Question
For this project, assume that sales amounts for an employee are saved to a file. We would like to know the average, number sold, total
For this project, assume that sales amounts for an employee are saved to a file. We would like to know the average, number sold, total sales, and maximum sale for an employee. Furthermore, we would like to know this information for all employees.
You will construct a Java program that
runs continuously (until user selects Exit option)
validates all user input
utilizes method overloading to calculate averages, maximums, counts, and totals for a specific employee id or all employees
Creates a text report of all information
For this project, you shall design a single class which contains all the necessary data and operations. A suggested class name is SalesAnalysisCalculator. You must decide upon the necessary import(s). The SalesAnalysisCalculator class contains
-Global variables: DecimalFormat object, File object.
-the main method
-Methods for calculations The main method should carry out the following tasks in the order given:
-Ask the user for the file name.
-Check if the file exists. If the file does not exist, then display an error message and the user makes an additional attempt.
- Display menu choices to the user.
- Menu choice is shown in picture below.
-Validate menu choice.
-Use an appropriate decision structure for the menu choice.
-For the employee choice, check if there is an employee with the entered id number. If not, then display an error message. Otherwise display the information.
-For all employees, display information as listed below based off of the information in the excel file (information is listed at the bottom).
-For create report, ask the user for the name of the file. Generate a text file that includes the average for all employees, number of sales, and total for all employees.
-For change file choice, implement similar to the beginning of the main method.
-For exit choice, exit the program.
Implement the following methods:
calculateAverage( ) - The return type is double. Takes a String parameter for an employee id. The method throws FileNotFound exception. Reads the file. Returns the average sales amount for entries with the id parameter.
calculateAverage( ) - The return type is double. The method throws FileNotFound exception. Reads the file. Returns the average sold value for all entries.
calculateTotal( ) - The return type is double. Takes a String parameter for an employee id. The method throws FileNotFound exception. Reads the file. Returns the total sales amount for entries with the id parameter.
calculateTotal( ) - The return type is double. The method throws FileNotFound exception. Reads the file. Returns the average total of entries.
calculateMax( ) - The return type is double. Takes a String parameter for an employee id. The method throws FileNotFound exception. Reads the file. Returns the max value for entries with the id parameter.
containsEmployee() - The return type is boolean. Takes a String parameter for an employee id. The method throws FileNotFound exception. Reads the file. Checks if the id parameter is contained in the file. Returns true if the id parameter is in the file; otherwise returns false.
calculateMax( ) - The return type is double. The method throws FileNotFound exception. Reads the file. Returns the max value for all entries.
calculateNumberSold( ) - The return type is int. Takes a String parameter for an employee id. The method throws FileNotFound exception. Reads the file. Returns the count of entries with the id parameter.
calculateNumberSold ( ) - The return type is int. The method throws FileNotFound exception. Reads the file. Returns the count of all entries.
createReport( ) - The return type is void. Takes a String parameter for a filename and a File parameter. The method throws FileNotFound exception. The method creates a file using the filename parameter. Use the above methods to print the analysis information. Refer to runnable for format.
The following information is located in an excel file named "data.csv". First number is employee id and second number are the sales amount. Both the Employee ID number and the Sales amount are listed in column A in the excel spreadsheet.
21-4500 211.71 |
10-0110 864.82 |
10-0110 951.86 |
31-2478 589.01 |
31-2478 325.70 |
31-2478 340.42 |
10-0110 409.66 |
10-0110 551.24 |
10-0110 173.46 |
21-4500 769.05 |
21-4500 586.86 |
10-0110 382.66 |
10-0110 919.90 |
21-4500 880.86 |
10-0110 781.47 |
31-2478 595.61 |
10-0110 949.67 |
31-2478 814.16 |
21-4500 639.28 |
21-4500 620.41 |
31-2478 467.93 |
31-2478 150.91 |
10-0110 684.22 |
10-0110 105.18 |
31-2478 313.92 |
10-0110 168.76 |
21-4500 631.38 |
31-2478 478.13 |
10-0110 454.71 |
21-4500 170.64 |
21-4500 978.51 |
31-2478 840.57 |
10-0110 724.80 |
21-4500 656.66 |
21-4500 563.56 |
10-0110 406.75 |
31-2478 358.49 |
21-4500 905.98 |
31-2478 416.32 |
10-0110 634.76 |
21-4500 709.38 |
10-0110 291.81 |
31-2478 818.65 |
31-2478 782.63 |
31-2478 330.42 |
21-4500 146.74 |
31-2478 444.91 |
31-2478 736.68 |
21-4500 718.63 |
21-4500 902.10 |
21-4500 531.18 |
31-2478 325.51 |
10-0110 649.64 |
10-0110 844.22 |
10-0110 474.06 |
10-0110 686.26 |
31-2478 581.69 |
10-0110 768.15 |
10-0110 387.45 |
21-4500 958.59 |
10-0110 754.35 |
21-4500 305.45 |
10-0110 931.03 |
21-4500 714.60 |
10-0110 888.20 |
10-0110 953.97 |
31-2478 338.54 |
31-2478 307.65 |
31-2478 581.29 |
21-4500 433.32 |
21-4500 527.68 |
21-4500 440.13 |
10-0110 902.46 |
31-2478 474.37 |
21-4500 360.27 |
10-0110 737.68 |
21-4500 978.92 |
31-2478 427.75 |
21-4500 441.00 |
10-0110 780.64 |
21-4500 414.35 |
31-2478 672.90 |
10-0110 228.78 |
21-4500 458.84 |
31-2478 860.88 |
21-4500 747.08 |
21-4500 463.05 |
10-0110 501.28 |
10-0110 726.40 |
31-2478 756.68 |
10-0110 168.33 |
31-2478 532.78 |
21-4500 347.38 |
21-4500 228.91 |
21-4500 317.60 |
21-4500 735.61 |
21-4500 259.22 |
10-0110 329.91 |
21-4500 673.48 |
21-4500 207.83 |
21-4500 641.82 |
10-0110 921.71 |
31-2478 218.26 |
21-4500 127.69 |
31-2478 889.45 |
21-4500 874.19 |
31-2478 994.94 |
21-4500 202.42 |
10-0110 152.56 |
21-4500 886.17 |
10-0110 417.98 |
31-2478 685.75 |
10-0110 248.22 |
31-2478 233.66 |
31-2478 226.82 |
10-0110 124.53 |
21-4500 494.38 |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
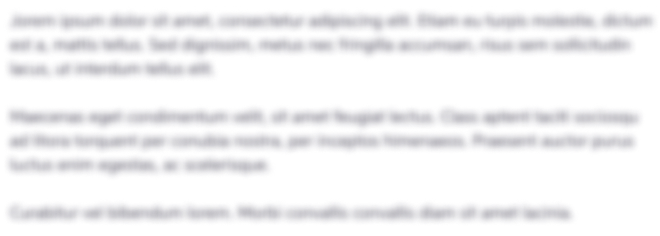
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started