Question
Given a program (Test.java) that gets two numbers from user input and then carries out a division, revise it to handle exceptional inputs. The given
Given a program (Test.java) that gets two numbers from user input and then carries out a division, revise it to handle exceptional inputs. The given program does those:
Prompt 1
Input 1
Prompt 2
Input 2
Calculation
For the purpose of this project, input 1 should be a double value and input 2 should be a non-zero int value. Upon an invalid input, your program should allow a user to re-enter a piece of data. This would require a loop to validate each input and allow for additional data entry. We need a loop instead of an if-else as you cant assume a second input would be valid.
You will complete this exercise using:
- Use defensive coding.
Version B: Defensive Coding
You need to use the hasNextXXX methods of Scanner class for the defensive coding version.
public boolean hasNextDouble()
public boolean hasNextInt()
A hasNextXXX method peeks (does NOT read) into user input to decide if the next string to read contains a valid XXX. Use Java API 8 documentation if needed. The following snippet of code illustrates how hasNextXXX may be used:
if (stdIn.hasNextDouble()) { //check before read
dividend = stdIn.nextDouble();
}
else {// no valid input
stdIn.next(); // read string and discard
System.out.println("Invalid dividend");
isValid = false;
}
Follow this pseudo code for defensive coding version:
Do // first num
isValid true
Prompt 1
If has double input
Input 1: nextDouble()
Else
extract input: next()
display err msg
isValid false
End If
While !isValid
Do // second num
isValid true
Prompt 2
If has int input
Input 2: nextInt()
If zero
display err msg
isValid false
End If
Else
extract input: next()
display err msg
isValid false
End If
While !isValid
calculation
This version may work like this:
Enter dividend: 4.2.3
Invalid dividend
Enter dividend: 4/5
Invalid dividend
Enter dividend: 4.5
Enter divisor: 1.5
Invalid divisor
Enter divisor: 0
Input error: divisor can't be zero. Please input again.
Enter divisor: 3
4.5 / 3 = 1.5
/** * Test.java * * If input is of wrong format (InputMismatchException), or * if 0 is provided for divisor, keep asking until valid * data is provided. */
import java.util.Scanner;
public class Test { public static void main(String[] args) { Scanner stdIn = new Scanner(System.in); double dividend; int divisor;
// input System.out.print("Enter dividend: "); dividend = stdIn.nextDouble();
System.out.print("Enter divisor: "); divisor = stdIn.nextInt();
stdIn.close();
// process and result System.out.println(dividend + " / " + divisor + " = " + dividend / divisor); } // end main
} // end Test class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
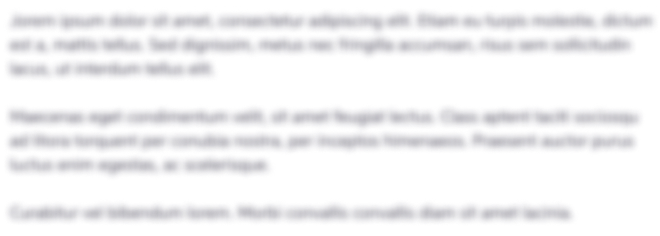
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started