Answered step by step
Verified Expert Solution
Question
1 Approved Answer
For the following linked list, I need to write a couple of functions: #include #include using namespace std; typedef string ItemType; struct Node { ItemType
For the following linked list, I need to write a couple of functions: #include#include using namespace std; typedef string ItemType; struct Node { ItemType value; Node *next; }; class LinkedList { private: Node *head; public: // default constructor LinkedList() : head(nullptr) { } // copy constructor LinkedList(const LinkedList& rhs); // Destroys all the dynamically allocated memory // in the list. ~LinkedList(); // assignment operator const LinkedList& operator=(const LinkedList& rhs); // Inserts val at the front of the list void insertToFront(const ItemType &val); // Prints the LinkedList void printList() const; // Sets item to the value at position i in this // LinkedList and return true, returns false if // there is no element i bool get(int i, ItemType& item) const; // Reverses the LinkedList void reverseList(); // Prints the LinkedList in reverse order void printReverse() const; // Appends the values of other onto the end of this // LinkedList. void append(const LinkedList &other); // Exchange the contents of this LinkedList with the other one. void swap(LinkedList &other); // Returns the number of items in the Linked List. int size() const; };
I have already written all the functions but append and swap.
Swap should print like this:
Here's an example of the swap function:
LinkedList e1; e1.insertToFront("A"); e1.insertToFront("B"); e1.insertToFront("C"); e1.insertToFront("D"); LinkedList e2; e2.insertToFront("X"); e2.insertToFront("Y"); e2.insertToFront("Z"); e1.swap(e2); // exchange contents of e1 and e2 string s; assert(e1.size() == 3 && e1.get(0, s) && s == "Z"); assert(e2.size() == 4 && e2.get(2, s) && s == "B");
and append should print like this:
LinkedList e1; e1.insertToFront("bell"); e1.insertToFront("biv"); e1.insertToFront("devoe"); LinkedList e2; e2.insertToFront("Andre"); e2.insertToFront("Big Boi"); e1.append(e2); // adds contents of e2 to the end of e1 string s; assert(e1.size() == 5 && e1.get(3, s) && s == "Big Boi"); assert(e2.size() == 2 && e2.get(1, s) && s == "Andre");
Step by Step Solution
There are 3 Steps involved in it
Step: 1
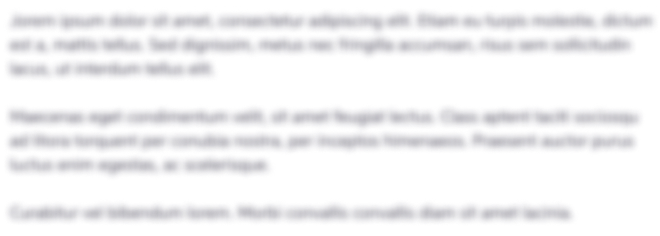
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started