Answered step by step
Verified Expert Solution
Question
1 Approved Answer
For this problem, write a class MyArray implemented as an instance class . This class will provide a series of instance ( i . e
For this problem, write a classMyArrayimplemented as aninstanceclass This class will provide a series ofinstanceienon static methods which will allow a client program to manipulate an array of integers.Effectively the classMyArraywill act as a custom data type ie Blueprint class which you can use to create objects, each one containing its own array that can be manipulated by calling the methods you will write.Begin by downloading the file:MyArrayjava, which setsup the skeleton of your class. As you can see the noargument constructor has been provided. This constructor creates the array of integers and assigns the address of the array to the data memberarr It also initializes the data membernumElementsto zero, as no elements have been added to the array.Important guidelines:The class membersmustnot be changed in any way. You must use the member names as provided or specified.Your methodsmusthave the exact headers that we have specified, or we wont be able to test them.Note in particular that the case of the letters matters.If you are asked to write a method that returns a value, make sure that itreturnsthe specified value, rather than printing itYou shouldnotuse any Java classes that we have not covered in the lecture notes.Make sure to do proper validation where necessary. For example, if an expected argument to a method should be positive do not allow for a negative number, and thrown an exception as needed.Each of your methods should be preceded by a comment that explainswhat your methods doesandwhat its inputs are. You should also include a comment at the top of the file.More generally, use good programming style. Use appropriate indentation, select descriptive variable names, insert blank lines between logical parts of your program, and add comments as necessary to explain what your code does. See thecoding conventionsfor more detail.Add the following data members as instance variables of the class:sum, represents thesumof all the elements in the arraymin, represents theminimumvalue of all elements in the arraymax, represents themaximumvalue of all the elements in the arrayavg, represents theaverageof all the elements in the arraymed, represents themedianvalue of all the elements in the arrayThese data members should be appropriately initialized in the constructors.Implement the following methods:Write a custom constructor that creates the array based on an argument to indicate the size of the array. This construcor should create an array of integers based on the input argument passed tonWrite a custom constructor that creates the array based on the array of integers passed to the method. This constructor should create an array of integers and initialize it from the array passed to the method.This constructor should initialize all the statistics in the object.Hint: This constructor shouldcreatea new array that is an exact copy of the array passed.Write a method that prompts the user to enter elements into the array of the object the method is called on The user may enter up to as many values as the array can holdor less. This method can use thesentinelmember of the class to determine end of input.A sample run of this method is:This assumes that the array can holdelements Calling thetoStringmethod on the object after user input would display the following array of elements:If the user enters more thanelements the remaining elements should not be accepted. You can assume that each time this method is called, it will override any elements currently in the array. However, it is important that the integrity of the object is maintained, therefore, this method must ensure thatallthe data members of the object are consistent with the elements in the array.Hint: This implies that the number of elements in the array does not necessarily have to be the same as the size of the array. Think of the purpose of the data memebernumElementsWrite a method thatcreatesandreturnsa String representing the contents of the array.Write a method that computes certain statistics of the array, specifically theminimummaximumsumaverageandmedianof all the elements contained in the array. These statistics should be stored internally in the object in the corresponding data members.Write a method that accepts a number and computes and returns how many times if any that number appears as an element in the array.Write a method that allows you toinserta specified number at a specified position within thecurrently filled portionof the array.In order to accomplish this, the method must first check to see ifpositionis betweenandnumElements The method must also check to see if there is room in the array to accept a new number.The method should returntrueif the number was inserted in the array. If the position specified is not within the filled range or if the array is currently full eg number of elements equals the size of the array then the method should returnfalseHint: Think carefully about what an insert into the array implies. The physical structure of the array cannot change, therefore what must happen if a value is to be inserted somewhere in the middle of the array without losing any of the existing elements.Write a method that removes an element at a specified position in the array.As with the insert function, the position specified must be within the filled bounds of the array. Again, the physical structure of the array cannot change, therefore we muatsimulatean element of the array being removed. Think how this can be accomplished.The method should returntrueif the element could be removed from the array orfalseif the position specified is not within bounds of the filled portion of the array.Write a method togrowthe array by some additionalsizeThis method shouldgrowthe size of the array of the object this method is called on bynpositions This method must also preserve all the elements that are currently in the array. In other words,growingthe array cannot lose any elements that are currently stored in the array.Hint: Again, the physical structure of the array cannot change, therefore we mustsimulategrowing the array. Think how this can be accomplished.Write accessor methods for each of the private data members.Hint:Gettermethods return the value of the data member they areaccessing Think what the return type for each method should be
Step by Step Solution
There are 3 Steps involved in it
Step: 1
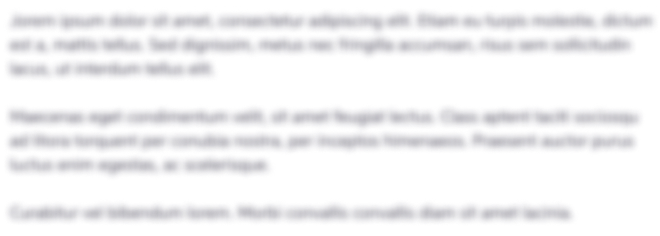
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started