Question
Game of Pig Rules The game requires two dice. Players take turns until someone wins by earning at least 100 points. A Player begins a
Game of Pig Rules
The game requires two dice.
Players take turns until someone wins by earning at least 100 points.
A Player begins a round (for a players turn), by rolling both dice and tallying the points. The current player may continue to roll and accumulate points until rolling a 1 on either of the two dice or choosing to hold. Whenever a player rolls a 1 on either of the two dice, all points for the round are lost. Whenever a player rolls a pair of 1s, (i.e. 1, 1) all player points are lost, and the players score starts over at 0.
The game turn advances to the next player.
For this Game of Pig project, the game will be between you and the computer, where you may have the first turn.
Project Requirements
For each of the provided classes that make up the project, do not create additional methods or fields. Do not make any changes to the following requirements without approval from your instructor.
Step 6: Implement methods in the PigGame class
Class Fields
dice as a Dice object, player, turn as 0 or 1, turnsTaken, and the roundScore.
Constant identifiers for a user index, a computer index, and the winning score.
Constructor
public PigGame( ) - a constructor that prints a welcome message and
instantiates dice
instantiates and populates the player array,
initializes turn, turnsTaken, and roundScore
Accessor Methods do not modify class fields.
public int getRoundScore( ) returns the round score.
public GVdie getDie( int n ) - returns the requested die. Legal values for the parameter n are 0 or 1.
public boolean playerWon( int n ) - returns true if either the player score or the computer score is currently high enough to win. Otherwise, returns false. Legal values for the parameter n are 0 or 1.
private boolean noWinnerYet( ) returns true if neither the USER player nor the COMPuter player is a winner, otherwise returns false.
Mutator Methods
The playerRolls and playerHolds methods, as designed below, are used by both players: user and computer.
public void restart( ) resets appropriate instance variables to start a new game. Do not instantiate new dice objects.
public void nextTurn( ) advances turn by 1 modulus 2, increments turnsTaken, and resets the round score to zero
public void playerRolls( ) - performs the first half of the player turn:
print the players name and score if the round score is 0.
invoke the dice.roll( )
If either die value is '1' then set the round score to 0. Otherwise, increment the round score by the new total.
Print the round score. Refer to the sample output below.
public void playerHolds( ) - performs the second half of the player's turn:
1) update the player's score by either adding the roundScore to the player score or setting the player score to zero if double 1s are rolled.
2) print the player's name and score. Refer to the sample output below.
3) print an appropriate message if the player wins.
4) invoke nextTurn to transfer control to the next player
private Boolean autoPlayContinues returns the boolean value for an expression where the roundScore is not 0, the players score plus the round score is less than 100, and the round score is less than 19 (i.e. game strategy)
public void play( ) - performs an entire turn
if the player[ turn ] is on auto
Uses a do while loop to repeatedly call playerRolls as long as autoPlayContinues is true
Calls playerHolds.
else call playerRolls once for one roll
public void autoPlay( ) - Restarts the game, sets both players to isOnAuto, and
repeatedly calls the play method in a do .. while loop as long as there is no winner.
public boolean isPlayerTurn( int n ) - this one line method returns true if it is the player's turn or false if it is the computer's turn.
Sample Text Output The following sample shows partial results of a game. Your messages can be more creative as long as they convey the necessary information. Note: the total number of rounds is only displayed at the end of the game.
Welcome to the game of Pig.
Amanda: 0
2, 4 Current Round Score: 6
4, 2 Current Round Score: 12
3, 4 Current Round Score: 19
Amanda: 19
computer: 0
5, 5 Current Round Score: 10
1, 1 Current Round Score: 0
computer: 0
later in the game
Amanda: 75
6, 1 Current Round Score: 0
Amanda: 75
computer: 94
1, 1 Current Round Score: 0
computer: 0
Amanda: 75
3, 5 Current Round Score: 8
3, 5 Current Round Score: 16
5, 6 Current Round Score: 27
Amanda: 102
Amanda wins!
