Question
For the following C++ program utilizing a singly linked list, convert the list into a doubly linked list. Add a new command of ShowReverse to
For the following C++ program utilizing a singly linked list, convert the list into a doubly linked list. Add a new command of ShowReverse to display the list in reverse order. Update the methods that are affected by the doubly linked list implementation such as the delete and add methods to update both next and previous pointers.
LinkedUsers.h
#ifndef LinkedUsers_H
#define LinkedUsers_H
#include
#include
#include
#include
using namespace std;
class User {
string username;
string password;
string type;
string email;
User* next;
public:
User() {
username = "";
password = "";
type = "";
email = "";
next = NULL;
}
User(string uname, string pwd, string typ, string em) {
username = uname;
password = pwd;
type = typ;
email = em;
next = NULL;
}
void setNext(User* nextptr) {
next = nextptr;
}
User* getNext() {
return next;
}
string getUsername() {
return username;
}
string getEmail() {
return email;
}
string getType() {
return type;
}
string getPassword() {
return password;
}
bool checkPassword(string pwd) {
if (password == pwd)
return true;
return false;
}
};
User* login(User* head, string uname, string pwd) {
User* curr = head->getNext();
while (curr != NULL) {
if (uname == curr->getUsername() && curr->checkPassword(pwd)) {
return curr;
}
curr = curr->getNext();
}
return NULL;
}
void show(User* curr) {
while (curr != NULL) {
cout << "USERNAME(" << curr->getUsername() << ") EMAIL(" << curr->getEmail() << ") ";
curr = curr->getNext();
}
}
void add(User* head) {
cout << "Please enter username, password, role and email: ";
string eachLine;
string uname, pwd, typ, em;
cin >> uname >> pwd >> typ >> em;
User* newuser = new User(uname, pwd, typ, em);
User* curr = head;
while (curr->getNext() != NULL) {
curr = curr->getNext();
}
curr->setNext(newuser);
cout << "Successfully added ";
}
void deleteuser(User* head) {
string name;
cout << "Please enter the username: ";
cin >> name;
User* curr = head->getNext();
User* prev = head;
while (curr != NULL) {
if (name == curr->getUsername()) {
prev->setNext(curr->getNext());
delete(curr);
cout << "Successfully deleted ";
return;
}
curr = curr->getNext();
prev = prev->getNext();
}
cout << "Not found ";
}
void saveToFile(char* fname, User* curr) {
ofstream fp(fname);
while (curr != NULL) {
fp << curr->getUsername() << " " << curr->getPassword() << " " << curr->getType() << " " << curr->getEmail() << endl;
curr = curr->getNext();
}
fp.close();
}
#endif
Managing.cpp
#include
#include
#include "LinkedUsers.h"
#include
#include
using namespace std;
int main(int argc, char* argv[]) {
if (argc != 2) {
cout << "Invalid Syntax Usage: ManageLinkedUsers user-data-file-name ";
return 0;
}
User* head = new User();
ifstream fp(argv[1]);
if (fp.eof()) {
cout << "Empty file, exiting! ";
return 0;
}
string eachLine;
while (getline(fp, eachLine)) {
stringstream ss(eachLine);
string uname, pwd, typ, em;
ss >> uname >> pwd >> typ >> em;
User* newuser = new User(uname, pwd, typ, em);
User* curr = head;
while (curr->getNext() != NULL) {
curr = curr->getNext();
}
curr->setNext(newuser);
}
fp.close();
string uname;
string pwd;
cout << "username: ";
cin >> uname;
cout << "password: ";
cin >> pwd;
User* ptr = login(head, uname, pwd);
if (ptr == NULL) {
cout << "Invalid login ";
return 0;
}
cout << "Successful login ";
if (ptr->getType() == "reg") {
cout << "USERNAME(" << ptr->getUsername() << ") EMAIL(" << ptr->getEmail() << ") ";
return 0;
}
while (true) {
cout << "Enter a command (SHOW, ADD, DELETE, SAVE, EXIT): ";
string comm;
cin >> comm;
if (comm == "EXIT" || comm == "exit") {
cout << "Thank You ";
break;
}
else if (comm == "show" || comm == "SHOW") {
show(head->getNext());
}
else if (comm == "add" || comm == "ADD") {
add(head);
}
else if (comm == "DELETE" || comm == "delete") {
deleteuser(head);
}
else if (comm == "SAVE" || comm == "save") {
saveToFile(argv[1], head->getNext());
}
else {
cout << "Invalid Command ";
}
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
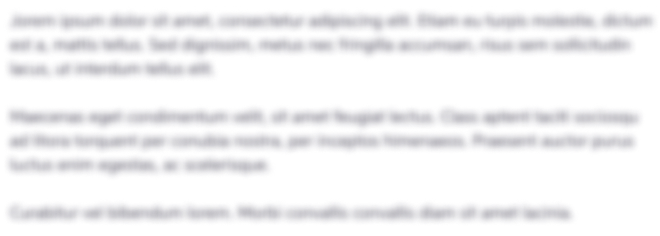
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started