Question
For this project we will explore the challenges associated with providing reliable delivery services over an unreliable network. Three different ARQ approaches have been described
For this project we will explore the challenges associated with providing reliable delivery services over an unreliable network. Three different ARQ approaches have been described in class: stop-and-wait, concurrent logical channels and sliding window. Your task during this project is to develop a set of Java classes capable of reliably transferring a file between two hosts over UDP (TCP already offers reliable delivery services).
Students will be provided with a UDPSocket class that extends the DatagramSocket class provided by Java to send a DatagramPacket over UDP. Take time to familiarize yourself with the main two classes provided by Java for UDP support and their functionality. The UDPSocket class has the additional capability of allowing programmers to modify the MTU (to model fragmentation), the packet drop rate (to model unreliable networks) and add random time delays to packets (to model out-of-order reception).
Support material
Parameters regulating message transfers using the UDPSocket class are located in a file named unet.properties. This file may be modified to test different scenarios (the file includes comments describing each parameter). Note that you can also use this file to enable and configure logging options (to file or standard output stream).
Unet.properties
#~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ # unet properties file #~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ #== log file name ================================ # # log.filename = system (standard output stream) log.filename = system log.enable = true #== packet drop rate ============================= # # n<0 --- drop random packets (n*-100) probability # n do not any all>0 --- Drop every nth packet (assuming no delay) # n=[d,]*d --- Drop select packets e.g. packet.droprate = 4,6,7 packet.droprate = 0 #== packet delay rate ============================ # # packet delay in milliseconds # min==max --- in order delivery packet.delay.minimum = 10 packet.delay.maximum = 100 #== packet maximum transmission size ============= # # -1 unlimited (Integer.MAX_VALUE) packet.mtu = 1500
A brief tutorial on how to use UDP in Java is provided in the Appendix. A zip file (unet.zip) containing sample code, a supporting jar file (unet.jar) as well as the default unet.properties file will be provided. Please note that Java 1.5 or better is required by UDPSocket.
Programs
A minimum of two classes will have to be submitted, RSendUDP.java and RReceiveUDP where each class must implement the edu.utulsa.unet.RSendUDPI and edu.utulsa.unet.RReceiveUDPI interfaces respectively (as specified below, provided in unet.jar).
package edu.utulsa.unet; import java.net.InetSocketAddress; public interface RSendUDPI { public boolean setMode(int mode); public int getMode(); public boolean setModeParameter(long n); public long getModeParameter(); public void setFilename(String fname); public String getFilename(); public boolean setTimeout(long timeout); public long getTimeout(); public boolean setLocalPort(int port); public int getLocalPort(); public boolean setReceiver(InetSocketAddress receiver); public InetSocketAddress getReceiver(); public boolean sendFile();
}
package edu.utulsa.unet; public interface RReceiveUDPI { public boolean setMode(int mode); public int getMode(); public boolean setModeParameter(long n); public long getModeParameter(); public void setFilename(String fname); public String getFilename(); public boolean setLocalPort(int port); public int getLocalPort(); public boolean receiveFile(); }
The setMode method specifies the algorithm used for reliable delivery where mode is 0 or 1 (to specify stop-and-wait and sliding window respectively). If the method is not called, the mode should default to stop-and-wait. Its companion, getMode simply returns an int indicating the mode of operation.
The setModeParameter method is used to indicate the size of the window in sliding window mode. A call to this method when using stop-and-wait should have no effect. The default value should be 256 for the sliding window algorithm.
The setFilename method is used to indicate the name of the file that should be sent (when used by the sender) or the name to give to a received file (when used by the receiver).
The setTimeout method specifies the timeout value in milliseconds. Its default value should be one second.
A sender uses setReceiver to specify IP address (or fully qualified name) of the receiver and the remote port number. Similarly, setLocalPort is used to indicate the local port number used by the host. The sender will send data to the specified IP and port number. The default local port number is 12987 and if an IP address is not specified then the local host is used.
The methods sendFile and receiveFile initiate file transmission and reception respectively. Methods returning a boolean should return true if the operation succeeded and false otherwise.
Operation requirements
RReceiveUDP
Should print an initial message indicating the local IP, the ARQ algorithm (indicating the value of n if appropriate) in use and the UDP port where the connection is expected.
