Question
// funcs.cpp #include using namespace std; template struct BinaryNode { T element; BinaryNode* left; BinaryNode* right; BinaryNode(const T & d = T()): element(d) { left
// funcs.cpp #includeusing namespace std; template struct BinaryNode { T element; BinaryNode* left; BinaryNode* right; BinaryNode(const T & d = T()): element(d) { left = nullptr; right = nullptr; } }; //print the elements of binary tree in preorder template void preorder(const BinaryNode * root) { // add your code } //print the elements of binary tree in inorder template void inorder(const BinaryNode * root) { // add your code } //print the elements of binary tree in postorder template void postorder(const BinaryNode * root) { // add your code }
================================================================================================= ================================================================================================= =================================================================================================
// lab06.cpp #include "funcs.cpp" BinaryNode* create_binary_tree() { BinaryNode * node_A = new BinaryNode ('A'); BinaryNode * node_B = new BinaryNode ('B'); BinaryNode * node_C = new BinaryNode ('C'); BinaryNode * node_D = new BinaryNode ('D'); BinaryNode * node_E = new BinaryNode ('E'); node_A->left = node_B; node_A->right = node_C; node_B->left = node_D; node_B->right = node_E; return node_A; } int main() { BinaryNode * root = create_binary_tree(); // add your code // call traversal functions to print elements }
// funcs.cpp
#include
#include "Stack.h"
#include "Queue.h"
using namespace std;
template
struct BinaryNode {
T element;
BinaryNode* left;
BinaryNode* right;
BinaryNode(const T & d = T()): element(d)
{
left = nullptr;
right = nullptr;
}
};
//print the elements of binary tree in preorder
template
// add your code
}
//print the elements of binary tree in level-order template
// add your code }
//
public:
void printRange() {
printRange(root,k1, k2); }
private:
void printRange(BinaryNode* t, int k1, int k2) {
// add your code
}
================================================================================================= ================================================================================================= BinarySearchTree.h // after Mark A. Weiss, Chapter 4, Dr. Kerstin Voigt #ifndef BINARY_SEARCH_TREE_H #define BINARY_SEARCH_TREE_H #include #include using namespace std; template class BinarySearchTree { public: BinarySearchTree( ) : root{ nullptr } { } ~BinarySearchTree( ) { makeEmpty(); } bool isEmpty( ) const { return root == nullptr; } const C & findMin( ) const { assert(!isEmpty()); return findMin( root )->element; } const C & findMax( ) const { assert(!isEmpty()); return findMax( root )->element; } bool contains( const C & x ) const { return contains( x, root ); } void printTree( ) const { if( isEmpty( ) ) out left == nullptr ) return t; return findMin( t->left ); } // Internal method to find the largest item in a subtree t. // Return node containing the largest item. BinaryNode* findMax( BinaryNode* t ) const { if( t != nullptr ) while( t->right != nullptr ) t = t->right; return t; } // Internal method to test if an item is in a subtree. // x is item to search for. // t is the node that roots the subtree. bool contains( const C & x, BinaryNode* t ) const { if( t == nullptr ) return false; else if( x element ) return contains( x, t->left ); else if( t->element right ); else return true; // Match } void printTree( BinaryNode* t) const { if( t != nullptr ) { printTree( t->left); cout element right); } } void makeEmpty( BinaryNode* & t ) { if( t != nullptr ) { makeEmpty( t->left ); makeEmpty( t->right ); delete t; } t = nullptr; } // Internal method to insert into a subtree. // x is the item to insert. // t is the node that roots the subtree. // Set the new root of the subtree. void insert( const C & x, BinaryNode* & t ) { if( t == nullptr ) t = new BinaryNode{ x, nullptr, nullptr }; else if( x element ) insert( x, t->left ); else if( t->element right ); else ; // Duplicate; do nothing } // Internal method to remove from a subtree. // x is the item to remove. // t is the node that roots the subtree. // Set the new root of the subtree. void remove( const C & x, BinaryNode* & t ) { if( t == nullptr ) return; // Item not found; do nothing if( x element ) remove( x, t->left ); else if( t->element right ); else if( t->left != nullptr && t->right != nullptr ) // Two children { t->element = findMin( t->right )->element; remove( t->element, t->right ); } else { BinaryNode* oldNode = t; if ( t->left == nullptr ) t = t->right; else t = t->left; delete oldNode; } } }; #endif
=================================================================================================
Note: Please answer all these questions to receive credit.
=================================================================================================
Thank you.
Write a C+program to print the elements of hinary trees using preorder, inorder, and postorder traversal. The program includes the following: 1. A) Declarand implemenl lun11uns preorder, inorder, and post order in the ile funcs. cpp. funce.cpp includeciostream> uaing nanespace atd; emplatetyename r struct inaryNode T element: BinaryNode left; BinaryHode right Binarynode {const T & d = T()}: lement (d) left = nullptr; rightnullptri print the eleaents o binary tree in preorder tamplataStep by Step Solution
There are 3 Steps involved in it
Step: 1
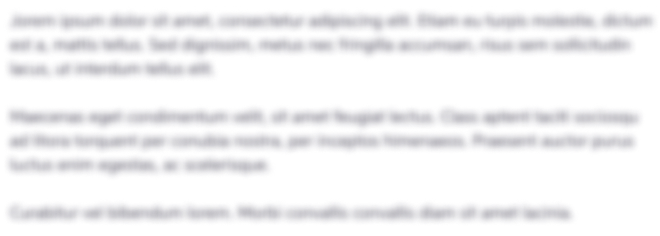
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started