Question
Given the following code, could someone help me to implement some extra functionality: // DrawingGUI.java import javafx.application.Application; import static javafx.application.Application.launch; import javafx.beans.value.ChangeListener; import javafx.beans.value.ObservableValue; import
Given the following code, could someone help me to implement some extra functionality:
// DrawingGUI.java
import javafx.application.Application;
import static javafx.application.Application.launch;
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ComboBox;
import javafx.scene.input.MouseEvent;
import javafx.scene.layout.HBox;
import javafx.scene.layout.Pane;
import javafx.scene.layout.VBox;
import javafx.scene.paint.Color;
import javafx.scene.shape.Line;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class DrawingGUI extends Application {
//array of color names for listing in combobox
final String colors[] = {"Red", "Green", "Blue"};
//declaring UI elements
Pane drawingSpace;
boolean drawing = false;
double currentX, currentY;
Text penStatus;
ComboBox
Button clear;
Color selectedColor = Color.RED; //default color
@Override
public void start(Stage primaryStage) {
//defining all the controls
penStatus = new Text("Pen: Off");
penStatus.setFont(new Font(20));
colorBox = new ComboBox();
colorBox.getItems().addAll(colors);
colorBox.setValue("Red");
clear = new Button("Clear");
//adding controls into an HBox (horizontal) and aligning center
HBox hBox = new HBox(penStatus, colorBox, clear);
hBox.setSpacing(10);
hBox.setAlignment(Pos.CENTER);
//creating a pane for drawing area
drawingSpace = new Pane();
drawingSpace.setMinSize(500, 500);
//creating a Vbox to arrange hbox and drawing area vertically
VBox box = new VBox(hBox, drawingSpace);
Pane root = new Pane(box);
//adding mouse event listeners
drawingSpace.addEventFilter(MouseEvent.MOUSE_CLICKED, e -> {
//changing drawing to false if true, true if false
if (drawing) {
drawing = false;
penStatus.setText("Pen: Off");
} else {
drawing = true;
currentX = e.getX();
currentY = e.getY();
penStatus.setText("Pen: On");
}
});
drawingSpace.addEventFilter(MouseEvent.MOUSE_MOVED, e -> {
//if drawing, creating a line from previous point to the new point
if (drawing) {
double x = e.getX();
double y = e.getY();
Line line = new Line(currentX, currentY, x, y);
line.setFill(selectedColor);
line.setStroke(selectedColor);
//adding to the drawing space
drawingSpace.getChildren().add(line);
currentX = x;
currentY = y;
}
});
//adding change listener for color combo box
colorBox.valueProperty().addListener(new ChangeListener
@Override
public void changed(ObservableValue ov, String t, String t1) {
//updating selected color
if (colorBox.getValue().equalsIgnoreCase("Red")) {
selectedColor = Color.RED;
} else if (colorBox.getValue().equalsIgnoreCase("Green")) {
selectedColor = Color.GREEN;
} else {
selectedColor = Color.BLUE;
}
}
});
//adding action listenr for resetting drawing space
clear.setOnAction(e -> {
drawingSpace.getChildren().clear();
});
Scene scene = new Scene(root, 500, 500);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
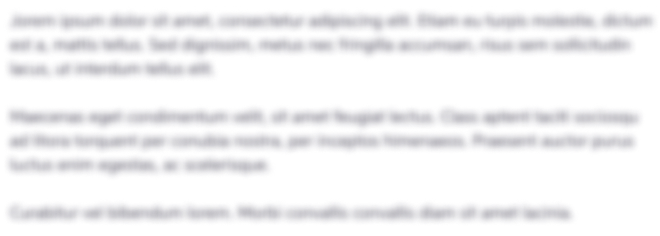
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started