Question
Go to this link to view full lab: https://github.com/Seneca-244200/OOP-Workshops/tree/main/WS07v Workshop #7: Derived Classes & Custom I/O Operators Lab (part 1) VehicleBasic Module Design and code
Go to this link to view full lab:
https://github.com/Seneca-244200/OOP-Workshops/tree/main/WS07v
Workshop #7: Derived Classes & Custom I/O Operators
Lab (part 1)
VehicleBasic Module
Design and code a class named VehicleBasic that holds information about a vehicle with an engine. Place your class definition in a header file named VehicleBasic.h and your function definitions in an implementation file named VehicleBasic.cpp.
Include in your solution all of the statements necessary for your code to compile under a standard C++ compiler and within the sdds namespace.
VehicleBasic Class
Design and code a class named VehicleBasic that holds information about a vehicle with an engine.
VehicleBasic Private Members
The class should be able to store the following data:
- a license plate number as a statically allocated array of characters of size 9.
- the address where the vehicle is at a given moment as a statically allocated array of characters of size 21.
- the year when the vehicle was built.
You can add any other private members to the class, as required by your design.
VehicleBasic Public Members
a custom constructor that receives as parameters the license plate number and the year when the vehicle was built. Set the location of the vehicle at Factory. Assume all data is valid.
void NewAddress(const char* address): moves the vehicle to the new address if the new address is different from the current address. Prints to the screen the message
|[LICENSE_PLATE]| |[CURRENT_ADDRESS] ---> [NEW_ADDRESS]|
where
- the license plate is a field of 8 characters aligned to the right
- current address is a field of 20 characters aligned to the right
- new address is a field of 20 characters aligned to left
ostream& write(ostream& os): a query that inserts into os the content of the object in the format
| [YEAR] | [PLATE] | [ADDRESS]
istream& read(istream& in): a mutator that reads from the stream in the data for the current object
Built year: [USER TYPES HERE] License plate: [USER TYPES HERE] Current location: [USER TYPES HERE]
Helper Functions
- overload the insertion and extraction operators to insert a VehicleBasic into a stream and extract a VehicleBasic from a stream. These operators should call the write/read member functions in the class VehicleBasic.
Dumper Module
Design and code a class named Dumper that holds information about a motor vehicle that can carry cargo. Place your class definition in a header file named Dumper.h and your function definitions in an implementation file named Dumper.cpp.
Include in your solution all of the statements necessary for your code to compile under a standard C++ compiler and within the sdds namespace.
Dumper Class
Design and code a class named Dumper that holds information about the basic vehicle that can carry cargo. This class should inherit from VehicleBasic class.
Dumper Private Members
The class should be able to store the following data (on top of data coming from the parent class):
- a capacity in kilograms as a floating-point number in double precision; this is the maximum weight of the cargo the Dumper can carry.
- the current cargo load (in kilograms) is a floating-point number in double precision; the load cannot exceed the capacity.
You can add any other private members in the class, as required by your design. Do not duplicate members from the base class!
Dumper Public Members
a custom constructor that receives as parameters the license plate number, the year when the Dumper was built, the capacity of the Dumper and the current address. Call the constructor from the base class and pass the license number and year to it. Set the capacity to the received value, the current cargo to 0, and move the Dumper to the address specified in the last parameter.
bool loaddCargo(double cargo): a mutator will verify if he can load the Cargo based on the cargo load weight specified as a parameter and the current cargo on the dumper and the capacity; ** all or none will be loaded **. ** If the current load has been changed, return true, otherwise, return false.
bool unloadCargo(): a mutator that unloads current cargo (sets the attribute to 0). If the current load has been changed, return true, otherwise, return false. ** the mutator will not make anything if the current cargo is 0 and thus return false **
ostream& write(ostream& os): a query that inserts into os the content of the object in the format
| [YEAR] | [PLATE] | [ADDRESS] | [CURRENT_CARGO]/[CAPACITY]
istream& read(istream& in): a mutator that reads from the stream in the data for the current object
Built year: [USER TYPES HERE] License plate: [USER TYPES HERE] Current location: [USER TYPES HERE] Capacity: [USER TYPES HERE] Cargo: [USER TYPES HERE]
Helper Functions
- overload the insertion and extraction operators to insert a Dumper into a stream and extract a Dumper from a stream. These operators should call the write/read member functions in the class Dumper.
w7_main Module (supplied)
Do not modify this module! Look at the code and make sure you understand it.
// Workshop 7: Inheritance // Version: 1.0 // Date: 2021-08-30 // Author: Wail Mardini ///////////////////////////////////////////// #include
Sample Output
---------------------------------------------------------- |> Vehicle 1: Basic Vehicle ---------------------------------------------------------- | 2010 | abc-123 | Factory | abc-123| | Factory ---> Downtown Toronto | | abc-123| | Downtown Toronto ---> Mississauga | | abc-123| | Mississauga ---> North York | | 2010 | abc-123 | North York ---------------------------------------------------------- |> Vehicle 2: Read/Write ---------------------------------------------------------- Built year: 2018 License plate: abc-123 Current location: Ottawa | 2018 | abc-123 | Ottawa ---------------------------------------------------------- |> Vehicle 3: Dumper ---------------------------------------------------------- | T-1111| | Factory ---> Toronto HQ | | T-1111| | Toronto HQ ---> Toronto Deposit | Cargo loaded! Status | 2015 | T-1111 | Toronto Deposit | 2345/5432 | T-1111| | Toronto Deposit ---> Ottawa | Cargo loaded! Status | 2015 | T-1111 | Ottawa | 2468/5432 | T-1111| | Ottawa ---> Montreal | Loading cargo failed! Status | 2015 | T-1111 | Montreal | 2468/5432 | T-1111| | Montreal ---> New York | Loading cargo failed! Status | 2015 | T-1111 | New York | 2468/5432 | T-1111| | New York ---> New Jersey | Cargo unloaded! Status | 2015 | T-1111 | New Jersey | 0/5432 | T-1111| | New Jersey ---> Toronto | Unloading cargo failed! Status | 2015 | T-1111 | Toronto | 0/5432 ---------------------------------------------------------- |> Vehicle 4: Read/Write ---------------------------------------------------------- Built year: 2020 License plate: dab-112 Current location: Montreal Capacity: 7000 Cargo: 0 | 2020 | dab-112 | Montreal | 2020 | dab-112 | Montreal | 0/7000
Step by Step Solution
There are 3 Steps involved in it
Step: 1
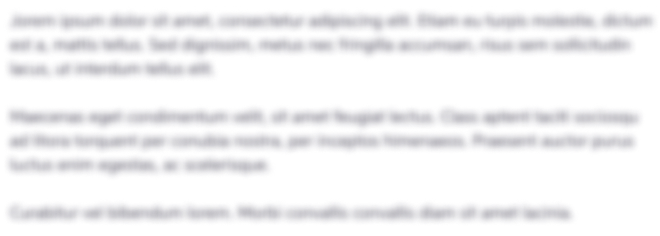
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started