Question
function [x] = example53(xinitial) % example53.m: 3-position synthesis for 4-bar linkage % for the specifications in problem 5-3 in the textbook % Input: % xinitial
function [x] = example53(xinitial)
% example53.m: 3-position synthesis for 4-bar linkage
% for the specifications in problem 5-3 in the textbook
% Input:
% xinitial (optional) initial gues, a 3N+10 x 1 matrix
%
% Output:
% x solution, a 3N+10 x 1 matrix
% data from steps 2 and 3 on page 256
% Px,Py,Theta(deg) for each of the N positions
clear global
global specs
specs.PQ = [ ...
0 0 0 ; ... % first position is defined as being at origin and zero angle
-0.244 0.013 -11.34 ; ... % second position
-0.542 0.029 -22.19 ]; % third position
% specified pivots from step 4 on page 257
specs.O2 = [-1.712 0.033]; % specified values for O2x and O2y
specs.O4 = [0.288 0.033]; % specified values for O4x and O4y
% convert column 3 of specs.PQ into radians
specs.PQ(:,3) = specs.PQ(:,3) * pi/180;
% determine how many positions there are (i.e. rows of specs matrix)
N = size(specs.PQ,1);
if (N ~= 3)
error('This code was written for 3-position synthesis only.');
end
% the unknowns are:
% x(1..2) global position of fixed pivot O2
% x(3..4) global position of fixed pivot O4
% x(5..7) link lengths a,b,c
% x(8..9) local coordinates of point P on the coupler
% x(10) angle between PQ and AB
% x(11...) angles theta2, theta3, theta4 in all N positions (3*N unknowns)
Nunknowns = 10 + 3*N;
% if no initial guess was provided, use random numbers
if nargin
xinitial = rand(Nunknowns,1);
end
% we may need many attempts to find a solution, allow 100 attempts
for i = 1:100
% solve the unknowns x (design parameters, and the N positions)
% from a set of simultaneous equations: func(x) = 0
% NRsolve does the same as Matlab's fsolve but the graphics
% is faster if you use NRsolve
[x, info] = NRsolve(@func, xinitial, 40); % allow 40 iterations in each attempt
if (info == 0)
break
end
disp('Hit ENTER to start a new attempt, or CTRL-C to quit');
pause
% make a new random initial guess
xinitial = rand(Nunknowns,1);
end
end
%============================================================================
function draw(x)
% draw the trial solution x
global specs
N = size(specs.PQ,1);
% extract the mechanism design parameters and print them
O2x = x(1);
O2y = x(2);
O4x = x(3);
O4y = x(4);
a = x(5);
b = x(6);
c = x(7);
Pxloc = x(8);
Pyloc = x(9);
PQangle = x(10);
fprintf('Design parameters: ');
fprintf('O2: %8.4f %8.4f ', O2x,O2y);
fprintf('O4: %8.4f %8.4f ', O4x,O4y);
fprintf('Link a: %8.4f ', a);
fprintf('Link b: %8.4f ', b);
fprintf('Link c: %8.4f ', c);
fprintf('Pxloc: %8.4f ', Pxloc);
fprintf('Pyloc: %8.4f ', Pyloc);
fprintf('Angle between PQ and AB: %8.4f deg ', PQangle*180/pi);
% draw the mechanism in the N specified positions
figure(1);
clf;
positioncolors = colormap('lines');
for i = 1:N
% extract the positions of A,B (4 unknowns per position)
theta2 = x(10 + 3*(i-1) + 1);
theta3 = x(10 + 3*(i-1) + 2);
theta4 = x(10 + 3*(i-1) + 3);
% draw the ground link O2-O4 as a solid black line
plot([O2x O4x],[O2y O4y],'k','LineWidth', 3);
hold on
% draw the links O2-A-B-O4 in the color that belongs to position i
Ax = O2x + a*cos(theta2);
Ay = O2y + a*sin(theta2);
Bx = Ax + b*cos(theta3);
By = Ay + b*sin(theta3);
plot([O2x Ax Bx O4x], [O2y Ay By O4y], '-o', 'Color', positioncolors(i,:), 'LineWidth', 1.5);
% do coordinate transformation to calculate where the the coupler point P is
Px = Ax + Pxloc*cos(theta3) - Pyloc*sin(theta3);
Py = Ay + Pxloc*sin(theta3) + Pyloc*cos(theta3);
% draw the coupler APB as a triangle
plot([Ax Px Bx Ax], [Ay Py By Ay], '-o', 'Color', positioncolors(i,:), 'LineWidth', 1.5);
% add some text labels
text(Ax, Ay, ' A', 'FontSize',12);
text(Bx, By, ' B', 'FontSize',12);
text(Px, Py, ' P', 'FontSize',12);
axis('equal');
end
end
%============================================================================
function [f] = func(x,display)
% equations for the N-position synthesis problem, in the form f(x) = 0
% there are
% 3N+10 unknowns x
% 5N equations f(x) = 0 from the linkage and performance specs
% 3N+10-5N equations from free choices
global specs
N = size(specs.PQ,1);
% do some error checking on input x
Nunknowns = 3*N+10;
if (size(x,1) ~= Nunknowns) || (size(x,2) ~= 1)
error('func: x was not a matrix of size (Nunknowns x 1)');
end
% extract the linkage parameters from x
O2x = x(1);
O2y = x(2);
O4x = x(3);
O4y = x(4);
a = x(5);
b = x(6);
c = x(7);
d = sqrt((O4x-O2x)^2 + (O4y-O2y)^2); % length of ground link
Pxloc = x(8);
Pyloc = x(9);
PQangle = x(10);
% generate the 5 equations from each of the N positions
f = zeros(Nunknowns,1);
for i = 1:N
% extract the positions of points A,B in position i, from x
theta2 = x(10 + 3*(i-1) + 1);
theta3 = x(10 + 3*(i-1) + 2);
theta4 = x(10 + 3*(i-1) + 3);
