Question
For the below JAVA Code, there appears to be an error in lines 121 (billamountstring) and 114 (calculate and display): package edu.rowan.ex4_1; import android.os.Bundle; import
For the below JAVA Code, there appears to be an error in lines 121 (billamountstring) and 114 (calculate and display):
package edu.rowan.ex4_1; import android.os.Bundle; import androidx.appcompat.app.AppCompatActivity; import androidx.appcompat.widget.Toolbar; import android.view.View; import android.view.Menu; import android.view.MenuItem; import java.text.NumberFormat; import android.view.KeyEvent; import android.view.View.OnClickListener; import android.view.inputmethod.EditorInfo; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.TextView.OnEditorActionListener; import android.content.SharedPreferences; import android.content.SharedPreferences.Editor; public class MainActivity extends AppCompatActivity implements OnEditorActionListener, OnClickListener{ // define variables for the widgets private EditText billAmountEditText; private TextView percentTextView; private Button percentUpButton; private Button percentDownButton; private TextView tipTextView; private TextView totalTextView; // define the SharedPreferences object private SharedPreferences savedValues; // define instance variables that should be saved private String billAmountString = ""; private float tipPercent = .15f; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Toolbar toolbar = findViewById(R.id.toolbar); setSupportActionBar(toolbar); // get references to the widgets billAmountEditText = (EditText) findViewById(R.id.billAmountEditText); percentTextView = (TextView) findViewById(R.id.percentTextView); percentUpButton = (Button) findViewById(R.id.percentUpButton); percentDownButton = (Button) findViewById(R.id.percentDownButton); tipTextView = (TextView) findViewById(R.id.tipTextView); totalTextView = (TextView) findViewById(R.id.totalTextView); // set the listeners billAmountEditText.setOnEditorActionListener(this); percentUpButton.setOnClickListener(this); percentDownButton.setOnClickListener(this); // get SharedPreferences object savedValues = getSharedPreferences("SavedValues", MODE_PRIVATE); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } @Override public void onPause() { // save the instance variables Editor editor = savedValues.edit(); editor.putString("billAmountString", billAmountString); editor.putFloat("tipPercent", tipPercent); editor.commit(); super.onPause(); } @Override public void onResume() { super.onResume(); // get the instance variables billAmountString = savedValues.getString("billAmountString", ""); tipPercent = savedValues.getFloat("tipPercent", 0.15f); // set the bill amount on its widget billAmountEditText.setText(billAmountString); // calculate and display calculateAndDisplay(); } public void calculateAndDisplay() { // get the bill amount billAmountString = billAmountEditText.getText().toString(); float billAmount = Float.parseFloat(billAmountString); // calculate tip and total float tipAmount = billAmount * tipPercent; float totalAmount = billAmount + tipAmount; // display the other results with formatting NumberFormat currency = NumberFormat.getCurrencyInstance(); tipTextView.setText(currency.format(tipAmount)); totalTextView.setText(currency.format(totalAmount)); NumberFormat percent = NumberFormat.getPercentInstance(); percentTextView.setText(percent.format(tipPercent)); } @Override public boolean onEditorAction(TextView v, int actionId, KeyEvent event) { if (actionId == EditorInfo.IME_ACTION_DONE || actionId == EditorInfo.IME_ACTION_UNSPECIFIED) { calculateAndDisplay(); } return false; } @Override public void onClick(View v) { switch (v.getId()) { case R.id.percentDownButton: tipPercent = tipPercent - .01f; calculateAndDisplay(); break; case R.id.percentUpButton: tipPercent = tipPercent + .01f; calculateAndDisplay(); break; } } }
CAN YOU USE A TRY/CATCH STATEMENT TO CORRECT THIS ERROR?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
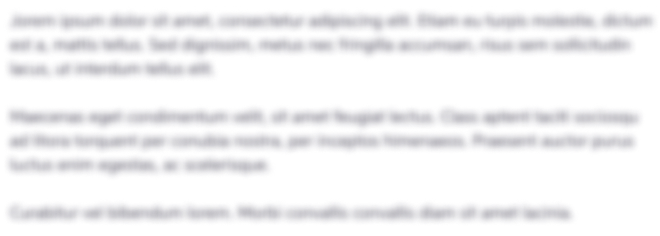
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started