Question
Good evening. I am really struggling with some C++ Code for my computer science class. I have some code written out and need to add
Good evening. I am really struggling with some C++ Code for my computer science class. I have some code written out and need to add more to it. I need to add where it asks for a "Measure Date:" category and then asks the user to enter a month 1-12 as an integer. Enter the day 1-31 as an integer. Enter the year as an integer. And then display the date later.
Here is the code so far:
#include
using std::cin;
using std::cout;
using std::endl;
// Declare a simple structure to hold a date.
struct Date
{
int month;
int day;
int year;
};
// Here are prototypes for access functions associated with Date.
void fillDate(Date&);
void showDate(Date);
// A Date can be used as a component of another structure.
struct Person
{
int height;
int weight;
Date birthday;
};
// Here is a prototype for a function to access a Person
void showPerson(Person);
int main()
{
Date first = { 10,6,2000 }; // Here is initialization on the fly.
Date second; // No initialization, so need to fill later.
cout << endl;
fillDate(second); // Here is initialization with a helper function.
cout << " Printing the dates:" << endl;
showDate(first); // Then display the results for either.
showDate(second);
Person Joe; // Some examples for manipulating a person
Joe.height = 72;
Joe.weight = 180;
cout << endl;
fillDate(Joe.birthday);
cout << endl << " Joe was born in " << Joe.birthday.year << endl;
cout << "Here's Joe:" << endl;
showPerson(Joe);
Person Fred = { 74,200,{ 11,27,1968 } }; // Here's the direct initializer.
cout << " And here is Fred." << endl;
showPerson(Fred);
cout << endl;
return(0);
}
void fillDate(Date& the_date)
{
int tmp;
// Enter the month with some verification of data.
do {
cout << "Enter month as integer 1-12: ";
cin >> tmp;
} while (tmp<1 || tmp>12);
the_date.month = tmp;
// Enter the day, limited verification.
do {
cout << "Enter day as integer 1-31: ";
cin >> tmp;
} while (tmp<1 || tmp>31);
the_date.day = tmp;
cout << "Enter year as integer: ";
cin >> the_date.year;
}
void showDate(Date the_date)
{
cout << the_date.month << "/"
<< the_date.day << "/"
<< the_date.year << endl;
}
void showPerson(Person the_person)
{
cout << "Height is " << the_person.height << endl
<< "Weight is " << the_person.weight << endl
<< "Birthday is ";
showDate(the_person.birthday);
}
AND HERE IS AN EXAMPLE OF WHAT THE CODE NEEDS TO LOOK LIKE:
Enter month as integer 1-12: 3
Enter day as integer 1-31: 21
Enter year as integer: 2002
Printing the dates: 10/6/2000 3/21/2002
Birthday:
Enter month as integer 1-12: 5
Enter day as integer 1-31:
10 Enter year as integer: 1991
Measure date:
Enter month as integer 1-12: 10
Enter day as integer 1-31: 6
Enter year as integer: 1962
Joe was born in 1991
Height is 72
Weight is 180
Last measured on 10/6/1962
Birthday is 5/10/1991
And here is Fred.
Height is 74
Weight is 200
Last measured on 6/21/2007
Birthday is 11/27/1968
Step by Step Solution
There are 3 Steps involved in it
Step: 1
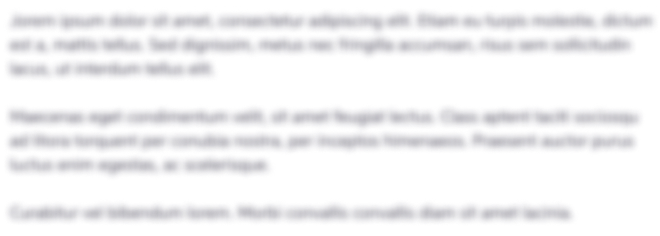
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started