Answered step by step
Verified Expert Solution
Question
1 Approved Answer
FORK_BASIC.C #include #include #include /* getpid() and fork() are system calls declared in unistd.h. They return */ /* values of type pid_t. This pid_t is
FORK_BASIC.C #include #include #include /* getpid() and fork() are system calls declared in unistd.h. They return */ /* values of type pid_t. This pid_t is a special type for process ids. */ /* It's equivalent to int. */ int main(void) { pid_t pid; /* fork a child process */ pid = fork(); if (pid fprintf (stderr, "Fork failed "); return 1; } else if (pid == 0) { /* child process */ printf ("I am the child process and my fork() call returned %d ", pid); } else { /* parent process */ printf ("I am the parent process and my fork() call returned %d ", pid); } return 1; } FORK_WAIT.C #include #include #include #include #include /* pid_t fork(void) is declared in unistd.h */ /* pid_t is a special type for process ids. it's equivalent to int. */ int main(void) { pid_t child_pid; int status; pid_t wait_result; child_pid = fork(); if (child_pid == 0) { /* this code is only executed in the child process */ printf ("I am a child and my pid = %d ", getpid()); printf ("My parent is %d ", getppid()); /* an exit here would stop only the child process */ } else if (child_pid > 0) { /* this code is only executed in the parent process */ printf ("I am the parent and my pid = %d ", getpid()); printf ("My child has pid = %d ", child_pid); } else { printf ("The fork system call failed to create a new process " ); exit(1); } /* this code is executed by both parent and child processes */ printf ("I am a happy, healthy process and my pid = %d ", getpid()); if (child_pid == 0) { /* this code is only executed by the child process */ printf ("I am a child and I am quitting work now! "); } else { /* this code is only executed by the parent process */ printf ("I am a parent and I am going to wait for my child "); do { /* parent waits for the SIGCHLD signal sent to the parent of a (child) process when the child process terminates */ wait_result = wait(&status); } while (wait_result != child_pid); printf ("I am a parent and I am quitting. "); } return 0; } SIMPLE_FORK1.C #include #include int main() { printf ("0. I am process %d ", getpid()); (void) fork(); printf ("1. I am process %d ", getpid()); (void) fork(); printf ("2. I am process %d ", getpid()); } SIMPLE_FORK2.C #include #include int main() { printf ("0. I am process %d ", getpid()); (void) fork(); printf ("1. I am process %d ", getpid()); (void) fork(); printf ("2. I am process %d ", getpid()); (void) fork(); printf ("3. I am process %d ", getpid()); } SIMPLE_FORK3.C #include #include int main() { printf ("0. I am process %d ", getpid()); (void) fork(); printf ("1. I am process %d ", getpid()); (void) fork(); printf ("2. I am process %d ", getpid()); (void) fork(); printf ("3. I am process %d ", getpid()); (void) fork(); printf ("4. I am process %d ", getpid()); } Prince Sultan University 182 CS330: Operating Systems Part one-C Programming (0.25) Write a program in Cthat prompts the user to enter a number, your program should then compute the square of that number (the number raised to the power of two) and prints it to the user Note: Square) should be implemented as a separate function and called in the main. Part two-Linux Commands (0.25) Perform the following tasks using Linux commands. You may use options commands when needed. Note: Please include your answer as a text below each point, and not a screenshot. 1. Create a directory named tmp in your current directory i.e. home directory. 2. Create a file myfile.txt in your home directory 3. Change the permissions for myfile.txt to be: RWX for Owner, Read only for Group, and none for Others 4. List the files in the directory 5. Make a copy of the file myfile.txt named newfile1.txt in the same directory 6. Make a copy of the file myfile.txt in the directory tmp and display a message that shows the copy was performed. 7. Move the two txt files from your current home directory into the tmp directory 8. Change your directory to tmp directory 9. Rename myfile.txt as newfile2.txt. 10. Delete all files in your current directory and ask the user for confirmation before deleting. 11. From your current directory which is tmp, go to the parent directory. 