Question
For this assignment, you will simulate the dice game called Shut the Box. Please code in Java and use proper javadoc documentation. Shut the box
For this assignment, you will simulate the dice game called Shut the Box. Please code in Java and use proper javadoc documentation.
Shut the box is played as follows:
The board consists of nine numbered (1-9) boxes that start open and can be closed.
There can be any number of players.
The order of play will be randomly determined.
On the players turn, the player rolls two dice. He then must close a combination of boxes that add up to the total rolled.
As long as the player is able to find such a combination, the players turn continues.
Once a player cannot find a combination of open boxes adding to the total rolled, the players turn is over.
When a players turn ends, the player's score is equal to the sum of the still open boxes.
Play then continues with the next player.
When all players have completed their turn, the player with the lowest score is declared the winner.
If any player is able to close all the boxes during their turn, he has shut the box and is immediately declared the winner.
Notes:
Think through this problem in an object oriented fashion.
Go through the nouns and verbs in the description (as demonstrated in class and chapter 15) to try to determine what classes you think you will need.
Be very systematic in planning and coding. Do it piece by piece.
You will need to implement an option to have a computer player.
The computer player can use one of two strategies.
Always picks the highest available box(es) that works for the combination.
Picks a combination of boxes that works at random.
You should have a ShutTheBox class that has a start method. This class will be responsible for guiding game play.
Use inheritance where appropriate.
You can use the Die classes provided.
Die Classes:
import java .util.Random;
public abstract class Die {
int numSides;
private static Random generator = new Random();
public Die (int s)
{
numSides = s;
}
public int roll()
{
return generator.nextInt(6) + 1;
}
abstract public void display(int side);
}
public class Driver {
public static void main(String[] args) {
Die d = new SixSidedDie();
int roll1=0, roll2=0, roll3=0, roll4=0, roll5=0, roll6=0, other=0;
for (int i = 1; i <= 10000000; i++)
{
switch(d.roll())
{
case 1: roll1 = roll1 + 1;
break;
case 2: roll2 = roll2 + 1;
break;
case 3: roll3 = roll3 + 1;
break;
case 4: roll4 = roll4 + 1;
break;
case 5: roll5 = roll5 + 1;
break;
case 6: roll6 = roll6 + 1;
break;
default: other = other + 1;
}
}
System.out.println("Rolled 1:" + roll1/10000000.0);
System.out.println("Rolled 2:" + roll2/10000000.0);
System.out.println("Rolled 3:" + roll3/10000000.0);
System.out.println("Rolled 4:" + roll4/10000000.0);
System.out.println("Rolled 5:" + roll5/10000000.0);
System.out.println("Rolled 6:" + roll6/10000000.0);
System.out.println("Rolled other:" + other/1000000.0);
}
}
public class SixSidedDie extends Die {
public SixSidedDie()
{
super(6);
}
public void display(int sides)
{
System.out.println("*******");
System.out.println("* *");
System.out.println("* " + sides + " *");
System.out.println("* *");
System.out.println("*******");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
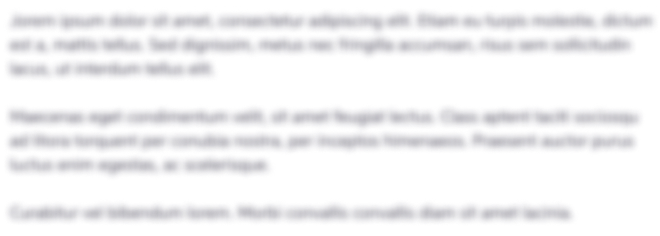
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started