Answered step by step
Verified Expert Solution
Question
1 Approved Answer
from business_layer import (Inventory, Menu, Order, RecipeManager, InventoryManager, MenuManager, OrderManager) from data_layer import DataLayer class PizzaStoreCLI: def __init__(self): # Initialize data and business layers self.data_layer
from business_layer import (Inventory, Menu, Order, RecipeManager, InventoryManager, MenuManager, OrderManager) from data_layer import DataLayer class PizzaStoreCLI: def __init__(self): # Initialize data and business layers self.data_layer = DataLayer() self.inventory = Inventory() self.menu = Menu() self.recipe_manager = RecipeManager(self.menu) self.inventory_manager = InventoryManager(self.inventory) self.menu_manager = MenuManager(self.menu) self.order_manager = OrderManager(self.data_layer, self.menu, self.inventory) def run(self): while True: self.display_main_menu() choice = input("Enter the number of your choice: ") if choice == '1': self.manage_recipes() elif choice == '2': self.manage_inventory() elif choice == '3': self.manage_menu() elif choice == '4': self.customize_pizza_order() elif choice == '5': self.manage_side_dishes() elif choice == '6': self.place_order() elif choice == '7': break else: print("Invalid choice. Please try again.") def manage_menu(self): while True: print(" Menu Management") print("1. Create New Pizza") print("2. Remove Pizza") print("3. Add New Side Dish") print("4. Remove Side Dish") print("5. Display Menu") print("6. Return to Main Menu") choice = input("Enter the number of your choice: ") if choice == '1': self.add_new_pizza_to_menu() elif choice == '2': self.remove_pizza_from_menu() elif choice == '3': self.add_new_side_dish_to_menu() elif choice == '4': self.remove_side_dish_from_menu() elif choice == '5': self.display_menu() elif choice == '6': break else: print("Invalid choice. Please try again.") def add_new_pizza_to_menu(self): print(" Adding a New Pizza to Menu") # Implement adding a new pizza to the menu pizza_name = input("Enter pizza name: ") pizza_description = input("Enter pizza description: ") pizza_ingredients = input("Enter pizza ingredients (comma-separated): ").split(', ') pizza_price = float(input("Enter pizza price: ")) pizza_type = input("Enter pizza type (e.g., vegetarian, meat lovers, specialty): ") # Implement the logic to add the pizza to the menu # Example: self.menu_manager.add_new_pizza(pizza_name, pizza_description, pizza_ingredients, pizza_price, pizza_type) print(f"Pizza '{pizza_name}' added to the menu.") Step 3/3 The final lines of code is as follows:- def remove_pizza_from_menu(self): print(" Removing a Pizza from Menu") # Implement removing a pizza from the menu pizza_name = input("Enter pizza name to remove: ") # Implement the logic to remove the pizza from the menu # Example: self.menu_manager.remove_pizza(pizza_name) print(f"Pizza '{pizza_name}' removed from the menu.") def add_new_side_dish_to_menu(self): print(" Adding a New Side Dish to Menu") # Implement adding a new side dish to the menu side_dish_name = input("Enter side dish name: ") side_dish_description = input("Enter side dish description: ") side_dish_price = float(input("Enter side dish price: ")) side_dish_type = input("Enter side dish type (e.g., appetizers, desserts, beverages): ") # Implement the logic to add the side dish to the menu # Example: self.menu_manager.add_new_side_dish(side_dish_name, side_dish_description, side_dish_price, side_dish_type) print(f"Side dish '{side_dish_name}' added to the menu.") def remove_side_dish_from_menu(self): print(" Removing a Side Dish from Menu") # Implement removing a side dish from the menu side_dish_name = input("Enter side dish name to remove: ") # Implement the logic to remove the side dish from the menu # Example: self.menu_manager.remove_side_dish(side_dish_name) print(f"Side dish '{side_dish_name}' removed from the menu.") def display_menu(self): print(" Displaying Menu") # Implement displaying the menu menu_items = self.menu_manager.get_menu_items() if not menu_items: print("The menu is empty.") else: print("Menu Items:") for item in menu_items: print(item) def manage_inventory(self): while True: print(" Ingredient Inventory Management") print("1. Maintain Inventory") print("2. Track Ingredient