Question
For this question, you will write several classes, and then create and use instances of those classes in order to code part of the card
For this question, you will write several classes, and then create and use instances of those classes in order to code part of the card game Bridge. Bridge is a 4 players game that uses a standard deck of 52 cards. At the beginning, all the cards are dealt to the players, and each player receives 13 cards. The game of Bridge has two main parts: the Bidding and the Play. In the bidding phase, a player must first evaluate ones hand of card in order to figure out how good the hand is. To do this, players use a basic point-count system which is a combination of different points. In this assignment, you will do the following:
Write a class to define a new type Card which is meant to represent a card in a game such as bridge, poker, or blackjack. Write a class to define a new type Deck which is meant to represent a deck of 52 cards. Write a BridgeUtilities class to store a set of methods needed to implement a basic point-count system for the game Bridge. Write a Game class where youll be coding the first part of the Bidding phase in a game of Bridge. Note: In addition to the required methods listed below, you are free to add as many other private methods as you want (no additional public method is allowed). It is up to you to figure out whether a method should be static or not based on its description.
(a) Write a class Card. A Card has the following private attributes: A String suit. An int value.
There are four possible options for suits: hearts, spades, clubs, and diamonds. There are 13 possible options for the value: ace, two, three, four, five, six, seven, eight, nine, ten, jack, queen, and king.
For this assignment, we will represent the value using an int which has a value between 1 and 13. The suit will be represented using a String. The Card class also contains the following public methods: A constructor that takes as input an int for the value and a String for the suit, and initializes the attributes accordingly. The inputs are considered to be valid if they create a card of value between 1 and 13 (both included) and of suit equal to either hearts, spades, clubs, or diamonds (please ignore capitalization). If the input are invalid, then the constructor should throw an IllegalArgumentException explaining that no card of such type can be created. getSuit() which returns the suit of the card. getValue() which returns the value of the card. print() which takes nothing as input and prints the content of the card. For example 3 of spades or 13 of clubs. Note that it is not necessary to print the words such as king or queen. You can leave them as 13 or 12 if you want to.
(b) (18 points) Write a class Deck. A Deck has the following private attribute: An array of Cards. The Deck class also contains the following public methods: A constructor that takes nothing as input and initializes the attribute with a Card[] of size 52. The constructor should also initialize such array with all 52 possible cards. To get full marks you must use at least 1 loop to accomplish this (that is, you cannot write 52 total statements to assign all possible values). Hint: Create a String array of size 4 with the suit values, and then use nested for loops to initialize the cards. shuffle() which takes no input and returns no value. This method should shuffle the array of Cards of this Deck. To do so, do the following: create an object of type Random (remember that to use Random you should add the appropriate import statement at the beginning of your file). To make your program easier to debug (and grade), you must provide a seed for the Random object. Use a seed equal to 123. Then create a loop that iterates 1000 times. Inside the loop, generate 2 random integers between 0 and 51 (both included) and swap the values at those positions in the array.
For example, suppose the array of Cards of this Deck is the following: [AH, 2H, 3H, 4H, 5H, 6H, 7H, 8H, 9H, 10H, JH, QH, KH, AS, 2S, 3S, 4S, 5S, 6S, 7S, 8S, 9S, 10S, JS, QS, KS, AC, 2C, 3C, 4C, 5C, 6C, 7C, 8C, 9C, 10C, JC, QC, KC, AD, 2D, 3D, 4D, 5D, 6D, 7D, 8D, 9D, 10D, JD, QD, KD] Where the first symbol denotes the value of the card and the second the suit (C stands for clubs, H for hearts, D for diamonds, and S for spades). Then, after the method shuffle() is appropriately called on such Deck, the array of Cards will look as follows: [AD, 6D, AS, QH, 7H, 5D, 5S, KS, 9D, AH, 4D, 6H, 4H, 2H, 9S, 10D, JH, JD, KD, 8H, 3C, 7S, 10C, 8S, JC, 10H, QS, 4C, 3H, 9H, 7D, 8C, KH, JS, 3S, 7C, 6C, 3D, 9C, 6S, QC, 10S, 5H, 5C, 4S, 2D, KC, 8D, 2S, 2C, QD, AC]
dealHand() which takes as input two integers. The first one, call it n, indicates how many cards should be dealt, the second one indicates the players number. The idea is that the first player should be dealt the first n cards in this Deck, the second player the next n cards, and so on. The method should return an array of Cards representing the hand dealt to the player. If there are not enough cards to deal (for instance we cannot deal a hand of 12 cards to a fifth player, since the first four players already took 48 cards, and only 4 cards are left in the deck), then the method should throw an IllegalArgumentException explaining that there are not enough cards in the deck.
