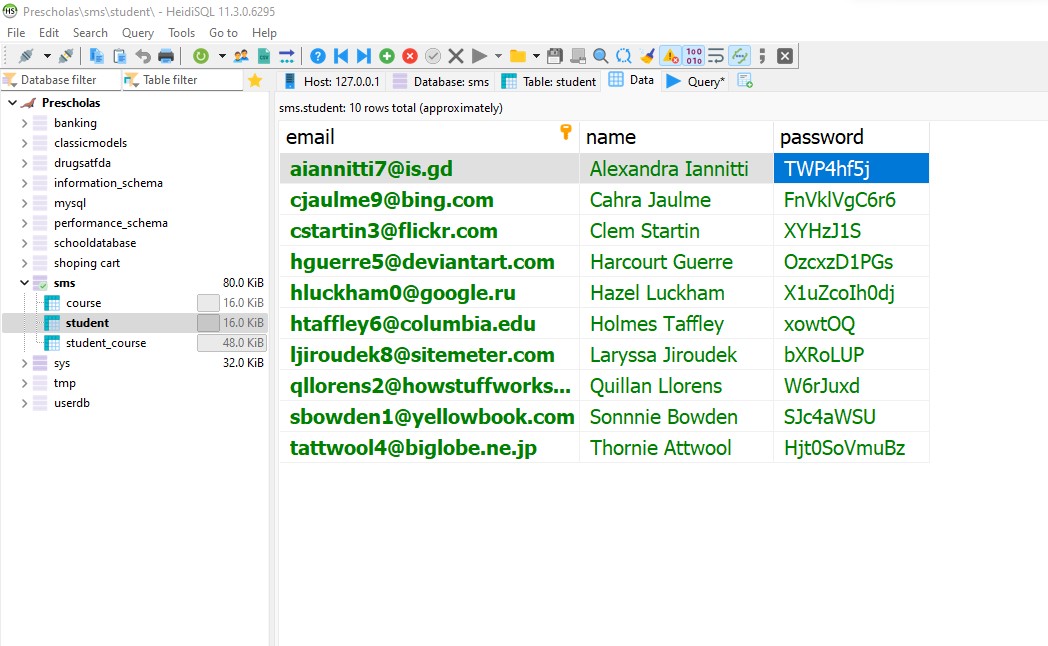
HS Prescholas\sms\coursel - HeidiSQL 11.3.0.6295 File Edit Search Query Tools Go to Help Database filter Prescholas banking > classicmodels drugsatfda information_schema mysql Table filter performance_schema schooldatabase shoping cart sms course student student_course sys tmp userdb 80.0 KiB 16.0 KiB 16.0 KiB 48.0 KiB 32.0 KiB XI OKH Host: 127.0.0.1 Database: sms sms.course: 10 rows total (approximately) id instructor 1 Anderea Scamaden 2 Eustace Niemetz 3 Reynolds Pastor 4 Odessa Belcher 5 Dani Swallow 6 Glenden Reilingen 7 Giselle Ardy 8 Carolan Stoller 9 10 Kingsly Doxsey Carmita De Maine Table: course Data 100 010 Query* IN X name English Mathematics Anatomy Organic Chemistry Physics Digital Logic Object Oriented Programming Data Structures Politics Art
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Lets break down what needs to be done in this method 1 Check if the student is already registered fo...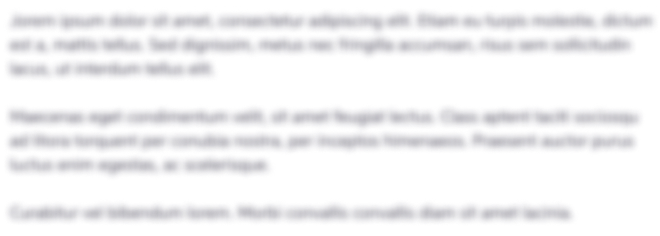
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started