Question
Given the CoffeeDrink class defined by two Ingredient objects contained in it (coffee and milk) as well as the Ingredient class representing an ingredient in
- Given the CoffeeDrink class defined by two Ingredient objects contained in it (coffee and milk) as well as the Ingredient class representing an ingredient in terms of its name and its strength. Strength is an integer between 1-5, 5 being the highest strength. The lower the ratio of milk strength to coffee strength, the stronger the coffee drink.
Implement a method testCoffeeDrinks that takes one parameter: - drinks, an non-empty array of CoffeeDrink objects The method finds and prints to the screen the strongest drink (You must use the compareTo method for full credit). If more than one such drink occur in the array, pick the first one. The method also creates, populates and returns an array of integers containing the frequency of each possible coffee strength (1-5), in the drinks array. For example, if the information of the drinks in the array is given in the table below:
Coffee drink num | Ingredient 1 | Ingredient 2 |
0 | Coffee, 4 | Milk, 3 |
1 | Coffee, 2 | Milk, 3 |
2 | Coffee, 2 | Milk, 1 |
3 | Coffee, 4 | Milk, 1 |
4 | Coffee, 3 | Milk, 2 |
5 | Coffee, 4 | Milk, 4 |
The program will display:
coffee strength:4
milk strength: 1
And return the array {0,2,1,3,0}
Given:
public class Ingredient{
private String name;
private int strength;
public Ingredient(String name, int strength) {
this.name=name; this.strength=strength;
}
public int getStrength()
{
return strength;
}
public String toString() {return name+" strength: "+strength;}
}
public class CoffeeDrink
{
private Ingredient coffee, milk;
public CoffeeDrink(Ingredient i1, Ingredient i2)
{
this.coffee=i1;
this.milk=i2;
}
public Ingredient getIngred1()
{
return coffee;
}
public Ingredient getIngred2()
{
return milk;
}
// compares two drinks based on their milk/coffee ratio.
//drink 1 is stronger than drink 2 if that ratio is smaller than
// the corresponding ratio of drink 2
public int compareTo(CoffeeDrink other)
{
if ((double)milk.getStrength()/coffee.getStrength() > (double) other.getIngred2().getStrength()/other.getIngred1().getStrength())
return 1;
else if ((double)milk.getStrength()/coffee.getStrength() < (double) other.getIngred2().getStrength()/other.getIngred1().getStrength())
return -1;
else return 0;
}
public String toString() { return coffee.toString()+" "+milk.toString();}
}
Step by Step Solution
3.53 Rating (160 Votes )
There are 3 Steps involved in it
Step: 1
Heres the implementation of the testCoffeeDrinks method Java public static int testCoffeeDrinksCoffeeDrink drinks Find the strongest drink CoffeeDrink strongestDrink drinks0 for int i 1 i drinkslength ...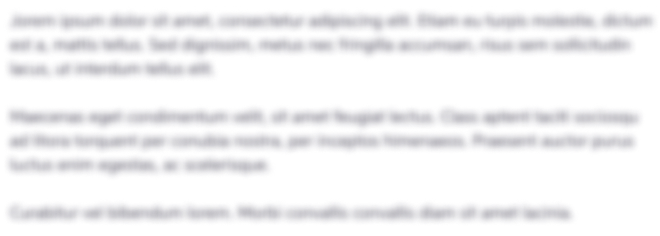
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started