Question
For this lab, you will create a 2D Array where you will be storing values of type integer, fill in the elements of that array
For this lab, you will create a 2D Array where you will be storing values of type integer, fill in the elements of that array by prompting the user for input then display the elements of the array. Then you will need to find the sum of each row of array and display it.
Step 1: Getting Started
At the beginning of each programming assignment you must have a comment block with the following information:
1. /*------------------------------------------------------------- |
2. // AUTHOR: your name. |
3. // FILENAME: title of the source file. |
4. // SPECIFICATION: your own description of the program. |
5. // FOR: CSE 110- Lab #11 |
6. // TIME SPENT: how long it took you to complete the assignment. |
7. //----------------------------------------------------------- */ |
Step 2: Import Libraries and Declare Variables You Need
1. import java.util.Scanner; |
2. |
3. public class Lab11 |
4. { |
5. public static void main(String[] args) |
6. { |
7. // ============================================================ |
8. // Step 2. Declaring Variables |
9. // |
10. // You might need four variables of the following types: |
11. // |
12. // - A Scanner object |
13. // - An integer for the number of rows |
14. // - An integer for the number of columns |
15. // - An integer for the sum of elements on a row |
16. |
17. int numRows = -1; |
18. int numCols = -1; |
19. |
20. } // End of the main function |
21. } // End of the Lab11 class |
After the documentation block, you will need to import Scanner from java.util. At the top of your main function, you can declare variables you need. You might need four
local variables of the following types:
- A Scanner
- An integer for the number of rows
- An integer for the number of columns
- An integer for the sum of elements on a row
You can declare extra variables if needed.
Step 3: Request Array Size from the User
1. // ============================================================ |
2. // Step 3. Request Array Size from the User |
3. |
4. // Print this message "Enter the number of rows in the array" |
5. // --> |
6. |
7. // Use your scanner to store the number of ROWS |
8. // --> |
9. |
10. // Print this message "Enter the number of columns in the array" |
11. // --> |
12. |
13. // Use your scanner to store the number of COLUMNS |
14. // --> |
15. |
Like Lab 9 and Lab 10, before creating an array we need to decide the size of it first (number of elements). Now we are going to create a 2D array which works like a table or a sheet, which has rows and columns. In this case, we will need two integers for the size, one is the number of rows and the other is the number of columns.
Please use your Scanner variable and .nextInt() method to request two integers from the user. Dont forget to prompt the users and they will know its their turn to input. You can find the prompt messages in the sample output (or the comments in templet file.)
Step 4: Declare a 2D integer Array
1. // ============================================================ |
2. // Step 4. Declare a 2D Array |
3. |
4. // Declare a 2D integer array using the number of rows and columns |
5. // previously entered by the user |
6. // |
7. // For a 1D array, we use one pair of brackets. For a 2D array, we |
8. // need two pairs of brackets. The first one is for "rows" and the |
9. // second one is for "columns". |
10. // |
11. // --> |
12. |
With the numbers of rows and columns, we are ready to declare a 2D array. In Lab 9 and Lab 10, we understand that an array type is denoted by brackets ([]). For a 1D array we use one pair of brackets. For a 2D array we use two pairs of brackets.
Here is an example to declare a variable of type 2D integer array:
1. int[][] my2DArray; Please note that this variable does not point to any real array instance now because we have
not instantiated an object. To instantiate an object of type 2D integer array, we can use
1. my2DArray = new int[numRows][numCols];
where numRows is my variable representing number of rows and numCols stands for number of columns. By convention, the first pair of brackets is for rows and the second one is for columns. However, just for your information, a 2D array in computer is actually stored like a 1D array. If you are interested in the implementation of 2D array, you may choose higher- level classes like Computer Organization in the future. For this class you only need to keep in mind the convention here.
