Question
GNG1106 Lab 5A) I need help finding three bugs in this program. I only found one online 59 (&), but I'm having trouble finding the
GNG1106 Lab 5A)
I need help finding three bugs in this program.
I only found one online 59 (&), but I'm having trouble finding the other two.
---------------------------------------------------------------------------------------------------
/*------------------------------------------------------------------ File: cylinderVolume.c (Lab 5) Description: Calculates how the volume changes w.r.t the depth of a liquid in a cylinder. ------------------------------------------------------------------*/ #include #include // Define symbolic constant #define TRUE 1 #define FALSE 0 #define NUM_VALUES 20 // Number of time/velocity values to display #define NUM_ROWS 2 // Number of rows in 2D array - one for depth and one for volume #define D_IX 0 // Index for elements containing depth values (row 0) #define V_IX 1 // Index for elements containing volume values (row 0) // Definition of a structure type for user input typedef struct { char orientation; // h for horizontal and v for vertical double radius; // in m double length; // in m } CYLINDER; // Prototypes void getInput(CYLINDER *); double getPositiveValue(char *); void fillArray(CYLINDER *, int n, double[][n]); double computeVolume(CYLINDER *, double); void displayTable(CYLINDER *, int n, double *, double *); /*-------------------------------------------------------------------- Function: main Description: Gets from the user values for the radius and length of the cylinder as well as it orientation. Fills an array with depth/volume values and then displays in a table these values. ----------------------------------------------------------------------*/ int main() { // Variable declarations CYLINDER cyl; // structure variable fo cylinder // Note in the following 2D array // points[D_IX] is a 1D array that contains the depth values // points[V_IX] is a 1D array that contains the volume values double values[NUM_ROWS][NUM_VALUES]; // NUM_VALUES depth/volume value pair // Get user input getInput(&cyl); //the error was that there was no "&" // Fill in the array with depth/volume values fillArray(&cyl, NUM_VALUES, values); // Display table on console displayTable(&cyl, NUM_VALUES, values[D_IX], values[V_IX]); return(0); } /*----------------------------------------------------------------------- Function: getOrientation Description: Requests from the user the orientation of the cylinder: v for vertical and h for horizontal ------------------------------------------------------------------------*/ void getInput(CYLINDER *cylPtr) { int flag; // Get the cylinder radius and length cylPtr.radius = getPositiveValue("Please enter the value for the cylinder radius: "); cylPtr->length = getPositiveValue("Please enter the value for the cylinder length: "); // Get the orientation of the cylinder do { flag = FALSE; printf("Give the cylinder's orientation, v for vertical, h for horizontal: "); fflush(stdin); scanf("%c", &cylPtr->orientation); if(cylPtr->orientation != 'v' && cylPtr->orientation != 'h') { printf("Bad input. "); flag = TRUE; } } while(flag); } /*------------------------------------------------------------------------ Function: getPositiveValue Parameter: prompt: reference to a prompt message to the user Returns: A value strictly positive (>0) Description: Prompts for and reads a real value from the user, checks that it is strickly positive, and returns the value. ------------------------------------------------------------------------*/ double getPositiveValue(char *prompt) { double value; do { printf(&prompt); scanf("%lf",&value); if(value orientation == 'v') inc = cyl->length/(NUM_VALUES-1); else inc = cyl->radius/(NUM_VALUES-1); for(cix = 0; cix orientation == 'v') vol = M_PI*cyl->radius*cyl->radius*depth; else { // Utilise plusieurs intructions pour faire le calcule // Les valeurs intermdiaires sont accumules dans vol vol = sqrt(2*cyl->radius*depth - depth*depth); vol = (cyl->radius - depth)*vol; vol = (pow(cyl->radius,2)*acos((cyl->radius - depth)/cyl->radius)) - vol; vol = vol*cyl->length; } return(vol); } /*----------------------------------------------------------------------- Function: displayTable Parameters: cyl - pointer to a variable structure that gives the cylinder characteristics. int n - nnumber of elements in the referenced arrays depth - pointer to an array that contains the depth values volume - pointer to an array that contains the volume values Description: Displays the characteristics of the cylinder followed by a table that shows how the volume varies the depth of a liquide in the cylinder. The data is given in the arrays referenced by the parameters. ------------------------------------------------------------------------*/ void displayTable(CYLINDER *cyl, int n, double *depth, double *volume) { // Declaration of variables int ix; // Index into arrays. // Display results printf(" The change in liquid volume of the cylinder with radius %.2f and length %.2f as depth changes when ", cyl->radius, cyl->length); if(cyl->orientation == 'v') printf("vertical "); else printf("horizontal "); printf("%10s %10s ","Depth", "Volume"); printf("------------------------ "); for(ix = 1; ix A. Exercise: Volume of a Horizontal Cylinder - Using arrays with functions (5 points) Another version of the cylinder problem has been provided in Cylinder VolumeLab5.zip (contains a CodeBlocks project). In this new version pointers are used in functions to receive references (i.e. addresses) to structures and/or arrays. This time there are 3 syntax errors and 2 bugs in this program that you need to discover; one of the bugs causes the program to crash. Recall that the volume of a liquid in a hollow vertical cylinder with radius r and length L is related to the depth of the liquid, h, as V =arh Recall that the volume of a liquid in a hollow horizontal cylinder with radius r and length L is related to the depth of the liquid, h, as v = r2 arccos ("") ----)/2rh="4 The following tables provides a test cases that can be used for testing the software (this table was generated using Microsoft Excel, see the file GNGI 106Lab5Test Cases.xls; note that the Excel file also contains values for each of the terms in the above equation to help with debugging. The volumes were computed using the above equations. The following table gives a test case (with radius = 4.5 m and length = 11.45 m for a vertical cylinder) for testing the program. Depth Volume 0.000 0.603 38.33776 1.205 76.67553 1.808 115.0133 2.411 153.3511 3.013 191.6888 3.616 230.0266 4.218 268.3644 4.821 306.7021 5.424 345.0399 6.026 383.3776 6.629 421.7154 7.232 460.0532 7.834498.3909 8.437536.7287 9.039575.0665 9.642 613.4042 10.245 651.742 10.847 690.0798 11.450728.4175 The following table provides a test case (with radius = 2.1 m and length = 5.6 m for horizontal cylinder). Volume r-h)/2rh-h Depth (m) /2rh-h (r-h 2rh-h (m) 0.000000 0.000000 0.000000 0.000000 0.000 0.110526 0.672305 1.337534 0.099610 0.558 0.221053 0.937847 1.762165 0.279465 1.565 0.331579 1.132558 2.002839 0.509191 2.851 0.442105 1.288947 2.136939 0.777389 4.353 0.552632 1.419736 2.196855 1.077168 6.032 0.663158 1.531498 2.200520 1.403666 7.861 0.773684 1.628154 2.159447 1.753142 9.818 0.884211 1.712266 2.081755 2.122559 11.886 0.994737 1.785607 1.973566 2.509351 14.052 1.105263 1.849459 1.839725 2.911287 16.303 1.215789 1.904776 1.684223 3.326378 18.628 1.326316 1.952284 1.510451 3.752823 21.016 1.4368421.992541 1.321370 4.188959 23.458 1.547368 2.025981 1.119621 4.633234 25.946 1.657895 2.052935 0.907613 5.084179 28.471 1.7684212.073657 0.687581 5.540389 31.026 1.8789472.088333 0.461632 6.000508 33.603 1.989474 2.097089 0.231784 .463216 36.194 2.100000 2.100000 0.000000 6.927212 38.792 The following are some features used in this program. In the main function The function main contains only the declaration of on structure variable and a 2D array as well as three calls to functions. o The called functions receive references (addresses) of the structure variable, the 2D array, and the rows of the 2D array. Thus the called functions will work directly with the variables in the working memory of main using these references. Note that the ampersand (&) is used only with the name of the structure variable; it is not used with the names of arrays. In C, arrays are passed by reference to functions and the & operator is not required. o Note in particular the call to the function display Table. The last 2 arguments, values [D_IX] and values [V_IX], are treated as single dimensional arrays (the 2D array rows). Thus the address of each row of the 2D array is passed to the function in each of these arguments. In the function getInput O Examine how the operator -> is used with the pointer to the structure variable. Note the call to get PositiveValue. In this version the prompt message is give as the argument in the function call. Recall that it is the address of the string that is sent to the function get PositiveValue. This function writes values directly into the variable referenced by the pointer cylPtr. Thus the function writes into the working memory of main. Note that the expression "cylPtr->orientation" is treated as a variable of type char. Thus the ampersand (&) operator is required with this expression in the call to scanf
Step by Step Solution
There are 3 Steps involved in it
Step: 1
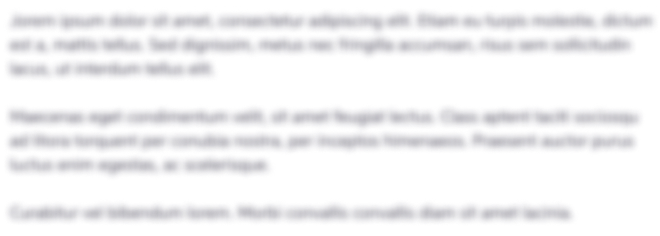
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started