Usage") print("3. Generate Low-Stock Alerts") print("4. Return to Main Menu") choice = input("Enter the number of your choice: ") if choice == '1': self.maintain_inventory() elif choice == '2': self.track_ingredient_usage() elif choice == '3': self.generate_low_stock_alerts() elif choice == '4': break else: print("Invalid choice. Please try again.") def maintain_inventory(self): print(" Maintaining Ingredient Inventory") # Implement maintaining ingredient inventory ingredient_name = input("Enter ingredient name: ") ingredient_quantity = float(input("Enter current quantity: ")) # Implement the logic to maintain ingredient inventory # Example: self.inventory_manager.update_ingredient_quantity(ingredient_name, ingredient_quantity) print(f"Inventory for '{ingredient_name}' updated successfully.") def track_ingredient_usage(self): print(" Tracking Ingredient Usage") # Implement tracking ingredient usage recipe_name = input("Enter recipe name to track usage: ") # Implement the logic to track ingredient usage based on recipe requirements # Example: self.inventory_manager.track_ingredient_usage(recipe_name) print(f"Ingredient usage for '{recipe_name}' tracked successfully.") def generate_low_stock_alerts(self): print(" Generating Low-Stock Alerts") # Implement generating low-stock alerts low_stock_ingredients = self.inventory_manager.check_low_stock() if low_stock_ingredients: print("Low stock ingredients:") for ingredient in low_stock_ingredients: print(ingredient) else: print("No low stock ingredients.") def manage_recipes(self): while True: print(" Recipe Management") print("1. Create New Recipe") print("2. Edit Recipe") print("3. Delete Recipe") print("4. Search Recipes") print("5. Return to Main Menu") choice = input("Enter the number of your choice: ") if choice == '1': self.create_recipe() elif choice == '2': self.edit_recipe() elif choice == '3': self.delete_recipe() elif choice == '4': self.search_recipes() elif choice == '5': break else: print("Invalid choice. Please try again.") def create_recipe(self): print(" Creating a New Recipe") # Implement creating a new recipe pizza_name = input("Enter pizza name: ") pizza_type = input("Enter pizza type (e.g., vegetarian, meat lovers, specialty): ") ingredients = input("Enter ingredients (comma-separated): ").split(', ') quantities = input("Enter quantities (comma-separated): ").split(', ') # Implement the logic to create a new recipe # Example: self.recipe_manager.create_recipe(pizza_name, pizza_type, ingredients, quantities) print(f"Recipe for '{pizza_name}' created successfully.") def edit_recipe(self): print(" Editing a Recipe") # Implement editing a recipe pizza_name = input("Enter pizza name to edit: ") new_name = input("Enter new pizza name (press enter to keep the same): ") new_type = input("Enter new pizza type (press enter to keep the same): ") new_ingredients = input("Enter new ingredients (comma-separated, press enter to keep the same): ").split(', ') new_quantities = input("Enter new quantities (comma-separated, press enter to keep the same): ").split(', ') # Implement the logic to edit a recipe # Example: self.recipe_manager.edit_recipe(pizza_name, new_name, new_type, new_ingredients, new_quantities) print(f"Recipe for '{pizza_name}' edited successfully.") def delete_recipe(self): print(" Deleting a Recipe") # Implement deleting a recipe pizza_name = input("Enter pizza name to delete: ") # Implement the logic to delete a recipe # Example: self.recipe_manager.delete_recipe(pizza_name) print(f"Recipe for '{pizza_name}' deleted successfully.") def search_recipes(self): print(" Searching Recipes") # Implement searching for recipes keyword = input("Enter keyword to search: ") results = self.recipe_manager.search_recipes(keyword) if results: print("Search results:") for result in results: print(result) else: print("No recipes found.") def customize_pizza_order(self): print(" Customize Your Pizza") selected_pizza = input("Select the pizza you want to customize: ") # Implement logic to offer pizza bases, sauces, toppings, and additional ingredients selected_base = input("Select pizza base (e.g., thin crust, pan): ") selected_sauce = input("Select pizza sauce (e.g., tomato, BBQ): ") # Offer toppings and additional ingredients available_toppings = ["Cheese", "Pepperoni", "Mushrooms", "Onions", "Peppers", "Sausage", "Bacon"] selected_toppings = self.select_ingredients("Select pizza toppings (comma-separated): ", available_toppings) # Calculate the total price based on the selected options total_price = self.calculate_custom_pizza_price(selected_base, selected_sauce, selected_toppings) # Create a CustomPizza object with the selected options and add it to the order custom_pizza = self.create_custom_pizza(selected_pizza, selected_base, selected_sauce, selected_toppings, total_price) self.order_manager.add_pizza_to_order(custom_pizza) print("Custom pizza added to your order.") def select_ingredients(self, prompt, available_ingredients): selected_ingredients = input(prompt).split(', ') # Validate selected ingredients against the available options for ingredient in selected_ingredients: if ingredient not in available_ingredients: print(f"Invalid ingredient: {ingredient}. Please select from the available options.") return self.select_ingredients(prompt, available_ingredients) return selected_ingredients def calculate_custom_pizza_price(self, selected_base, selected_sauce, selected_toppings): # Implement logic to calculate the total price for a custom pizza based on selected options base_price = 8.99 # Example base price topping_price = 1.5 # Example price per topping total_price = base_price + len(selected_toppings) * topping_price return total_price def create_custom_pizza(self, selected_pizza, selected_base, selected_sauce, selected_toppings, total_price): # Implement logic to create a CustomPizza object with the selected options base_ingredients = ["Dough", "Tomato Sauce", "Cheese"] # Example base ingredients custom_pizza = CustomPizza(selected_pizza, base_ingredients + selected_toppings, total_price) return custom_pizza def manage_side_dishes(self): while True: print(" Side Dish Management") print("1. Add New Side Dish") print("2. Remove Side Dish") print("3. Display Side Dishes") print("4. Return to Main Menu") choice = input("Enter the number of your choice: ") if choice == '1': self.add_new_side_dish() elif choice == '2': self.remove_side_dish() elif choice == '3': self.display_side_dishes() elif choice == '4': break else: print("Invalid choice. Please try again.") def add_new_side_dish(self): print(" Adding a New Side Dish") # Implement adding a new side dish side_dish_name = input("Enter side dish name: ") side_dish_price = float(input("Enter side dish price: ")) # Implement the logic to add the side dish # Example: self.menu_manager.add_new_side_dish(side_dish_name, side_dish_price) print(f"Side dish '{side_dish_name}' added successfully.") def remove_side_dish(self): print(" Removing a Side Dish") # Implement removing a side dish side_dish_name = input("Enter side dish name to remove: ") # Implement the logic to remove the side dish # Example: self.menu_manager.remove_side_dish(side_dish_name) print(f"Side dish '{side_dish_name}' removed successfully.") def display_side_dishes(self): print(" Displaying Side Dishes") # Implement displaying side dishes side_dishes = self.menu_manager.get_side_dishes() if not side_dishes: print("No side dishes available.") else: print("Side Dishes:") for side_dish in side_dishes: print(side_dish) def place_order(self): print(" Placing Order") # Implement placing an order order = self.order_manager.create_order() self.order_manager.display_order(order) confirm = input("Confirm order (yes/no): ").lower() if confirm == 'yes': # Implement logic to finalize the order # Example: order_id = self.order_manager.place_order(order) print("Order placed successfully. Your order ID is: XXX") else: print("Order canceled.") def display_main_menu(self): print(" Pizza Store Management System") print("1. Manage Recipes") print("2. Manage Inventory") print("3. Manage Menu") print("4. Customize Pizza Order") print("5. Manage Side Dishes") print("6. Place Order") print("7. Exit") # Run the application if __name__ == "__main__": pizza_store = PizzaStoreCLI() pizza_store.run() there is still errors in displaying menus , recipes and generating low stocks
Step by Step Solution
There are 3 Steps involved in it
Step: 1
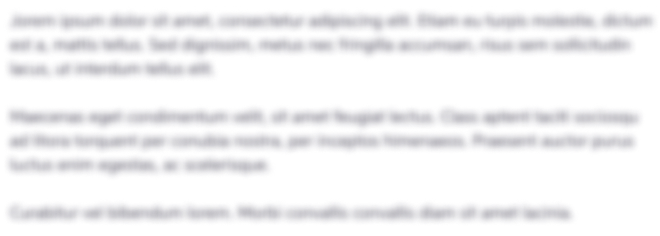
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started