Question
For this assignment I am working with 2 python files and they will have syntax and logic errors in them. The first file is the
For this assignment I am working with 2 python files and they will have syntax and logic errors in them.
The first file is the ReminderApp.pv and is essentially to input the code from the other file and run it. There are no errors here it is simply to run the code.
from Reminder import * def main_setup(file_name): agenda = Agenda() general_list = agenda.add_list("General") grocery_list = agenda.add_list("Grocery Store") books_list = agenda.add_list("Books") general_list.add_reminder("Pick up flowers", agenda.PRIORITY_HIGH, "02/13/2022") general_list.add_task("Clean garage", agenda.PRIORITY_LOW) general_list.add_reminder("Start working on final project", agenda.PRIORITY_HIGH, "02/14/2022") general_list.add_reminder("Bindge 'The Office' Season 1", agenda.PRIORITY_NONE, "03/07/2022") grocery_list.add_task("Milk", agenda.PRIORITY_NONE) grocery_list.add_task("Eggs", agenda.PRIORITY_NONE) grocery_list.add_task("Bread", agenda.PRIORITY_NONE) grocery_list.add_task("Waffles", agenda.PRIORITY_NONE) grocery_list.add_task("Bananas", agenda.PRIORITY_NONE) books_list.add_reminder("Drop off library books", agenda.PRIORITY_MEDIUM, "02/15/2022") books_list.add_reminder("Books Galore Annual Clearance", agenda.PRIORITY_HIGH, "03/01/2022") books_list.add_task("Pick next book club selection", agenda.PRIORITY_MEDIUM) agenda.save(file_name) def main(): agenda = Agenda() file_name = "myagenda.dat" main_setup( file_name ) agenda.load( file_name ) agenda.print_list("General") print() agenda.print_list("Grocery Store") print() agenda.print_list("Books") main()
This is what the output needs to look like
General List: ------------- [3] Pick up flowers (02/13/2022) [1] Clean garage [3] Start working on final project (02/14/2022) Bindge 'The Office' Season 1 (03/07/2022) Grocery Store List: ------------------- Milk Eggs Bread Waffles Bananas Books List: ----------- [2] Drop off library books (02/15/2022) [3] Books Galore Annual Clearance (03/01/2022) [2] Pick next book club selection
This is the Reminder.pv file and is the file that has the errors in it.
class Reminder: def __init__( self, title, priority, due_date ): self.title = title self.priority = priority self.due_date = due_date def get_title( self ): return self.title def get_priority( self ): return self.priority def get_due_date( self ): return self.due_date def __repr__( self ): return ",".join([self.title, self.priority, str("None" if self.due_date is None else self.due_date)]) def __str__( self ): if (self.due_date == None): return "{1}{0}".format(self.title, str("" if (self.priority == 0) else "[" + self.priority + "] ")) else: return "{1}{0} ({2})".format(self.title, str("" if (self.priority == 0) else "[" + self.priority + "] "), self.due_date) class ReminderList: def __init__( self, list_name ): self.list_name = list_name self.reminders = [] def get_list_name( self ): return self.list_name def add_reminder( self, title, priority, due_date ): self.reminders.append( Reminder(title, priority, due_date) ) def remove_reminder( self, reminder ): self.reminders.remove(reminder) def get_reminders( self ): return self.reminders def add_task( self, title, priority ): self.reminders.append( Reminder(title, priority, None) ) class Agenda: PRIORITY_NONE = 0 PRIORITY_LOW = 1 PRIORITY_MEDIUM = 2
PRIORITY_HIGH = 3 def __init__( self ): pass self.reminder_lists = [] def add_list( self, title ): new_list = ReminderList(title) self.reminder_lists.append( new_list ) return new_list def print_list( self, title ): reminder_list = self.get_reminder_list(title) if reminder_list == None: print(f"Could not find list {title}") return print(f"{title} List:") print( "-" * len(title) + 6) for item in reminder_list.get_reminders(): print(item) # TODO: remove str add + " " def get_reminder_list( self, title ): for reminder_list in self.reminder_lists: if reminder_list.get_list_name() == title: return reminder_list return None def load( self, filename ): agenda_data = open(filename, 'r') for agenda_item in agenda_data: if agenda_item[0] == 'L': fields = agenda_item[1:].split(',') self.add_list( fields[0] ) if agenda_item[0] == 'R': fields = agenda_item[1:].split(',') reminder_list = self.get_reminder_list(fields[0]) if (reminder_list != None): reminder_list.add_reminder( fields[1],fields[2],fields[3] ) else: print(f"Could not find {fields[0]} as a list.") if agenda_item[0] == 'T': fields = agenda_item[1:].strip().split(',') reminder_list = self.get_reminder_list(fields[0]) if (reminder_list != None): reminder_list.add_task( fields[1],fields[2] ) else: print(f"Could not find {fields[0]} as a list.") agenda_data.close() def save( self, filename): agenda_data = open(filename, 'w')
for reminder_list in self.reminder_lists: agenda_data.write("L{} ".format( reminder_list.get_list_name() )) for reminder in reminder_list.get_reminders(): if (reminder.get_due_date() == None): agenda_data.write("T{},{},{}\ n".format(reminder_list.get_list_name(), reminder.get_title(), reminder.get_priority() )) else: agenda_data.write("R{},{},{},{}\ n".format(reminder_list.get_list_name(), reminder.get_title(), reminder.get_priority(), reminder.get_due_date())) agenda_data.flush() agenda_data.close()
Please note where the errors are and how I can fix them. Additionally sorry for the spacing errors please bear with me. Let me know where the errors are and how to fix them I am having trouble spotting the problems.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
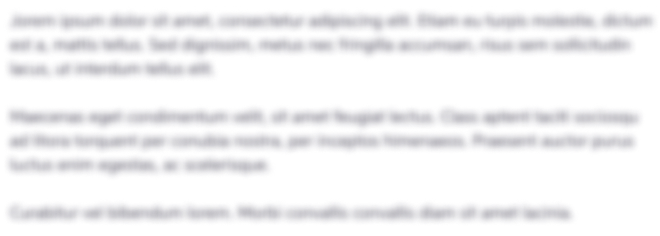
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started