Question
Ghost Primer In this assignment, you will implement a series of functions and classes you will need for the upcoming Ghost project. Please do your
Ghost Primer
In this assignment, you will implement a series of functions and classes you will need for the upcoming Ghost project. Please do your best job on this assignment as early as possible. As you will depend on the code in this assignment in the ghost project.
You will be given two interfaces and two abstract classes, FileTextReader, FileTextWriter, AbstractFileMonitor, and AbstractDictionary. Your job is to create two classes the first class should be named FileManager, the second class should be named Dictionary. The FileManager will implement the interfaces FileTextReader and FileTextWriter and extend the class AbstractFileMonitor. Your class signature would look something like the following:
public class FileManager extends AbstractFileMonitor implements FileTextReader, FileTextWriter{... The constructor signature of the FileManager should look like the following:
public FileManager(String filePath){... The Dictionary will extend the abstract class AbstractDictionary. Your class signature would look something like the following:
public class Dictionary extends AbstractDictionary {... The constructor signature of the Dictionary should look like the following:
public Dictionary(String path, FileManager fileManager) throws IOException {... Please read the programmatic documentation of the two interfaces and abstract classes to gain an understanding of what each function should do. This assignment will test your knowledge of reading and writing to a text file, implementing interfaces, extending abstract classes, throwing exceptions and working with collections specifically sets.
This project is accompanied by two test classes, ManagerTest, and DictionaryTest. They will test the functionality of the functions in the interfaces and the abstract methods of classes. Its recommended that you implement the FileManager before working on the AbstractDictionary.
AbstractDictionary
package homework;
import java.io.IOException; import java.util.Objects; import java.util.Set;
/** * A class that holds a collection of words read from a text file. The collection of words is used in the methods of this class * methods provided in this class. * @author Harris Williams * @since Sep 28, 2018 */ public abstract class AbstractDictionary { private final FileTextReader FILE_READER; private final Set
FILE_READER = dictionaryFileReader;
ALL_WORDS = FILE_READER.getAllLines(path); } /** * Returns a set of all the words contained in the dictionary text file. * @return a set containing all the words in the dictionary file. */ public Set
/** * Counts the number of words in this Dictionary that start with the specified prefix and have a length that is equal or greater * than the specified size. If size the specified size is less than 1 then word size is not taken into account. * @param prefix the prefix to be found * @param size the length that the word must equal or be greater than. If a value less than 1 is specified, all words regardless of their * characters size should be considered. In other words if the size parameter is < 1 word size is disregarded in the calculations. * @param ignoreCase if true this will ignore case differences when matching the strings. If false this considers * case differences when matching the strings * @return The number of words that start with the specified prefix * @throws IllegalArgumentException if the specified string is null or empty (Meaning contains no characters or only white space or blank) */ public abstract int countWordsThatStartWith(String prefix, int size, boolean ignoreCase) throws IllegalArgumentException;
/** * Tests if this Dictionary contains at least one word with a length equal to or greater than the specified size that starts with the specified prefix. * If size the specified size is less than 1 then word size is not taken into account. * @param prefix the prefix to be found * @param size the length that the word must equal or be greater than. If a value less than 1 is specified, all words regardless of their * characters size should be considered. In other words if the size parameter is < 1 word size is disregarded in the calculations. * @param ignoreCase if true this will ignore case differences when matching the strings. If false this considers * case differences when matching the strings * @return The number of words that start with the specified prefix * @throws IllegalArgumentException if the specified string is null or empty (Meaning contains no characters or only white space) */ public abstract boolean containsWordsThatStartWith(String prefix, int size, boolean ignoreCase) throws IllegalArgumentException;
/** * Returns a set of all the words within in this Dictionary that start with the specified prefix and have a length that is equal or greater * than the specified size. If size the specified size is less than 1 then word size is not taken into account. * @param prefix the prefix to be found * @param size the length that the word must equal or be greater than. If a value less than 1 is specified, all words regardless of their * characters size should be considered. In other words if the size parameter is < 1 word size is disregarded in the calculations. * @param ignoreCase if true this will ignore case differences when matching the strings. If false this considers * case differences when matching the strings * @return A list of all strings that start with the specified prefix * @throws IllegalArgumentException if the specified string is null or empty (Meaning contains no characters or only white space) */ public abstract Set
