Question
Game.java Update class to add the following member variables int trumpCheck Team trumpTeam Add a getter for member variable trumpCheck Update customer constructor Game() to
Game.java | Update class to add the following member variables int trumpCheck Team trumpTeam |
| Add a getter for member variable trumpCheck Update customer constructor Game() to do the followingComment out the call the method play() Update method createTeams() to do the followingOn the instance of class HumanPlayer, call method setGame() passing as an argument a reference to this class Game On the instance of class AiPlayer, call method setGame() passing as an argument a reference to this class Game Update method play() to do the followingComment out any current code Add a call to method trumpCheck Add method trumpCheck to do the followingReturn type void Empty parameter list Initialize member variable trumpCheck to the value of 0 Create a local variable of data type int to represent the current player, initialized to the member variable leadIdx Loop while the value of member variable trumpCheck is less than the number of playersInstantiate an instance of class Player set equal to member variable getting the player at the position represented by local variable created in step d. (i.e. starting with the lead player) On the Player object from above, call method makeTrump() Check if the Player object accepted the trump by calling method getAcceptTrump()If true, loop through the member variable of data type ArrayList that stores the teams in the game Check if the current team contains the current player (HINT: use method contains() that is part of the class ArrayList)If true, set the member variable trumpTeam equal to the current team Display a dialog message to state which team has accepted the trump suit Break out of the while loop if trump has been accepted ElseIncrement the member variable trumpCheck by one Increment the local variable representing the current player by one; be sure to check if the counter is equal to 3, then reset it to 0 (e.g. remember, the table member variable has only 4 elements so the maximum index is 3) |
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package core;
/** * * @author */
import constants.Constants; import constants.Constants.Suit; import java.util.ArrayList; import java.util.Iterator; import java.util.Random; import java.util.Scanner; import javax.swing.JFrame; import javax.swing.JOptionPane; import userinterface.GameUi;
public class Game { // member variables private Card trump; private Player leadPlayer; private Player dealer; private Player wonTrick; private int round; private ArrayList
private void outputTeams() { for(Team team : teams) { team.outputTeam(); } } private void setTable() { // players are set up so that team members sit across from each other // therefore the deal would be to TeamOne.PlayerOne, TeamTwo.PlayerTwo, // TeamOne.PlayerTwo, TeamTwo.PlayerTwo as an example table = new ArrayList(); // get the teams in the game Team teamOne = teams.get(Constants.ONE); Team teamTwo = teams.get(Constants.TWO); // get the players from each team Player teamOnePlayerOne = teamOne.getTeam().get(Constants.ONE); Player teamOnePlayerTwo = teamOne.getTeam().get(Constants.TWO); Player teamTwoPlayerOne = teamTwo.getTeam().get(Constants.ONE); Player teamTwoPlayerTwo = teamTwo.getTeam().get(Constants.TWO); // we want to explicitly dictate which seat each player is in so we are // using the add method that takes two arguments, one to set the position // in the ArrayList and the associated object at that position table.add(0, teamOnePlayerOne); table.add(1, teamTwoPlayerOne); table.add(2, teamOnePlayerTwo); table.add(3, teamTwoPlayerTwo); System.out.println("************************"); System.out.println("Players at the table are"); System.out.println("************************");
for(Player player : table) { System.out.println(player.getName()); } } private void setDealerAndLead() { // select the first dealer Random random = new Random(); dealerIdx = random.nextInt(Constants.NUM_PLAYERS); dealer = table.get(dealerIdx); // create an index to keep track of which player got the card; // reset when get to 3 // set the leadIdx based on which player was selected as the dealer and // add one to it if(dealerIdx < 3) leadIdx = dealerIdx + 1; else leadIdx = 0; leadPlayer = table.get(leadIdx); } private void dealHand() { setDealerAndLead(); System.out.println("********************************"); System.out.println(" DEALING THE HAND"); System.out.println("********************************");
System.out.println("Player " + dealer.getName() + " will deal the hand");
int playerIdx = leadIdx; // loop through the shuffled deck and deal five cards to each player // first round, two at a time // second round, three at a time Iterator
for(int p = 0; p < Constants.NUM_PLAYERS; p++) { dealOne(playerIdx, currentCard); // increment the player index until value of 3, then reset to 0 if(playerIdx == 3) playerIdx = 0; else playerIdx++; }
// System.out.println("********************************"); // System.out.println(" SECOND DEAL, THREE CARDS EACH"); // System.out.println("********************************");
for(int p = 0; p < Constants.NUM_PLAYERS; p++) { dealTwo(playerIdx, currentCard); // increment the player index until value of 3, then reset to 0 if(playerIdx == 3) playerIdx = 0; else playerIdx++; } // set trump to next card on deck trump = currentCard.next(); System.out.println("********************************"); System.out.println("Trump card is " + trump.getFace() + " of " + trump.getSuit() + " color " + trump.getColor()); System.out.println("********************************"); }
public Card getTrump() { return trump; } private void dealOne(int playerIdx, Iterator
// System.out.println("Dealing " + card.getFace() + " of " + // card.getSuit() + " to player " + // table.get(playerIdx).getName()); // add card to a player's hand table.get(playerIdx).getHand().add(card); // remove the card from the deck after it has been dealt currentCard.remove(); } } } private void dealTwo(int playerIdx, Iterator
// System.out.println("Dealing " + card.getFace() + " of " + // card.getSuit() + " to player " + // table.get(playerIdx).getName()); // add card to a player's hand table.get(playerIdx).getHand().add(card);
// remove the card from the deck after it has been dealt currentCard.remove(); } } } private void displayHands() { for(Team team : teams) { team.outputHands(); } } private void createDeck() { deck = new Deck(); } public void play() { for(Player player : table) { player.makeTrump(); } } public void setGameUi(GameUi ui) { this.ui = ui; } /** * @return the wonTrick */ public Player getWonTrick() { return wonTrick; }
/** * @param wonTrick the wonTrick to set */ public void setWonTrick(Player wonTrick) { this.wonTrick = wonTrick; }
/** * @return the round */ public int getRound() { return round; }
/** * @param round the round to set */ public void setRound(int round) { this.round = round; }
/** * @return the leadPlayer */ public Player getLeadPlayer() { return leadPlayer; }
/** * @param leadPlayer the leadPlayer to set */ public void setLeadPlayer(Player leadPlayer) { this.leadPlayer = leadPlayer; }
/** * @return the dealer */ public Player getDealer() { return dealer; }
/** * @param dealer the dealer to set */ public void setDealer(Player dealer) { this.dealer = dealer; }
/** * @return the table */ public ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
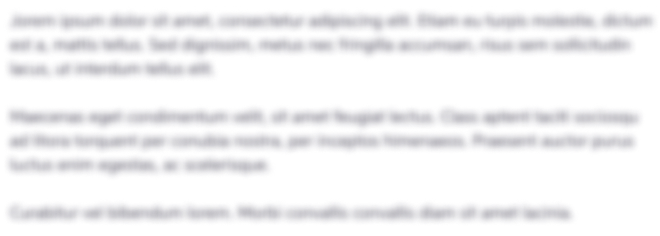
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started