Question
For this assignment, you will need to create 2 separate programs (code is attached). Run the server first then run the client. The port number
For this assignment, you will need to create 2 separate programs (code is attached). Run the server first then run the client. The port number used is 6789 so if you are experiencing problems try to open that port. EXAMING THE CODE CAREFULLY TO SEE WHAT EACH STATEMENT IS DOING! If you are using 2 computers then you will need to get the IP address of the server using IPCONFIG at the command prompt (ifconfig for linux) and enter it in the socket constructor statement instead of the loopback address 127.0.0.1.
When complete upload a screenshot of the running program (with the messages shown - use your name for message)
Extra Credit Challenge (2 pts) can you put it in a windowbuilder GUI?
Client program: ChatClient.java
import java.io.*;
import java.net.*;
public class ChatClient
{
public static void main(String[] args) throws Exception
{
Socket sock = new Socket("127.0.0.1",6789);
// reading from keyboard (keyRead object)
BufferedReader keyRead = new BufferedReader(new InputStreamReader(System.in));
// sending to client (pwrite object)
OutputStream ostream = sock.getOutputStream();
PrintWriter pwrite = new PrintWriter(ostream, true);
// receiving from server ( receiveRead object)
InputStream istream = sock.getInputStream();
BufferedReader receiveRead = new BufferedReader(new InputStreamReader(istream));
System.out.println("To start the chat, type and press Enter key");
String receiveMessage, sendMessage;
while(true)
{
sendMessage = keyRead.readLine(); // keyboard reading
pwrite.println(sendMessage); // sending to server
pwrite.flush(); // flush the data
if((receiveMessage = receiveRead.readLine()) != null) //receive from server
{
System.out.println(receiveMessage); // displaying at DOS prompt
}
}
}
}
// Note: To come out of the chat, type Ctrl+C.
Server program: ChatServer.java
import java.io.*;
import java.net.*;
public class ChatServer
{
public static void main(String[] args) throws Exception
{
ServerSocket sersock = new ServerSocket(6789);
System.out.println("Server ready for chatting");
Socket sock = sersock.accept( );
// reading from keyboard (keyRead object)
BufferedReader keyRead = new BufferedReader(new InputStreamReader(System.in));
// sending to client (pwrite object)
OutputStream ostream = sock.getOutputStream();
PrintWriter pwrite = new PrintWriter(ostream, true);
// receiving from server ( receiveRead object)
InputStream istream = sock.getInputStream();
BufferedReader receiveRead = new BufferedReader(new InputStreamReader(istream));
String receiveMessage, sendMessage;
while(true)
{
if((receiveMessage = receiveRead.readLine()) != null)
{
System.out.println(receiveMessage);
}
sendMessage = keyRead.readLine();
pwrite.println(sendMessage);
pwrite.flush();
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
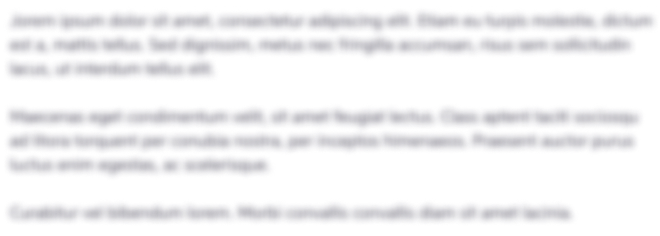
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started