Step 5: Fill in the Array by Loops
1. // ============================================================ |
2. // Step 5. Fill in the Array by Loops |
3. |
4. for(inti=0;i<=numRows-1;i++) |
5. { |
6. for (int j = 0; j <= numCols - 1; j++) |
7. { |
8. // Display the message: "Please enter a value for position (i, j)" |
9. // --> |
10. |
11. // Use your scanner to get an integer from the user and store it |
12. // at the position (i, j) of the 2D array you declared in Step 4. |
13. // --> |
14. } |
15. } |
16. |
Just like 1D array, we can use indices to access each element (or cell) in a 2D array. The intuition here is to fix a row index and iterate all possible column indices. Following this idea, we will need a nested loop structure for iterating all row indices (denoted as i) and column indices (denoted as j). Please implement such nested loop and ask the user for the content at each index pair (i, j).
Dont forget that the lowest index in Java is always 0 and the greatest index for an array is the size 1. Thus, the first row (or column) is labeled with index 0 and the last row (or column) is labeled as numRow 1 (or numCols 1).
You can find the prompt message in the sample output or in the comments of template file.
Step 6: Display the Elements in the Array
1. // ============================================================ |
2. // Step 6. Display the Elements in the Array |
3. // |
4. // Remember the outer for loop walks through the rows |
5. // while the inner for loop walks through the elements of the rows |
6. |
7. System.out.println(" Your 2D array looks like:"); |
8. |
9. for (int i = 0; i <= ?????????; i++) |
10. { |
11. for (int j = 0; j <= ?????????; j++) |
12. { |
13. // Print the element at (i, j) with a space in between each element |
14. // --> |
15. } |
16. // Print a new line for ith row |
17. // --> |
18. } |
To display all elements in 2D array, you will need a loop structure which is similar to the one in the previous stage. You are supposed to print the elements of one row on the same line and give it a line break after the row is complete. Please check the sample output for details.
Step 7: Calculating the Sum of Each Row and Show the Result
1. // ============================================================ |
2. // Step 7. Calculating the Sum of Each Row and Show the Result |
3. // |
4. // Don't forget to reset the variable holding the result |
5. |
6. System.out.println(" The summary of each row:"); |
7. |
8. for (int i = 0; i <= ?????????; i++) |
9. { |
10. // Reset the variable sum to 0 here |
11. // --> |
12. |
13. for (int j = 0; j <= ?????????; j++) |
14. { |
15. // Add value at (i, j) to sum |
16. // --> |
17. } |
18. |
19. // Display the sum like "Sum of the elements of row i is: X" |
20. // --> |
21. } |
Finally, please use another nested loop to find the sums of each rows. You can use the loop structure in the previous step. After finding the sum of one row, you are supposed to print it out like Sum of the elements of row i is: X, where i is a row index and X is the sum. Please check the sample output for details.
Sample Output
Below is an example of what your output should roughly look like when this lab is completed. Text in RED represents user input.
Sample Output 1
Enter the number of rows in the array
3
Enter the number of columns in the array
2
Please enter a value for position (0, 0)
3
Please enter a value for position (0, 1)
4
Please enter a value for position (1, 0)
1
Please enter a value for position (1, 1)
5
Please enter a value for position (2, 0)
7
Please enter a value for position (2, 1)
4
Your 2D array looks like: 34 15 74
The summary of each row: Sum of the elements of row 0 is: 7 Sum of the elements of row 1 is: 6 Sum of the elements of row 2 is: 11
Sample Output 2
Enter the number of rows in the array
2
Enter the number of columns in the array
2
Please enter a value for position (0, 0)
8
Please enter a value for position (0, 1)
23
Please enter a value for position (1, 0)
1
Please enter a value for position (1, 1)
9
Your 2D array looks like:
8 23 1 9
The summary of each row: Sum of the elements of row 0 is: 31 Sum of the elements of row 1 is: 10
Step by Step Solution
There are 3 Steps involved in it
Step: 1
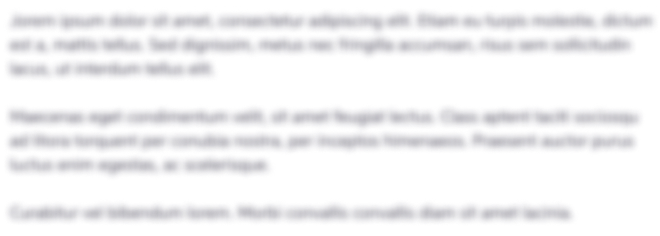
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started