Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Given a file of unsorted words with mixed case: read the entries in the file and sort those words into alphabetical order. The program should
Given a file of unsorted words with mixed case: read the entries in the file and sort those words into alphabetical order. The program should then prompt the user for an index, and display the word at that index. Since you must store the entire list in an array, you will need to know the length. The "List of Mixed Case Words" contains words. Note that some of these "words" have spaces, so read them one line at a time.
You are guaranteed that the words in the array are unique, so you don't have to worry about the order of say, "bat" and "Bat."
For example, if the array contains ten words and the contents are
cat Rat bat Mat SAT vat Hat pat TAT eat
the sorted list is
bat cat eat Hat Mat pat Rat SAT TAT vat
and after sorting, the word at index is Rat
You are encouraged to use this data to test your program.
The code I have is saying exception thrown at line and the Int Main is underlined in green saying that the amount of bytes is too large. How do I fix this?
#include
#include
#include
#include
#include
#include
#include Include the vector library
using namespace std;
bool isUniquestring words int cnt string word;
void sortstring words int cnt;
int main
const int SIZE ;
string wordsSIZE;
string word;
int count index;
ifstream dataIn;
dataIn.openMixedCaseWordstxt;
if dataInfail
cout File Not Found ;
return ;
else
while dataIn word
bool b isUniquewords count, word;
if b
wordscount word;
count;
dataIn.close;
sortwords count;
while true
cout "Enter Index :;
cin index;
if index indexcount
cout Invalid.Must be between count endl;
else
break;
cout "After sorting, the word at index index is wordsindex endl;
return ;
bool isUniquestring words int cnt string word
string str str;
for int k ; k word.length; k
str tolowerwordatk;
for int i ; i cnt; i
str;
for int j ; j wordsilength; j
str tolowerwordsiatj;
if strcomparestr
return true;
return false;
void sortstring array int cnt
string temp, mixedTemp;
string str;
vector lowercnt; Use a vector instead of an array
for int m ; m cnt; m
for int s ; s arraymlength; s
str tolowerarraymats;
lowerm str;
str;
for int i ; i cnt; i
for int j i ; j cnt; j
if lowericomparelowerj
temp loweri;
loweri lowerj;
lowerj temp;
mixedTemp arrayi;
arrayi arrayj;
arrayj mixedTemp;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
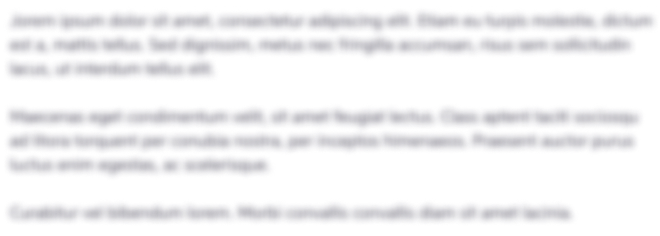
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started