Question
Functionality The main objective is to make View display the information about 3 students. The students are created in Model. Controller will retrieve the data
Functionality
The main objective is to make View display the information about 3 students. The students are created in Model. Controller will retrieve the data from Model and pass it to View. View will use "System,out.println" statements to display the following result:
NAME = Emily Smith Age = 20, address=107 W College Avenue,State College,PA,16801 11 credits NAME = Mary Doe Age = 20, address=200 W College Avenue,State College,PA,16801 13 credits NAME = John Doe Age = 20, address=300 W College Avenue,State College,PA,16801 6 credits
------
NetBeans Starter file with classes:
App
- it has the main method which is the method that Java looks for and runs to start any application
- it creates 3 objects, one of the Model class, one of the View class and one of the Controller class.
- When it creates Controller, it passes the two other objects as input parameters so that Controller has access to Model and View.
import Controller.Controller; import Model.Model; import View.View;
class App {
public static void main(String[] args) { Model model = new Model(); View view = new View(); Controller controller = new Controller(model, view); } }
Controller
- will retrieve from Model (using the model object) data about each student to be displayed
- will pass the data to View, which will display it
- the two operations above will work best if Model and View have methods that help with that.
- You are writing these methods
- For instance, controller might look like this:
package Controller;
import Model.Model; import View.View; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.Scanner; import javax.swing.JButton;
public class Controller {
Model model; View view; int k = 0;
public Controller(Model model, View view) { this.model = model; this.view = view; }
}
Model
- it is already given in the starter project although you will need to add some functionality
- it needs a method that returns data about a specific student in the array (you will write this method or a similar one)
package Model;
import java.util.ArrayList;
public class Model {
ArrayList
public Model() { //creates 3 students MailAddress addr1 = new MailAddress("107 W College Avenue", "State College", "PA", 16801); Student st1 = new Student("Emily", "Smith", 20, addr1); MailAddress addr2 = new MailAddress("200 W College Avenue", "State College", "PA", 16801); Student st2 = new Student("Mary", "Doe", 20, addr2); MailAddress addr3 = new MailAddress("300 W College Avenue", "State College", "PA", 16801); Student st3 = new Student("John", "Doe", 20, addr3); //add them to the array of students sts.add(st1); sts.add(st2); sts.add(st3); }
}
MailAddress
- it is used by the Student class and doesn't need any updates
package Model;
public class MailAddress {
String streetAddress; String city; String state; int zipcode;
public MailAddress(String inf_streetAddress, String inf_city, String inf_state, int inf_zipcode) { streetAddress = inf_streetAddress; city = inf_city; state = inf_state; zipcode = inf_zipcode; }
@Override public String toString() { return streetAddress + "," + city + "," + state + "," + zipcode; }
}
- Student
- it is used in Model and doesn't need any updates.
package Model;
public class Student { //---------Declaring attributes----
String firstName; String lastName; int age; MailAddress address; int credits; //------------------------------ //----------Constructor------------
public Student(String a, String b, int c, MailAddress d) { firstName = a; lastName = b; age = c; address = d; credits = creditsThisSemester(); }
//---------- METHODS -------- public String getInfo() { return "NAME = " + firstName + " " + lastName + " " + "Age = " + age + ", address=" + address.toString() + " credits=" + credits; }
//------------------------------------------------ public int creditsThisSemester() { //calculate randomly a new value for credits in the semester //and updates the attribute credits double dn = Math.random(); credits = (int) (15.0 * dn); return credits; } }
View
- In this assignment, it is a very simple class
- it just needs to display the text data that it will receive from Controller
- It needs a method to do this
package View;
import java.util.ArrayList; import javax.swing.JButton;
public class View {
public View() { }
}
Do not use the scanner class or any other user input request. You application should be self-contained and run without user input. Assignment Objectives 1. Practice on implementing a MVC project Deliverables A zipped Java project according to the How to submit Labs and Assignments guide. 0.0. Requirements (these items will be part of your grade) 1. One class, one file. Don't create multiple classes in the same.java file 2. Don't use static variables and methods 3. Encapsulation: make sure you protect your class variables and provide access to them through get and set methods 4. all the classes are required to have a constructor that receives all the attributes as parameters and update the attributes accordingly 5. Follow Horstmann's Java Language Coding Guidelines 6. Organized in packages (MVC - Model - View Controller) Contents Model #1 - Controller asks Model for student data as text student Controller View #3 - View receives the data and displays it as text #2 - Controller passes student data as text to to View and asks View to displays it as text WWE - Emily Smith Age - 20, address-187 College Avenue, State College, PA,16801 11 credits WWE - Mary Die Age = 20, address=290 College Avenue, State College, PA, 16801 13 credits WWE - John Die Age : 2), addressCollege Avenue, State College, PA, 168116 credits The Netbeans Project You are required to use this NetBeansstarter project. This assignment focus on using the MVC architecture. The main components are the three packages, Model, View and Controller. From the lesson you know that View objects are objects that the users can see, the graphical part of an application. Model objects will have all the data and processing needs of an application. The Controller objects will usually help and support the communication and data transfer between Model and view objects. Prelec Services 9103C Starter Source Packages
Step by Step Solution
There are 3 Steps involved in it
Step: 1
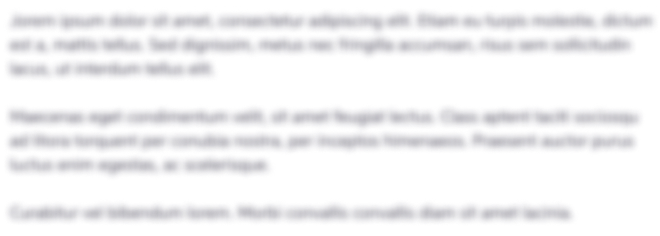
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started