package homework;
import java.io.File; import java.io.FileInputStream; import java.io.IOException; import java.security.DigestInputStream; import java.security.MessageDigest; import java.security.NoSuchAlgorithmException;
/** * A class with methods to monitor a text file. * @author Harris Williams * @since Sep 28, 2018 */ public abstract class AbstractFileMonitor {
private MessageDigest messageDigest; private int currentCheckSum; private boolean hasChanged = false; private long nextUpateTime = 0; public AbstractFileMonitor(String path){ setFilePath(path); } /** * Updates the variables that correspond to the file being monitored * * * This function has been throttled such that it will only update values every 250 ms. In other words successive calls to this * function in time intervals that are less than 250 ms will yield no change. * @throws IOException Thrown if any type of I/O exception occurs while writing to the file * @throws IllegalStateException If the {@link #setFile(String)} method in not invoked with a proper file prior to invocation of this * @throws NoSuchAlgorithmException If the computer's OS is missing the SHA-256 hashing algorithm * method. In other words if no file is currently set to be monitored. */ public void update() throws IOException, IllegalStateException, NoSuchAlgorithmException {
if(messageDigest == null){ messageDigest = MessageDigest.getInstance("SHA-256"); } if(nextUpateTime > System.currentTimeMillis()) { hasChanged = false; return; } nextUpateTime = System.currentTimeMillis() + 250; File file = new File(getFilePath()); if(!file.exists()) return; try (DigestInputStream dis = new DigestInputStream(new FileInputStream(getFilePath()), messageDigest)) { while (dis.read() != -1) ; messageDigest = dis.getMessageDigest(); }
StringBuilder result = new StringBuilder(); for (byte b : messageDigest.digest()) { result.append(String.format("%02x", b)); } hasChanged = currentCheckSum != result.toString().hashCode(); currentCheckSum = result.toString().hashCode(); }
/** * Tests if the file being monitored has changed since the last time {@link #update()} was invoked. * @return true if and only if this monitor deems this file has changed. This will return false if the {@link #update()} method is not * invoked prior to invocation of this method */ public boolean hasChanged(){ return hasChanged; } /** * Sets the path of the file to be monitored. * @param path the location of the file to be monitored * @throws IllegalArgumentException if path is null, empty or blank. */ public abstract void setFilePath(String path);
/** * Get the path of the file that is monitored * @return path the location of the file that is monitored * @throws IllegalStateException if {@link #setFile(String)} is not invoked prior to calling this method. */ public abstract String getFilePath() throws IllegalStateException; } FileTextReader.java
package homework;
import java.io.IOException; import java.util.Collection; import java.util.List; import java.util.Set;
/** * A class with methods specifically for reading from text files * @author Harris Williams * @since Sep 28, 2018 */ public interface FileTextReader{ /** * Reads all the text in text file at the specified path. * @param path the location of a file that the text will be read * @return A string of all the text contained in the specified text file * @throws IOException Thrown if any type of I/O exception occurs while writing to the file */ public String readText(String path) throws IOException; /** * Reads all the lines of the text file at the specified path. Adds each line of the text to the a collection and * returns that collection. * * Each line in the collection should have all the leading and trailing white space characters removed prior to * adding it to the collection. See the trim() method in the String class as an alternative. * * @param path the location of a file that the text will be read * @return A collection of all the lines of text contained in the specified text file * @throws IOException Thrown if any type of I/O exception occurs while writing to the file */ public Set
package homework;
import java.io.IOException;
/** * A class with methods specifically for reading from text files * @author Harris Williams * @since Sep 28, 2018 */ public interface FileTextWriter {
/** * Writes the specified string to a file at the specified path. It creates a new line in the file then writes the string * to the file. It has the same result of calling System.out.println(String) has with the console but in this case its for a * file. * @param string the text that will be written to the file * @param path the location of a file that the string will be written to * @throws IOException Thrown if any type of I/O exception occurs while writing to the file * @throws IllegalArgumentException if the specified string is null or empty (Meaning contains no characters or only white space) */ public void writeToFile(String string, String path) throws IOException, IllegalArgumentException; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
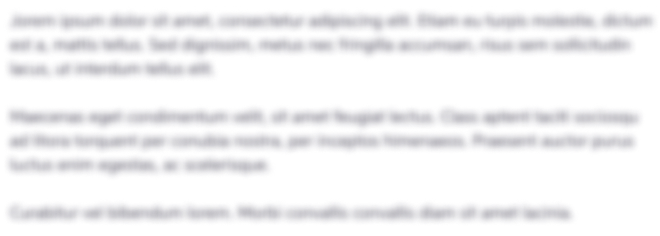
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started