Answered step by step
Verified Expert Solution
Question
1 Approved Answer
for this lab, you will be implementing both a * Last In/First Out * and * First In/First Out * data structures. These data structures
for this lab, you will be implementing both a *Last In/First Out* and *First In/First Out* data structures. These data structures store data in a way that allows you to only see one value from the structure. You can't see any other value in the structure unless it is the top value. These structures are important because they allow us to add or remove data members to the structure in an O(1) complexity. ### Lab Instructions Implement each of the functions to perform the necessary actions outlined in the `.h` files. As you are writing your functions, read the instructions and think of how you would test that functions while you are writing it. Write your Test first and then implement your functions. Try running your test and then fixing your issues. `lifo_storage` and `fifo_storage` will both be statically sized, meaning that you don't need to worry about dynamically growing the stringVector. Treat it just like an array. #### Fifo #### **fifo()**: Default constructor. Set index properly and reserve 100 spaces in fifo_storage **explicit fifo(std::string input_string)**: Constructor that does the same thing as the default constructor, but adds a single item to the Fifo **fifo(const fifo &original)**: Copy constructor **virtual ~fifo()**: Destructor **fifo &operator=(const fifo &right)**: Assignment operator **bool is_empty() const**: Return true if the fifo is empty and false if it is not **unsigned size() const**: Return the size of the fifo **std::string top() const**: Return the front string of the fifo. **void enqueue(std::string input)**: Add input string to the back of the fifo **void dequeue()**: Remove the front string from the fifo #### Lifo #### **lifo()**: Default constructor. Set index properly and reserve 100 spaces in fifo_storage **explicit lifo(std::string input_string)**: Constructor that does the same thing as the default constructor, but adds a single item to the Fifo **lifo(const lifo &original)**: Copy constructor **virtual ~lifo()**: Destructor **lifo &operator=(const lifo &right)**: Assignment operator **bool is_empty() const**: Return true if the lifo is empty and false if it is not **unsigned size() const**: Return the size of the lifo **std::string top() const**: Return the top of the lifo. **void push(std::string input)**: Add input string to the top of the string **void pop()**: Remove the top string from the lifo.
fifo.h
#ifndef CMPE126S18_LABS_QUEUE_H #define CMPE126S18_LABS_QUEUE_H #include "stringVector.h" namespace lab3 { class fifo { lab2::stringVector fifo_storage; unsigned front_index; unsigned back_index; public: fifo(); //Default constructor. Reserve 100 spaces in lifo_storage explicit fifo(std::string input_string); //Create new fifo from string input fifo(const fifo &original); //Copy constructor virtual ~fifo(); //Destructor fifo &operator=(const fifo &right); //Assignment operator bool is_empty() const; // Return true if the fifo is empty and false if it is not unsigned size() const; // Return the size of the fifo std::string top() const; // Return the front string of the fifo. void enqueue(std::string input); // Add input string to the back of the fifo void dequeue(); // Remove the front string from the fifo }; }
fifo.cpp
#include "fifo.h" namespace lab3{ fifo::fifo() { //Reserve 100 spaces in fifo_storage } fifo::fifo(std::string input_string) { } fifo::fifo(const fifo &original) { } fifo::~fifo() { } fifo &fifo::operator=(const fifo &right) { //return <#initializer#>; } bool fifo::is_empty() const { //return false; } unsigned fifo::size() const { //return 0; } std::string fifo::top() const { //return std::__cxx11::string(); } void fifo::enqueue(std::string input) { } void fifo::dequeue() { } }
lifo.h
