Question
For this project, you shall define a new class BankDatabase . The BankDatabase contains the bank account information for all accounts including saving accounts and
For this project, you shall define a new class BankDatabase. The BankDatabase contains the bank account information for all accounts including saving accounts and checking accounts. The customer names are passed as firstname lastname. Your application must split the string into two separate strings for first name and last name (Hint: use the split() method). If you decide to use an array to store the bank accounts, you can assume there will be maximum of 100 bank accounts in the Bank Database. Alternatively, you can consider using an ArrayList in which case its size can grow automatically as new bank accounts are added. BankDatabase class implements the following methods:
BankDatabase() constructor
void creatCheckingAccount(String customerName, String ssn, float deposit) This method creates a checking account
void creatSavingAccount(String customerName, String ssn, float deposit) This method creates a saving account
void print() This method prints the bank account information in the database in ascending order of the account balances.
void applyIntrest This methods applies interest to all bank accounts. The interest for each type of account is the same as project 5.
Make the following updates to the BankAccount super class:
1) Make the BankAccount super class an abstract class and make applyInterest an abstract method.
2) The BankAccount super class shall implement the Comparable Interface. You shall implement the compareTo method to provide the means to sort the bank accounts in the ascending order of the account balance.
Identify the objects for this application and define each class attribute with proper access qualifier. You can use the main method shown below to test your application. The expected output is also provided.
public class BankApp {
public static void main(String[] args) {
BankDatabase acctDatabase = new BankDatabase();
acctDatabase.createCheckingAccount("Alin Parker", "123-45-6789", 20000.0f);
acctDatabase.createSavingAccount("Mary Jones", "987-65-4321", 15000.0f);
acctDatabase.createSavingAccount("John Smith", "123-45-6789", 12000.0f);
acctDatabase.print();
acctDatabase.applyInterest();
acctDatabase.print();
}
======= expected output =============
Succesfully created account for Alin Parker Account Number 5435736518
Succesfully created account for Mary Jones Account Number 3612979581
Succesfully created account for John Smith Account Number 6998050883
John Smith, accn #: 6998050883, Balance $12000.0
Mary Jones, accn #: 3612979581, Balance $15000.0
Alin Parker, accn #: 5435736518, Balance $20000.0
John Smith, accn #: 6998050883, Balance $12600.0
Mary Jones, accn #: 3612979581, Balance $15750.0
Alin Parker, accn #: 5435736518, Balance $20200.0
java code from previous week & the code to build upon:
//create the customer class with variables and methods */ class Customer { //declare the variables with private private String first_Name; //declare the variables with private private String last_Name; //declare the variables with private private String SSN; //declare the variables with private private Boolean valid_SSN; //constructor for customer public Customer(String first_Name, String last_Name, String SSN) { this.first_Name = first_Name; this.last_Name = last_Name; this.SSN = SSN; this.checkIsSSN_Valid(); /*method to verify SSN is valid or not by splitting the input string and parsing to Integer to check range*/ } /*method to verify SSN is valid or not by splitting the input string and parsing to Integer to check range*/ private void checkIsSSN_Valid() { String[] arrOfStr = this.SSN.split("-", 5); int number = Integer.parseInt(arrOfStr[0]); if (number >= 100 && number <= 999) { number = Integer.parseInt(arrOfStr[1]); if (number >= 10 && number <= 99) { number = Integer.parseInt(arrOfStr[2]); if (number >= 1000 && number <= 9999) { this.valid_SSN = true; } } else { this.valid_SSN = false; } } else { this.valid_SSN = false; } } //getters public String getFirstName() { return this.first_Name; } //getters public String getLastName() { return this.last_Name; } //getters public boolean getValidSSN() { return this.valid_SSN; } } //create BankAccount which extends customer class BankAccount extends Customer { //declare the variables with private private long accountNumber; //declare the variables with private private float balance; //create constructor public BankAccount(String first_Name, String last_Name, String SSN, Float balance) { super(first_Name, last_Name, SSN); this.balance = balance; this.generateAccountNumber(); this.displayAccount(); } // this method generate the random account number private void generateAccountNumber() { this.accountNumber = (long) Math.floor(Math.random() * 9_000_000_000L) + 1_000_000_000L; } //method displayAccount account public void displayAccount() { String status = "Successfully created account for " + super.getFirstName() + " " + super.getLastName() + " Account Number " + this.accountNumber; String valid = ""; //check for gervalidSSN if (!super.getValidSSN()) { valid = "Successfully created account for " + super.getFirstName() + " " + super.getLastName() + " Invalid SSN!"; System.out.println(valid); } String balance = this.getFirstName() + " " + this.getLastName() + " ,Balance $" + this.balance; System.out.println(status); System.out.println(balance); } // to deposit in account public void deposit(float amount) { this.balance = this.balance + amount; String message = this.getFirstName() + " " + this.getLastName() + " deposited $" + amount + " .Current balance " + this.balance; System.out.println(message); } // to withdraw amount public void withdraw(float amount) { if (amount > this.balance) { String message = "Unable to withdraw " + amount + " for " + super.getFirstName() + " " + super.getLastName() + " due to insufficient funds "; System.out.println(message); } else { this.balance = this.balance - amount; String message = this.getFirstName() + " " + this.getLastName() + " withdrew $" + amount + " .Current balance " + this.balance; System.out.println(message); } } //method declaration public void checkBalance() { String message = this.getFirstName() + " " + this.getLastName() + " , Balance $" + this.balance; System.out.println(message); } public void applyInterest() { // find amount exceeding 10,000 float amount = (float) (this.balance - 10000.0); this.balance = (float) (this.balance +((amount *2.0)/100)); } //setter method public void setBalance(float balance) { this.balance = this.balance + balance; } //getters methods public float getBalance() { return this.balance; } } class CheckingAccount extends BankAccount { CheckingAccount(String first_Name, String last_Name, String SSN, float balance) { super(first_Name, last_Name, SSN, balance); } } class SavingAccount extends BankAccount { SavingAccount(String first_Name, String lastname, String SSN, float balance) { super(first_Name, lastname, SSN, balance); } // override interest method for Saving account public void applyInterest() { float interest = (float) ((this.getBalance() * 5.0)/100); this.setBalance(interest); } } public class BankMain { public static void main(String[] args) { CheckingAccount acct1 = new CheckingAccount("Alin", "Parker", "123-45-6789", 1000.0f); CheckingAccount acct2 = new CheckingAccount("Mary", "Jones", "987-65-4321", 500.0f); SavingAccount acct3 = new SavingAccount("John", "Smith", "1233-45-6789", 200.0f); acct1.deposit(22000.00f); acct2.deposit(12000.00f); acct1.withdraw(2000.00f); acct2.withdraw(1000.00f); acct1.applyInterest(); acct2.applyInterest(); acct1.checkBalance(); acct2.checkBalance(); acct1.withdraw(30000.00f); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
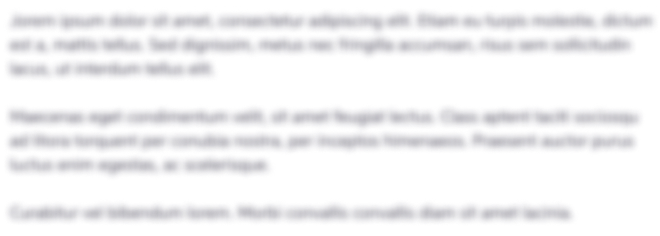
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started