(c) (20 points) Write a class BridgeUtilities. This class is not meant to represent a new data type. You will write this class simply to group together methods that are needed to implement a basic point-count system for the game Bridge. As such, this class has no attributes. To evaluate the points associated to a hand of cards, we will be using a combination of high card points (HCP) and distributional points, as follows. High Card Points. The top four cards in each suit are given point values relative to their rank as follows: Every Ace is worth 4 points. Every King is worth 3 points. Every Queen is worth 2 points. Every Jack is worth 1 point. All other cards are worth 0. Remember that the values associated to an ace, king, queen, and jack are equal to 1, 13, 12, and 11 respectively. Distributional Points. In addition to HCP, another factor that determines the strength of the a bridge hand is the length of each suit. Since there are 13 cards and 4 suits, a balanced hand would have 4 cards in one suit and 3 cards in the other three suits. In bridge, a balanced hand is a very weak hand. Thus, if the hand is unbalanced it is considered to be good. For this reason, the hand is worth 1 extra point for every card more than 4 in each suit. For example, if the hand has 5 cards of the same suit one extra point is given, if it has 6 cards of the same suit 2 extra points are given, if it has 7 cards of the same suit 3 extra points are given, and so on. For example, suppose you were dealt the following hand: 1C, 2C, JC, KC, 9C, 3H, 4H, 5H, 6H, 7H, KH, 1D, 1S Then, the evaluation of your hand would go as follows High Cards Points 3 aces (1C, 1D, 1S) = 3 * 4 = 12 points 2 kings (KC, KH) = 2 * 3 = 6 points 0 queens = 0 points 1 jack (JC) = 1 * 1 = 1 point Distributional Points 5 clubs = 1 more than 4 = 1 point 6 hearts = 2 more than 4 = 2 points 1 diamonds = less than or equal to 4 = 0 points 1 spades = less than or equal to 4 = 0 points For a total of 12 + 6 + 1 + 1 + 2 = 22 points. Inside the BridgeUtilities class write the following methods: A private constructor that takes no inputs and does nothing. By adding this constructor we make sure that no objects of type BridgeUtilities can be created from outside this class. A private method countValue that takes as input a Card[] and an int representing a specific card value. The method should return how many Cards in the array have the specified value. A private method countSuit that takes as input a Card[] and a String representing a specific card suit. The method should return how many Cards in the array have the specified suit. Note: make sure to ignore capitalization. A public method countPoints that takes as input a Card[] and returns the number of points that the hand is worth. The method should compute the points using the schema described above: HCP + Distributional points. To get full marks, you need to use the countValue and countSuit methods.
(d) (10 points) Write a class Game. This class is where the main method should go. Inside the main method do the following: 1. Create a Deck. 2. Shuffle the Deck. 3. Deal four hands of Cards (one per player). Each hand should contain 13 cards. 4. Compute how many points each hand is worth. 5. For each player, display the cards inside their hand and how many points their hand is worth.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
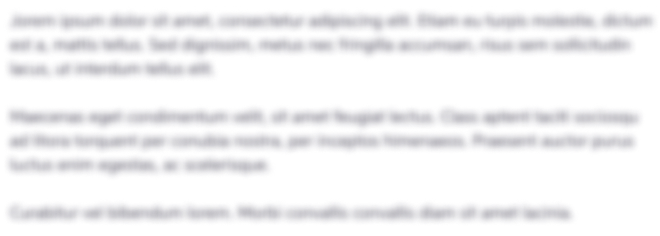
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started