#ifndef CMPE126S18_LABS_STACK_H #define CMPE126S18_LABS_STACK_H #include "stringVector.h" namespace lab3 { class lifo { lab2::stringVector lifo_storage; unsigned index; public: lifo(); //Default constructor. Reserve 100 spaces in lifo_storage explicit lifo(std::string input_string); //Create new lifo from string input lifo(const lifo &original); //Copy constructor virtual ~lifo(); //Destructor lifo &operator=(const lifo &right); //Assignment operator bool is_empty() const; // Return true if the lifo is empty and false if it is not unsigned size() const; // Return the size of the lifo std::string top() const; // Return the top of the lifo. void push(std::string input); // Add input string to the top of the string void pop(); // Remove the top string from the lifo }; }
lifo.cpp
#include "lifo.h" namespace lab3{ lifo::lifo() { //Reserve 100 spaces in lifo_storage } lifo::lifo(std::string input_string) { } lifo::lifo(const lifo &original) { } lifo::~lifo() { } lifo &lifo::operator=(const lifo &right) { //return <#initializer#>; } bool lifo::is_empty() const { //return false; } unsigned lifo::size() const { //return 0; } std::string lifo::top() const { //return std::__cxx11::string(); } void lifo::push(std::string input) { } void lifo::pop() { } }
stringVector.cpp
#include "../inc/stringVector.h" #includenamespace lab2 { int stringCompare(std::string str1, std::string str2); stringVector::stringVector() { data = nullptr; length = 0; allocated_length = 0; } stringVector::~stringVector() { delete[]data; } unsigned stringVector::size() { return length; //return ; } unsigned stringVector::capacity() { return allocated_length; //return ; } void stringVector::reserve(unsigned new_size) { if (new_size == allocated_length) return; std::string *temp = new std::string[new_size]; for (int i = 0; i < new_size && i < length; i++) { temp[i] = data[i]; } if (allocated_length < length) { length = new_size; allocated_length = new_size; delete[] data; data = temp; } } void lab2::stringVector::set_size(unsigned new_size) { length = new_size; if(allocated_length != new_size) reserve(new_size); } bool stringVector::empty() { return (length == 0) ? true : false;//return ; } void stringVector::append(std::string new_data) { if (length < allocated_length) { data[length++] = new_data; } else { reserve(allocated_length == 0 ? 1 : 2 * allocated_length); } } void stringVector::swap(unsigned pos1, unsigned pos2) { std::string temp; //a temporary string to hold the data and switch it if ((pos1 >= length) || (pos2 >= length)) { std::cout << "index Out of bounds" << std::endl; return; } { temp = data[pos1]; data[pos1] = data[pos2]; data[pos2] = temp; } } stringVector &stringVector::operator=(stringVector const &rhs) { if (this == &rhs) return *this; delete[] data; length = rhs.length; allocated_length = rhs.allocated_length; this->data = new std::string[allocated_length]; for (int i = 0; i < length; i++) { this->data[i] = rhs.data[i]; } return *this; //return ; } std::string &stringVector::operator[](unsigned position) { if (position > allocated_length) { throw std::out_of_range("position out of range"); } return data[position]; } //return ; void stringVector::sort() { std::string temp; for (int index = length - 1; index > 0; index--) { for (int j = 0; j < index; j++) { if (stringCompare(data[j], data[j + 1]) > 0) { temp = data[j]; data[j] = data[j + 1]; data[j + 1] = temp; } } } } int stringCompare(std::string str1, std::string str2) { int pos; for (pos = 0; pos < str1.length() && pos < str2.length() && tolower(str1[pos]) == tolower(str2[pos]); ++pos); if (str1.length() < str2.length() && pos == str1.length()) return -1; else if (str2.length() < str1.length() && pos == str2.length()) return 1; else return pos == str1.length() ? 0 : tolower(str1[pos]) - tolower(str2[pos]); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
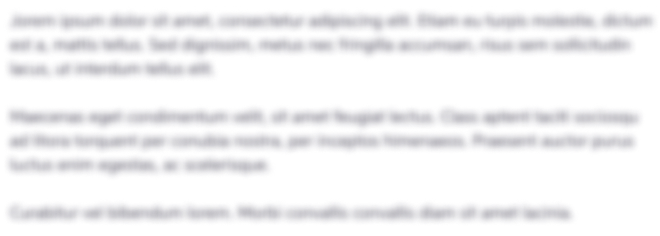
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started