Question
For this lab you will implement a dynamic array by completing the class called MyDynamicArray. The MyDynamicArray class should manage the storage of an array
For this lab you will implement a dynamic array by completing the class called MyDynamicArray. The MyDynamicArray class should manage the storage of an array that can grow and shrink.
The public methods of your class should be the following:
MyDynamicArray(); Default Constructor. The array should be of size 2.
MyDynamicArray(int s); For this constructor the array should be of size s.
~MyDynamicArray(); Destructor for the class.
int& operator[](int i); Traditional [] operator. Should print a message if i is out of bounds and return a reference to a zero value.
void add(int v); increases the size of the array by 1 and stores v there. Should double the capacity when the new element doesn't fit.
void del(); reduces the size of the array by 1. Should shrink the capacity when only 25% of the array is in use after the delete.
int length(); returns the length of the array.
int clear(); Frees any space currently used and starts over with an array of size 2.
you should also add a copy constructor and a copy assignment operator for the class.
MyDynamicArray& operator= (const MyDynamicArray& src)
MyDynamicArray(const MyDynamicArray& src)
You should complete the class by writing the add and del methods. When you need to grow the array size, the add method should print out the message Doubling to : followed by the new size of the array. When the del method shrinks the size of the array, print out the message Reducing to : followed by the new size of the array.
The output below should result from running the main.cpp file with your MyDynamicArray class:
Here is the given main.cpp:
#include
void foo(MyDynamicArray param) { for(int i=0; i int main() { MyDynamicArray x; for (int i=0; i This is also the code given to start MyDynamicArray.cpp : #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
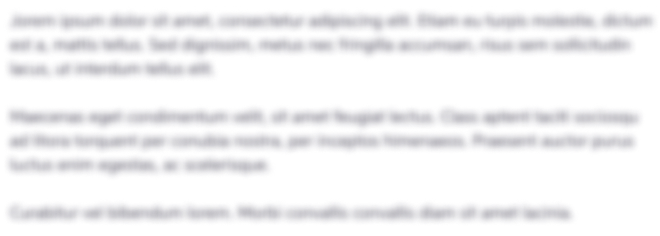
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started