Question
For question 3 and 4, thank you Coding (finish in 60min) - just try to finish as many as you can 1. Add an overloaded
For question 3 and 4, thank you
Coding (finish in 60min) - just try to finish as many as you can
1. Add an overloaded assignment operator to the Square class (this is already done, included in the given code for your reference. So, there is nothing you need to do here).
2. Add a "<" operator for Square objects, which returns "true" when a Square object's size is smaller than the compared one; false otherwise;
3. Does the Square class need an overloaded assignment operator? Why or why not? Answer this as a comment in the code.
4. Add an overloaded assignment operator to the SquareContainer class, using the copy() and clear() methods.
(Optional) Add a stream output operator to the Square class to print out the size of the square.
(Optional) Add a stream output operator to the SquareContainer class to print out the size of each square in the container.
Update "ClassTest.cpp" to test your updated classes.
Further Take away for Exercise 3 Operator overload Friend classes and operator overload Start thinking about the future, need for copy constructor and assignment operator overload
ClassTest.cpp
#include
#include "Square.h"
#include "SquareContainer.h"
using namespace std;
int main (int argc, char * const argv[]) {
SquareContainer c;
for (int i = 0; i < 20; i++) {
Square s;
s.setSize(i);
c.insertNext(s);
}
SquareContainer d(c); // copy
SquareContainer e = d; // assignment
try {
for (int i = 0; i < 21; i++) {
cout << "Square " << i << " size is "
<< c.deleteLast().getSize() << endl;
}
}
catch (SquareContainerException sqe) {
cerr << sqe.what();
}
return 0;
}
Square.cpp
/*
* Square.cpp
* Class-example
*
*/
#include "Square.h"
void Square::setSize(int newSize)
{
theSize = newSize;
}
int Square::getSize(void) const
{
return theSize;
}
Square& Square::operator = (const Square& other)
{
theSize = other.getSize();
return *this;
}
Square.h
/*
* Square.h
* Class-example
*
*/
#ifndef SQUARE_H
#define SQUARE_H
class Square {
public:
void setSize(int newSize);
int getSize(void) const;
Square& operator = (const Square&);
private:
int theSize;
};
#endif
SquareContainer.cpp
/*
* SquareContainer.cpp
* Class-example
*
*/
#include "SquareContainer.h"
const unsigned DEFAULTSIZE = 10;
// Plain constructor
SquareContainer::SquareContainer() : currentSize(DEFAULTSIZE), nextItem(0)
{
theSquares = new Square[currentSize];
}
// Copy constructor
SquareContainer::SquareContainer(const SquareContainer& old)
: currentSize(old.currentSize), nextItem(old.nextItem)
{
theSquares = new Square[currentSize];
copy(theSquares, old.theSquares);
}
// Destructor
SquareContainer::~SquareContainer()
{
clear();
}
// Insert an item in next location, reallocating storage if necessary
void SquareContainer::insertNext(Square item)
{
// Check if we have room
if (nextItem < currentSize) {
// If we have room, insert item
theSquares[nextItem++] = item;
} else {
// If not, reallocate storage, copy old contents to new, then inert new item
unsigned newSize = currentSize*2;
Square* newStorage = new Square[newSize];
copy(newStorage, theSquares);
newStorage[nextItem++] = item;
currentSize = newSize;
delete [] theSquares;
theSquares = newStorage;
}
}
// Delete an item at last location, returning a copy, but not a reference
Square SquareContainer::deleteLast(void)
{
// It's an array, and we never shrink it, so all we need to do is grab
// the item and decrement nextItem (assuming there's an item to delete).
if (nextItem == 0) {
throw SquareContainerException("SquareContainer: Attempt to delete when empty.");
}
return theSquares[--nextItem];
}
// Number of items in the container
unsigned SquareContainer::size(void) const
{
return nextItem;
}
// Current container capacity
unsigned SquareContainer::capacity(void) const
{
return currentSize;
}
// Utility function: copy contents of current object to new storage
// "to" must be allocated at least as much memory as theSquares
void SquareContainer::copy(Square* to, Square* from)
{
for (int i = 0; i to[i] = from[i]; } } // Delete dynamically allocated storage for this object void SquareContainer::clear(void) { for (int i=0; i theSquares[i].setSize(0); } delete [] theSquares; theSquares = NULL; nextItem = currentSize = 0; } SquareContainer.h /* * SquareContainer.h * Class-example * */ #ifndef SQUARECONTAINER_H #define SQUARECONTAINER_H #include #include #include "Square.h" using namespace std; class SquareContainerException : public out_of_range { public: SquareContainerException(const string& message = "") : out_of_range(message.c_str()) { } }; class SquareContainer { public: // Plain constructor SquareContainer(); // Copy constructor SquareContainer(const SquareContainer& old); // Destructor ~SquareContainer(); // Insert an item in next location, reallocating storage if necessary void insertNext(Square item); // Delete an item at last location, returning a copy Square deleteLast(void); // Number of items in the container unsigned size(void) const; // Current container capacity unsigned capacity(void) const; private: // Utility functions // Utility function: copy contents of current object to new storage // "to" must be allocated at least as much memory as theSquares void copy(Square* to, Square* from); // Delete dynamically allocated storage for this object void clear(void); // Dynamically allocated array Square* theSquares; // Where we are and how big we are unsigned nextItem, currentSize; }; #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
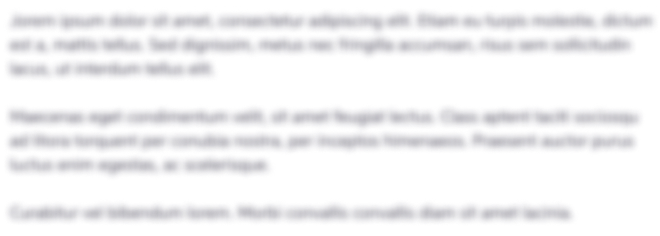
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started