Question
For this assignment you will create a set of simple classes that model a system that maintains a collection of library assets. The system utilizes
For this assignment you will create a set of simple classes that model a system that maintains a collection of library assets. The system utilizes abstract classes and polymorphism to enable an asset manager to keep track of books and periodicals as a single collection. Books are able to be checked out whereas periodicals cannot.
In addition to the classes described below, you will also need to provide a test driver - a separate class with a main() - to exercise the functionality of your system.
You will need to implement the following classes based on the description of their attributes and operations:
- Asset - this class must be declared abstract, attributes are all private
- name - the name of the asset
- id - the unique id of the asset
- isAvailable - a flag to indicate whether the asset is currently in the library
- a constructor that requires the creator to supply the id of the asset and appropriately initializes an object
- public operations to set and get the asset's name
- public operation to get the asset's id
- protected operation to get whether or not the asset is in the library
- public, abstract operation to check out the asset, returns a boolean
- public, abstract operation to check in the asset, returns a boolean
- Book - this class is a subclass of Asset
- authors - a collection (array) of the names the books authors
- authorCount - the number of authors currently in the collection
- a constructor that requires the creator to supply the id of the asset and appropriately initializes an object
- public operations to add an author to the collection of authors and obtain the collection (array) of authors
- an implementation of the the check out operation
- if the asset is in the library, it is made unavailable and the operation returns true
- if the asset is not in the library the operation returns false
- an implementation of the the check out operation
- if the asset is not the library, it is made available and the operation returns true
- if the asset is already in the library the operation returns false
- an override of toString() which returns a description of the book of the format
- "
" by , , ... ::
- "Introduction to Algorithms" by Cormen, Leiserson, Rivest :: 123456
- "
- Periodical - this class is a subclass of Asset
- volume - the volume number of the periodical
- issue - the issue number of the periodical
- a constructor that requires the creator to supply the id of the asset and appropriately initializes an object
- public operations to set and get the volume number
- public operations to set and get the issue number
- an implementation of the the check out operation
- periodicals can't be checked out so this operation always returns false
- an implementation of the the check out operation
- since periodicals cannot be checked out this operation always returns true
- an override of toString() which returns a description of the book of the format
- "
", : ::
- "Nature" 493:2 :: 9876543
- "
- AssetManager
- assets - a collection (array) of assets, no two assets have the same id
- assetCount - the number of assets currently in the collection
- a constructor (no parameters) that appropriately initializes the object
- public operation to add an asset, returns a boolean
- if there is no asset in the collection with the same id, the asset is added and true is returned
- if there is an asset in the collection with the same id, the asset is not added and false is returned
- public operation to find an asset by id
- if an asset with the id is in the collection it is returned
- if no asset with the id is in the collection null is returned
Your submission will consist of a zip file containing just the necessary .java files. As always your code must meet the coding standards.
Previous
Step by Step Solution
There are 3 Steps involved in it
Step: 1
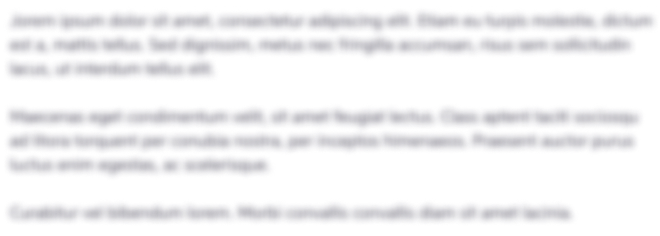
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started