Question
Given the following IntTree class, I am wondering if the implementations of the preorder, postorder and level order methods are correct, and if not what
Given the following IntTree class, I am wondering if the implementations of the preorder, postorder and level order methods are correct, and if not what should be changed in them.
Would like to keep the 3 constructors the same if possible.
import java.io.*;
import java.util.*;
public class IntTree {
private class Node {
private int data;
private Node firstChild;
private Node sibling;
private Node parent;
private Node (int d, Node f, Node s, Node p) {
data = d;
firstChild = f;
sibling = s;
parent = p;
}
}
private Node root;
public IntTree(int d) {
//create a one node tree
root = addNode(root, d);
}
public IntTree(IntTree t[], int d) {
//create a new tree whose children are the trees in t and whose root value is d
Node newTree = new Node(d, t[d].root.firstChild, t[d].root.sibling, t[d].root.parent);
}
public IntTree(int d[]) {
//create a tree with d[0] as the root value and the other values as children of the root
for(int i = 0; i < d.length; i++) {
addNode(root, d[i]);
}
}
private Node addNode(Node currNode, int value) {
//Add a Node to the tree
if(currNode == null) {
return new Node(value, root, null, null);
}
if(value < currNode.data) {
currNode.firstChild = addNode(currNode.firstChild, value);
}
else if(value > currNode.data) {
currNode.sibling = addNode(currNode.sibling, value);
}
else {
return currNode;
}
return currNode;
}
public String preorder() {
//return a string of the ints in the tree in preorder
//separate the ints with commas
//the implementation must be recursive
return preOrder(root);
}
private String preOrder(Node r) {
String s = "";
if(r != null) {
preOrder(r.parent);
preOrder(r.firstChild);
preOrder(r.sibling);
s = r.data + "" + ",";
}
return s;
}
public String postorder() {
//return a string of the ints in the tree in postorder
//separate the ints with commas
//the implementation must be recursive
return postOrder(root);
}
private String postOrder(Node r) {
String s = "";
if(r != null) {
postOrder(r.firstChild);
postOrder(r.sibling);
postOrder(r.parent);
s = r.data + "" + ",";
}
return s;
}
public String levelorder() {
//return a string of the ints in the tree in level order (also know a breadth first order)
//separate the ints with commas
//the implementation must be iterative
return printLevelOrder();
}
private String printLevelOrder() {
LinkedList
nodeList.add(root);
String s = "";
while(nodeList.size() > 0) {
Node r = nodeList.remove();
if(r.parent != null) {
nodeList.add(r.parent);
}
if(r.firstChild != null) {
nodeList.add(r.firstChild);
}
if(r.sibling != null) {
nodeList.add(r.sibling);
}
s = r.data + "" + ",";
}
return s;
}
}
Thanks for your help!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
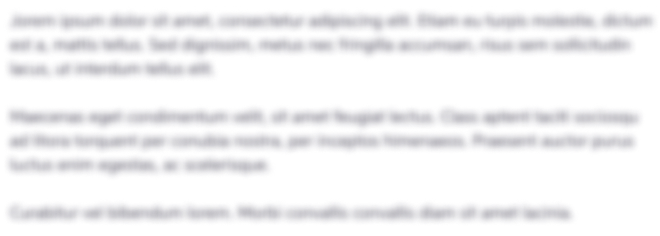
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started