12. Delete the directory tmp. Prince Sultan University 182 CS330: Operating Systems Part three -Processes (0.5) Question 1: Fork() system call Look at the program FORK_BASIC.C. Compile and execute it. Fill the following table: Note: include a screenshot of your answer. Value returned by fork to the child process Process ID of the child Question 2: Wait() system call Suppose we want to have the parent wait for a signal from the child process. Look at the program FORK-WAIT.. Compile and execute it. Answer the following questions: Note: include a screenshot of your answer. What is the child process's id? What is the return value of the wait system call? Question 3: Multiple fork) calls 1. Look at the program SIMPLE_FORK1.C which contains two fork) call. Compile and execute it. How many processes are created by executing this programi Note: include a screenshot of your answer. 2. Look at the program SIMPLE FORK2.C which contains three fork)calls. Compile and execute it. How many processes are created by executing this program? Note: include a screenshot of your answer. Prince Sultan University 182 CS330: Operating Systems 3. Look at the program SIMPLE_FORK3.C which contains four fork() call. Compile and execute it. How many processes are created by executing this program? Note: include a screenshot of your answer. 4. Based on the above, how many processes are created by executing a program that contains n fork()calls? Part four-Processes Creation (0.5 Write a C program that creates 64 processes using fork() system calls. Have each process print out its process ID. . Note: include the code and a screenshot of your run



FORK_BASIC.C
#include
#include
#include
/* getpid() and fork() are system calls declared in unistd.h. They return */
/* values of type pid_t. This pid_t is a special type for process ids. */
/* It's equivalent to int. */
int main(void)
{
pid_t pid;
/* fork a child process */
pid = fork();
if (pid
fprintf (stderr, "Fork failed ");
return 1;
}
else if (pid == 0) { /* child process */
printf ("I am the child process and my fork() call returned %d ", pid);
}
else { /* parent process */
printf ("I am the parent process and my fork() call returned %d ", pid);
}
return 1;
}
FORK_WAIT.C
#include
#include
#include
#include
#include
/* pid_t fork(void) is declared in unistd.h */
/* pid_t is a special type for process ids. it's equivalent to int. */
int main(void) {
pid_t child_pid;
int status;
pid_t wait_result;
child_pid = fork();
if (child_pid == 0)
{
/* this code is only executed in the child process */
printf ("I am a child and my pid = %d ", getpid());
printf ("My parent is %d ", getppid());
/* an exit here would stop only the child process */
}
else if (child_pid > 0)
{
/* this code is only executed in the parent process */
printf ("I am the parent and my pid = %d ", getpid());
printf ("My child has pid = %d ", child_pid);
}
else
{
printf ("The fork system call failed to create a new process "
);
exit(1);
}
/* this code is executed by both parent and child processes */
printf ("I am a happy, healthy process and my pid = %d ", getpid());
if (child_pid == 0)
{
/* this code is only executed by the child process */
printf ("I am a child and I am quitting work now! ");
}
else
{
/* this code is only executed by the parent process */
printf ("I am a parent and I am going to wait for my child ");
do
{
/* parent waits for the SIGCHLD signal sent
to the parent of a (child) process when
the child process terminates */
wait_result = wait(&status);
} while (wait_result != child_pid);
printf ("I am a parent and I am quitting. ");
}
return 0;
}
SIMPLE_FORK1.C
#include
#include
int main()
{
printf ("0. I am process %d ", getpid());
(void) fork();
printf ("1. I am process %d ", getpid());
(void) fork();
printf ("2. I am process %d ", getpid());
}
SIMPLE_FORK2.C
#include
#include
int main()
{
printf ("0. I am process %d ", getpid());
(void) fork();
printf ("1. I am process %d ", getpid());
(void) fork();
printf ("2. I am process %d ", getpid());
(void) fork();
printf ("3. I am process %d ", getpid());
}
SIMPLE_FORK3.C
#include
#include
int main()
{
printf ("0. I am process %d ", getpid());
(void) fork();
printf ("1. I am process %d ", getpid());
(void) fork();
printf ("2. I am process %d ", getpid());
(void) fork();
printf ("3. I am process %d ", getpid());
(void) fork();
printf ("4. I am process %d ", getpid());
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
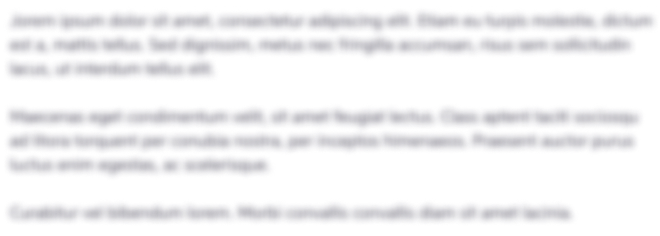
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started