Question
Given three numbers, create a class Area with following characteristics. Must extend Rectangle and Square class. Public function named getSumOfAreas that accept two parameter of
Given three numbers, create a class Area with following characteristics.
- Must extend Rectangle and Square class.
- Public function named getSumOfAreas that accept two parameter of double type and return addition of those number.
Note: Rectangle and Square class already created.
Input
5.5
5.5
6.5
Where,
- The first line is length of Rectangle.
- The second line is width of Rectangle.
- The third line is side of Square.
Output
30.25
42.25
72.50
Where,
- The first line of output contains area of Rectangle.
- The second line of output contains area of Square.
- The third line of output contains sum of above area.
Assume that,
- Inputs are in double within the range [ 1 to 10^20].
Not sure where to begin, please use code template below:
#include
#include
using namespace std;
//rectangle class
class Rectangle {
public:
double areaOfRectangle(double l, double w) {
if (l > 0 && w > 0)
return l * w;
else
return 0;
}
};
// Square class
class Square {
public:
double areaOfSquare(double s) {
if (s > 0)
return s * s;
else
return 0;
}
};
like cin/cout.
//write your code here
int main() {
double l,w,s;
Area area;
cin >> l;
cin >> w;
cin >> s;
cout << fixed << setprecision(2) << area.areaOfRectangle(l,w) << endl;
cout << fixed << setprecision(2) << area.areaOfSquare(s) << endl;
cout << fixed << setprecision(2) << area.getSumOfAreas(area.areaOfRectangle(l,w),area.areaOfSquare(s));
return 0;
}
Step by Step Solution
3.47 Rating (163 Votes )
There are 3 Steps involved in it
Step: 1
include include using namespace std rectangle class class Rectangle pub...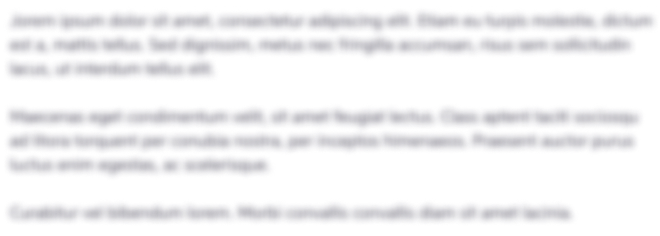
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started