10 rounds
piggame ------------------------------------------------
------------------------------------------
public class PigGame { private Dice dice; public Player[ ] player; private int turn; private int turnsTaken; private int roundScore; // the current round score
// CONSTANTS public static final int USER = 0; // user index public static final int COMP = 1; // computer index private static final int WINNING_SCORE = 100;
public PigGame( ) { this.dice = new Dice( 2 ); this.player = new Player[ 2 ]; this.player[ USER ] = new Player( ); this.player[ COMP ] = new Player( "computer" );
this.restart( ); } /** * resets appropriate instance variables to start a new game. * Do not instantiate new dice objects. */ public void restart( ) { System.out.print( "\f" ); System.out.println( "Welcome to the game of Pig. " );
this.player[ USER ].score = 0; this.player[ COMP ].score = 0;
this.turn = COMP; // or USER this.turnsTaken = 0; this.nextTurn( ); }
/** * advances turn by 1 modulus 2, * increments turnsTaken, and * resets the round score to zero */ private void nextTurn( ) {
} /** * returns the roundScore */ public int getRoundScore( ) { return -1; }
/** * This method is needed by the PigQUI class. * * returns the requested die, * dice.die[0] or dice.die[ 1 ], * from the public dice field. * * Legal values for the parameter n are 0 or 1. */ public GVdie getDie( int n ) { return this.dice.die[ n ]; }
/** * returns true if either the player score or the * computer score is currently high enough to win. * Otherwise, returns false. * Legal values for the parameter n are 0 or 1. */ public boolean playerWon( int n ) { return false; }
/** * returns true if neither the USER player nor the * COMPuter player is yet a winner, otherwise returns false. */ public boolean noWinnerYet( ) { return false; }
/** * performs the first half of the player turn */ public void playerRolls( ) { // 1) print the player's name and score if the round score is 0.
// 2) invoke the dice.roll( )
// 3) If either die value is '1' then set the round score to 0. // Otherwise, increment the round score by the new total.
// 4) Print the dice followed by the round score. // See the sample output in the project description.
}
/** * performs the second half of the player's turn */ public void playerHolds( ) { //1) update the player's score by either adding the // roundScore to the player score or setting the // player score to zero if double 1s are rolled.
// 2) print the player name and score. See the sample output in the project description.
// 3) print an appropriate message if the player wins.
//4) invoke nextTurn
}
/** * returns the boolean value for an expression where * the roundScore is not 0, * the players score plus the round score is less than 100, and * the round score is less than 19 (i.e. game strategy) */ private boolean autoPlayContinues() { return ( true ); }
/** * performs an entire turn */ public void play( ) { if (this.player[turn].isOnAuto) { // 1) For a whole turn, use a do ... while loop to repeatedly call // playerRolls as long as autoPlayContinues returns true
// 2) Call playerHolds, which completes the turn.
} else { // 3) Call playerRolls once for one round as this is not the whole turn
} }
/** * This method is needed by the PigQUI class * * this one line method returns true if it is the * player's turn or false if it is the computer's turn. */ public boolean isPlayerTurn( int n ) { return false; }
/** * Restarts the game, * sets both players to isOnAuto, and * repeatedly calls play in a do .. while loop * as long as there is no winner. */ public void autoPlay( ) { this.restart( ); this.player[ USER ].isOnAuto = true; this.player[ COMP ].isOnAuto = true;
} public static void main( String[ ] args ) { PigGame pig = new PigGame(); pig.autoPlay( ); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
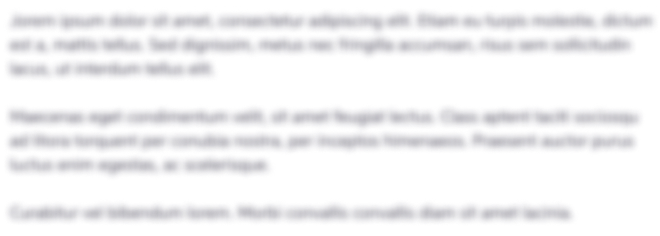
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started