Question
Goals: (A) Learn how to use array for storing objects (B) Learn how to use array for storing pointers (C) Compare the differences of these
Goals:
(A) Learn how to use array for storing objects
(B) Learn how to use array for storing pointers
(C) Compare the differences of these two methods
Requirements:
Follow the guidelines intertwined with the code to complete the works.
Write comments on the top of the program to answer these questions:
(1) Should we delete the data stored in studArr1? Why?
(2) Should we delete the data stored in studArr2? Why? When we delete the pointers, do we actually release the memory which was allocated for (1) the studArr2 array, (2) the pointer stored in the array, or (3) the student objects pointed by the pointers?
#include
using namespace std;
struct student
{
int ID = 0;
string name = "";
long long phone = 0;
string addr = "";
};
int main()
{
const int SIZE = 10;
/// TO DO: Declare a student object array here.
/// Check the size of the array
cout << "The size of the studArr1: " << sizeof(studArr1) << endl;
/// TO DO: Hard-code some data for two students
/// Populate the 4 fields of studArr1[0] with the data: 12345, Amy, 9256776789, "123 Main St, Livermore, CA, 94550"
/// Populate the 4 fields of studArr1[3] with the data: 12666, Bill, 9256776000, "29 Johnson Dr, Livermore, CA, 94550"
cout << "Display the data in studArr1" << endl;
/// TO DO: Use a for loop to display the data of studArr1[0] and studArr1[3]
/// Use one line to display the information of a person
/// TO DO: Declare a student pointer array (Type 2 in the document) here. How can we initialize the pointer with nullptr?
/// Check the size of the array
cout << "The size of the studArr2: " << sizeof(studArr2) << endl;
/// TO DO: Hard-code some data for two students
/// Populate the 4 fields of studArr2[0] with the data: 12345, Amy, 9256776789, "123 Main St, Livermore, CA, 94550"
/// Populate the 4 fields of studArr2[3] with the data: 12666, Bill, 9256776000, "29 Johnson Dr, Livermore, CA, 94550"
cout << "Display the data in studArr2" << endl;
/// TO DO: Use a for loop to display the data of studArr2[0] and studArr2[3]
/// Use one line to display the information of a person
/// TO DO: Release data in studArr1, if needed. Answer the #1 question above.
/// TO DO: Release data in studArr2, if needed. Answer the #2 question above.
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
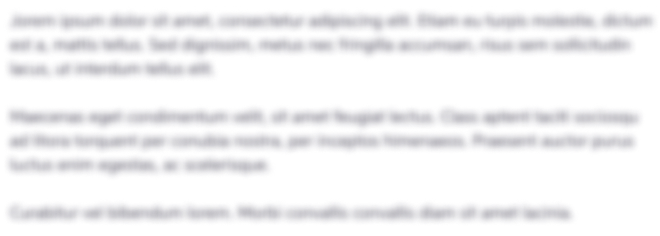
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started