Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Generics The goal: Use two functional interfaces to implement helpful methods that take and change lists of any element type. All classes must follow








Generics The goal: Use two functional interfaces to implement helpful methods that take and change lists of any element type. All classes must follow all good design and coding practices, including good design of classes, code indenting, appropriate comments, and readable and meaningful output. First, create these two functional interfaces: @FunctionalInterface public interface Predicate { boolean test (T t); } @FunctionalInterface public interface Function { T apply (T t); } Now, create a class ListProcessor. It is a helper class that acts as a container for four static methods: destructiveFilter (ArrayList a, Predicate p) nondestructive Filter (ArrayList a, Predicate p) nondestructive FunctionApplyer (ArrayList a, Function f) destructive FunctionApplyer (ArrayList a, Function f) All four of these static methods take an ArrayList of and either a Predicate or a Function , and all four of these methods should return an ArrayList of integers. The nondestructive methods shouldn't modify the list they're given; they should create a new ArrayList with the correct elements and return that. The destructive methods, on the other hand, should actually change the ArrayList they're given, and then return them. The filter methods should apply the Predicate they're given to each element in the list, and whether the Predicate returns true or false determines whether that element will be in the list that's returned. The functionApplyer methods should apply the Function they're given to each element in the list, and put each result at the same index in the list. What your Tester.java main method should do: Create ArrayLists of at least two different type parameters one of which is Integer, fill them, and test each of the four helper methods on them. 1. Test and print the input and output of destructiveFilter and nondestructive Filter on test lists for a particular Predicate. Use a lambda expression for the Predicate! 2. Do the above again, but with a different Predicate. Again, use a lambda expression for the Predicate! 3. Test and print the input and output of destructive FunctionApplyer and nondestructive FunctionApplyer on test lists for a particular Function. Use a lambda expression for the Function! 4. Do the above again, but with a different Function. And again, use a lambda expression for the Function. Example output is shown below: Tester: intlist: [393, 974, 79, 38, 793, 96, 318, 514, 962, 479] intlist non-destructively filtered to remove odds: [974, 38, 96, 318, 514, 962] intlist (should be unchanged): [393, 974, 79, 38, 793, 96, 318, 514, 962, 479] intlist destructively filtered to remove odds... intList: [974, 38, 96, 318, 514, 962] [974, 38, 96, 318, 514, 962] strlist2: [California, Utah, New York, Idaho, Nevada, Wyoming] strlist2 non-destructively filtered to remove elements containing 'a': [New York, Wyoming] strlist2 (should be unchanged): [California, Utah, New York, Idaho, Nevada, Wyoming] strlist destructively filtered to remove elements containing 'a'... strlist2: [New York, Wyoming] intList4: [5, 6, 7, 8, 9] intlist4 non-destructively made all values decremented: [4, 5, 6, 7, 8] intList4 (should be unchanged): [5, 6, 7, 8, 9] intlist4 destructively made all values decremented... intList4: [4, 5, 6, 7, 8] strlist: [cat, dog, house, zebra, Constantinople] strlist non-destructively made all values truncated by one char: [ca, do, hous, zebr, Constantinopl] strlist (should be unchanged): [cat, dog, house, zebra, Constantinople] strlist destructively made all values truncated by one char... strlist: [ca, do, hous, zebr, Constantinopll import java.util.ArrayList; import java.util.ListIterator; public class List Processor { public T a; public T p; public T f; public ListProcessor (T ArrayList, T Predicate, T Function) { ArrayList = a; Predicate = p; Function = f; } public void setArrayList (Tn){ this.a = n; } public void setPredicate (T n) { this.p = n; } public void setFunction (Tn){ this. f = n; } public T getArrayList() { return a; } public T getPredicate() { return p; } public T getFunction () { return f; } public String toString(){ return " < >"; public static ArrayList //Iterator ListIterator iterator = a.listIterator(); while(iterator.hasNext()) } // if item doesn't pass test, remove it) if (!p.test(iterator.next())) iterator.remove(); return a; public static ArrayList nondestructiveFilter(ArrayList a, Predicate p) { ArrayList result = new ArrayList (); //Iterator ListIterator iterator = a.listIterator(); while(iterator.hasNext()) { destructiveFilter(ArrayList a, Predicate p) { T item iterator.next(); // if item pass if (p.test(item)) result.add(item); } return result; public static ArrayList nondestructiveFunctionApplyer (ArrayList a, Function f) { ArrayList result = new ArrayList (); //iterator ListIterator iterator = a.listIterator(); while(iterator.hasNext()){ // add result of function to the result list result.add(f.apply(iterator.next())); } return result; public static ArrayList destructiveFunctionApplyer (ArrayList a, Function f) { // iterator ListIterator iterator = a.listIterator(); while(iterator.hasNext()){ //set the current item to the result of the function iterator.set(f.apply(iterator.next())); } return a; @FunctionalInterface public interface Function { T apply (T nexT); } @FunctionalInterface public interface Predicate { boolean test (T Next); } import java.util.ArrayList; public class Tester { public static void main(String[] args) { System.out.println("Tester: "); //Random list of 10 times ArrayList intlist= new ArrayList (); for (int i = 0; i
Step by Step Solution
There are 3 Steps involved in it
Step: 1
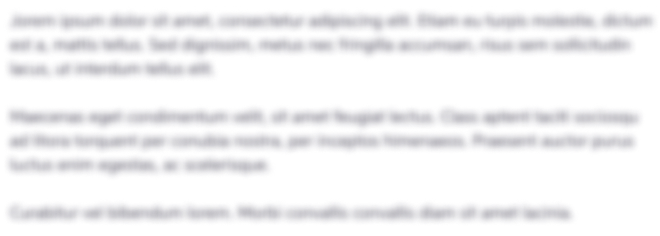
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started