Upon successful initial connection from a sender, a line should be printed indicating IP address and port used by the sender.
For each received message print its sequence number and number of data bytes
For each ACK sent to the sender, print a message indicating which sequence number is being acknowledged
Upon successful file reception, print a line indicating how many messages/bytes were received and how long it took.
RSendUDP
Should print an initial message indicating the local IP, the ARQ algorithm (indicating the value of n if appropriate) in use and the local source UDP port used by the sender.
Upon successful initial connection, a line should be printed indicating address and port used by the receiver.
For each sent message print the message sequence number and number of data bytes in the message
For each received ACK print a message indicating which sequence number is being acknowledged
If a timeout occurs, i.e. an ACK has been delayed or lost, and a message needs to be resent, print a message indicating this condition
Upon successful file transmission, print a line indicating how many bytes were sent and how long it took.
Fragmentation and in-order-delivery
In-order delivery is implicitly solved by the stop-and-wait algorithm (only one outstanding packet at all times) and explicitly by the sliding-window algorithm. However, the concurrent logical channels approach, while reliable, does not provide in order delivery. You are responsible for developing an operating solution that will allow your programs to reconstruct the file even if fragments were received out-of-order.
Design
Your programs are essentially operating at the application layer (just like ftp, http and many other protocols) using an unreliable transport layer (UDP). Please plan your design before you start coding and define a packet format that will allow safe and consistent file transfers.
I encourage group discussions and sharing of the packet format used for the file transfers. Implementation should be individual. The advantage of sharing the packet format with other students is that you will have the ability of testing your program with others.
Usage
Program usage will be illustrated by the following example. Assume Host A has IP address 192.168.1.23 and Host B has IP address 172.17.34.56 (note that none of these IP addresses are routable over the Internet). Host A wants to send a file named important.txt to Host B. Host A wants to use local port 23456 and Host B wants to use local port 32456 during the file transfer. Host B does not consider the file so important and it wants to save the received file as less_important.txt. Both Host A and B have agreed to use the sliding-window protocol with a window size of 512 bytes and Host A will use a timeout value of 10 seconds (the path between A and B has a rather large delay).
Sample Code
The following code running on Host A should accomplish the task of reliably sending the file:
RSendUDP sender = new RSendUDP(); sender.setMode(2); sender.setModeParameter(512); sender.setTimeout(10000); sender.setFilename(important.txt); sender.setLocalPort(23456) sender.setReceiver(new InetSocketAddress(172.17.34.56, 32456) sender.sendFile();
The following code running on Host B should accomplish the task of receiving the file:
RReceiveUDP receiver = new RReceiveUDP(); receiver.setMode(2); receiver.setModeParameter(512); receiver.setFilename(less_important.txt); receiver.setLocalPort(32456) receiver.receiveFile();
Sample Output
Assume our solution uses 10 bytes of header for every message, the network cannot deliver messages larger than 200 bytes and the file we are transferring is 800 bytes long. The output of your program (on the sender side) when running the stop-and-wait algorithm could look like this:
Sending important.txt from 192.168.1.23:23456 to 172.17.34.56:32456 with 800 bytes Using stop-and-wait Message 1 sent with 190 bytes of actual data Message 1 acknowledged Message 2 sent with 190 bytes of actual data Message 2 acknowledged Message 3 sent with 190 bytes of actual data Message 3 timed-out Message 3 sent with 190 bytes of actual data Message 3 acknowledged Message 4 sent with 190 bytes of actual data Message 4 acknowledged Message 5 sent with 40 bytes of actual data Message 5 acknowledged Successfully transferred important.txt (800 bytes) in 1.2 seconds
A similar output should be seen on the receiver side.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
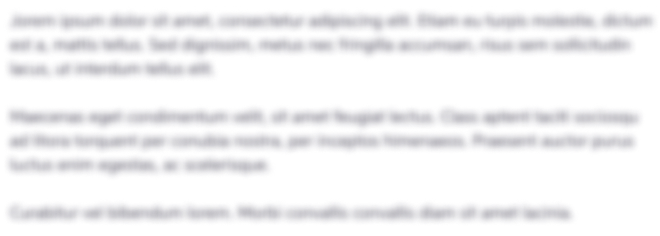
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started