Answered step by step
Verified Expert Solution
Question
1 Approved Answer
from socket import * from struct import pack, unpack import os , time, sys , logging , hashlib, math Protocol Description 1
from socket import
from struct import pack, unpack
import os time, sys logging hashlib, math
Protocol Description
Client initiates connection with the server
Client sends a request to the server to transfer a file. This request includes
the name of the file
the size of the file
the chunk size to be used during transfer
the checksum length used to validate the file chunks
Server responds with an ACK indicating it is ready to receive the file. The ACK uses the following format
ACK type
the next expected chunk number. This should be zero here as the server needs the first chunk
The client begins sending chunks of the file to the server. Chunks use the following format
the chunk number
the current file chunk
a checksum of the other data in this chunk
The server receives chunks and sends an ACK message. The ACK uses the following format:
ACK type
the next expected chunk number. Note: if the packet is received successfully, this is the next packet number. If the packet was NOT received successfully ie the checksum didn't validate then this should be the previously expected chunk number
This continues until the entire file has been received and the server saves the file and sends a final ACK to the client. The client can then either send another file, or close the connection and quit.
class FileTransferClient:
def initself host, port, chunksize hashlength:
Initializes the FileTransferClient.
This constructor sets up the logging configuration for the client and stores and provided parameters.
Parameters:
host string: the hostname or IP address to connect to
port int: The port number to connect TODO
chunksize int: the number of bytes in each chunk to use when transmitting the file
hashlength int: the length of the hash in bytes
Note:
The logger setup provided should not be modified and is used for outputting
status and debugging messages.
# Do not modify this logger code. You can continue to use print commands for your code.
# The logger is in place to ensure that you receive proper output and debugging messages from the tester
self.logger logginggetLoggerCLIENT
self.handler loggingStreamHandlersysstdout
formatter loggingFormatterasctimes levelnames messages
self.handler.setFormatterformatter
self.loggeraddHandlerselfhandler
self.loggerpropagate False
self.loggerinfoInitializing client..."
# End of logger code
self.host host
self.port port
self.chunksize chunksize
self.hashlength hashlength
self.clientsocket None
def startself:
Initiates a connection to the server and marks the client as running. This function
does not attempt to send or receive any messages to or from the server.
This method is responsible for establishing a socket connection to the specified
server using the host and port attributes set during the client's initialization.
It creates a TCP socket object and attempts to connect to the server. It logs
the connection attempt and reports any errors encountered during the process.
Returns:
True or False depending on whether the connection was successful.
Exceptions:
OSError: Raised if the socket encounters a problem eg network
issue, invalid hostport
Usage Example:
client FileTransferClienthost port hashlength
if client.start:
do something
Note:
It is important to call this method before attempting to send or receive
messages using the client.
Ensure that the server is up and running at the specified host and port
before calling this method.
# TODO: Implement the functionality described in this function's docstring
pass
def readfileself filepath:
Reads the contents of a file and returns the data as a byte object.
This is a helper function. You do not need to modify it You can use it in the sendfile
method to read the contents of a file and return the data as a byte object.
with openfilepathrb as file:
data file.read
return data
def sendfileself filepath:
Sends a file to the server using the established connection.
File Transfer Protocol:
Send a file transfer request to the server. Generate this request using the
packtransferrequestmessage function
Receive
Step by Step Solution
There are 3 Steps involved in it
Step: 1
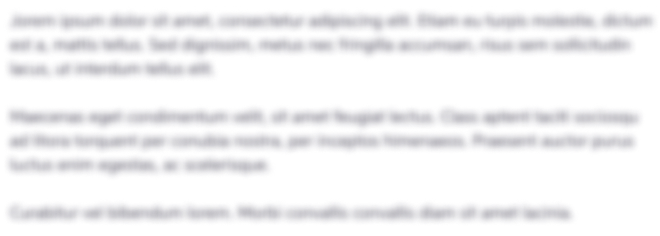
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started