% do coordinate transformation to calculate where the the coupler point P is
Ax = O2x + a*cos(theta2);
Ay = O2y + a*sin(theta2);
Px = Ax + Pxloc*cos(theta3) - Pyloc*sin(theta3);
Py = Ay + Pxloc*sin(theta3) + Pyloc*cos(theta3);
% 2 equations from the vector loop
f(5*(i-1) + 1) = O2x + a*cos(theta2) + b*cos(theta3) - c*cos(theta4) - O4x;
f(5*(i-1) + 2) = O2y + a*sin(theta2) + b*sin(theta3) - c*sin(theta4) - O4y;
% 2 equations that require point P to be at specified locations in position i
f(5*(i-1) + 3) = Px - specs.PQ(i,1); % specified Px is in row i, column 1 of specs.PQ matrix
f(5*(i-1) + 4) = Py - specs.PQ(i,2); % Py is in column 2
% 1 equation that requires line PQ to be at specified angle in position i
% (orientation of line PQ is orientation of line AB plus PQangle)
f(5*(i-1) + 5) = theta3 + PQangle - specs.PQ(i,3);
end
% now generate extra equations that represent the free choices
if (N == 2)
error('Code for 2-position synthesis is not available in this version.');
elseif (N == 3)
% for 3-position synthesis, there are 4 free choices
% here we require specified locations for the fixed pivots O2 and O4
f(5*N+1) = O2x - specs.O2(1);
f(5*N+2) = O2y - specs.O2(2);
f(5*N+3) = O4x - specs.O4(1);
f(5*N+4) = O4y - specs.O4(2);
else
error('Free choices were not programmed yet for the %d-position synthesis', N);
end
% at each iteration, print x and f side by side
if (nargin > 1)
[x f]
% also print the norm of f (which should go to zero during the solution process
fprintf('Norm of f: %f ', norm(f));
draw(x);
pause(0.001);
end
end
%=======================================================================
function [x, info] = NRsolve(fun, x0, maxiterations)
% solves f(x) = 0 using Newton-Raphson method
% input:
% fun...........pointer to function that computes f(x)
% x0............initial guess for solution
%
% output:
% x.............solution of f(x) = 0
if (nargin
maxiterations = 20; % maximum number of iterations
end
tolerance = 1e-8; % iterations will stop if norm(f)
x = x0; % start with x at initial guess
for i = 1:maxiterations
[f,A] = evaluate(fun, x); % evaluate function and Jacobian matrix
if norm(f)
disp('solved.');
info = 0; % info=0 indicates success
return; % return to main program
end
dx = A\f; % solve A*dx = f
x = x - dx; % move x towards the solution of f(x) = 0
% if norm f did not decrease, undo half of the step dx, and so on
nbacktrack = 0;
while ( norm(fun(x)) > norm(f) ) && nbacktrack
dx = dx/2;
x = x + dx;
nbacktrack = nbacktrack + 1;
end
end
% if we arrive here, we have done too many iterations
disp('a solution could not be found');
% info = 1 indicates that the solution was not found
info = 1;
end
%========================================================================
function [f,A] = evaluate(fun, x)
% evaluates a function fun(x) and estimates its Jacobian matrix at x
Nx = numel(x);
f = fun(x,1); % the "1" causes something to be printed within fun
h = 1e-5; % perturbation step size for derivative estimation
for i = 1:numel(x)
x(i) = x(i) + h; % perturb x(i)
newf = fun(x);
x(i) = x(i) - h; % restore original x(i)
A(:,i) = (newf - f) / h; % one column of Jacobian matrix
end
end
Homework 7.pdf 12 MICE 260 Spring 2018 PRESENT CLEARLY HOW YOU DEVELOPED THE SOLUTION TO THE PROBLEMS Each problem is worth up to S points. Points are given as follows: points: points: Work was complete, but not deariy presented or some errors in cakcuation work w complete and presented deernthe answer is correct 3 points: Some errors oromissians in methods or presentation 2 points: Majorerrors or omissions in methods or presentation 1 point Problm was understoed but incorrect approach was usod 1. Modify the Matlab code example53m to design a fourbar inkage that moves the box through the three positions shown. Use the fixed pivots that are shown in the disgram. snore the attachment points A and B lbut don't be surprsed your design process produkes similar attachment points). 2. Modify the Matlab code examples3.m to design a fourbar Inkage that moves the box through the three posltions shown. All maving links must have lengths that are mult ples o 10mm. 3. Modify the Matlab code exarmple53opt m to desnaourbar lirkage that moves the box through the three positions shown, Make an objective function x) to get the fixed piwots as close as passible to the IMPORTANT: For problems 1-3, your sclution should include: lal the design parameters of the linkage, (b) the Figure produced by Matlab, (c) the lines of Matlab coce that you changed (and no other codel), and (d) do you think there are ary cireuit, branch, ar ordor (CRO) detects (based on visual inspection only)? contiued on next page) Page 1 of 2Step by Step Solution
There are 3 Steps involved in it
Step: 1
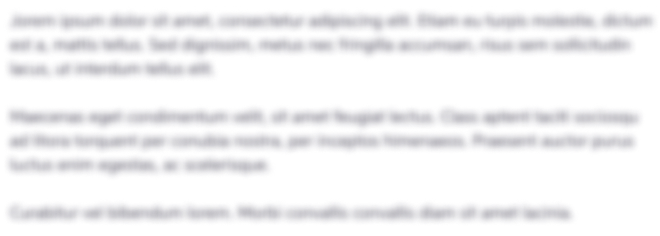
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started