Question
Handler.java: /** * Handler class containing the logic for echoing results back * to the client. */ import java.io.*; import java.net.*; import java.util.*; public class
Handler.java:
/**
* Handler class containing the logic for echoing results back
* to the client.
*/
import java.io.*;
import java.net.*;
import java.util.*;
public class Handler
{
public static final int BUFFER_SIZE = 256;
private BufferedReader in = null;
/**
* this method is invoked by a separate thread
*/
public void process(Socket client) throws IOException {
byte[] buffer = new byte[BUFFER_SIZE];
String message, quote;
InputStream fromClient = null;
PrintWriter toClient = null;
try {
in = new BufferedReader(new FileReader("one-liners.txt"));
} catch (FileNotFoundException e) {
System.err.println("Could not open quote file. Serving time instead.");
}
try {
/**
* get the input and output streams associated with the socket.
*/
fromClient = new BufferedInputStream(client.getInputStream());
toClient = new PrintWriter (client.getOutputStream(), true);
int numBytes;
boolean done = false;
/** continually loop until the client closes the connection */
while ( !done && (numBytes = fromClient.read(buffer)) > 0) {
message = (new String(buffer)).trim();
if (message.equalsIgnoreCase("quote")){
if (in == null)
quote = new Date().toString();
else
quote = getNextQuote ();
toClient.println(quote);
}
else
done = true;
}
}
catch (IOException ioe) {
System.err.println(ioe);
}
finally {
// close streams and socket
if (fromClient != null)
fromClient.close();
if (toClient != null)
toClient.close();
if (client != null)
client.close();
}
}
private String getNextQuote() {
String returnValue = null;
try {
if ((returnValue = in.readLine()) == null) {
in.close();
returnValue = "No more quotes. Goodbye.";
}
} catch (IOException e) {
returnValue = "IOException occurred in server.";
}
return returnValue;
}
}
One-Liners.txt:
Life is wonderful. Without it we'd all be dead. Daddy, why doesn't this magnet pick up this floppy disk? Give me ambiguity or give me something else. I.R.S.: We've got what it takes to take what you've got! We are born naked, wet and hungry. Then things get worse. Make it idiot proof and someone will make a better idiot. He who laughs last thinks slowest! Always remember you're unique, just like everyone else. "More hay, Trigger?" "No thanks, Roy, I'm stuffed!" A flashlight is a case for holding dead batteries. Lottery: A tax on people who are bad at math. Error, no keyboard - press F1 to continue. There's too much blood in my caffeine system. Artificial Intelligence usually beats real stupidity. Hard work has a future payoff. Laziness pays off now. "Very funny, Scotty. Now beam down my clothes." Puritanism: The haunting fear that someone, somewhere may be happy. Consciousness: that annoying time between naps. Don't take life too seriously, you won't get out alive. I don't suffer from insanity. I enjoy every minute of it. Better to understand a little than to misunderstand a lot. The gene pool could use a little chlorine. When there's a will, I want to be in it. Okay, who put a "stop payment" on my reality check? We have enough youth, how about a fountain of SMART? Programming is an art form that fights back. "Daddy, what does FORMATTING DRIVE C mean?" All wiyht. Rho sritched mg kegtops awound? My mail reader can beat up your mail reader. Never forget: 2 + 2 = 5 for extremely large values of 2. Nobody has ever, ever, EVER learned all of WordPerfect. To define recursion, we must first define recursion. Good programming is 99% sweat and 1% coffee. Home is where you hang your @ The E-mail of the species is more deadly than the mail. A journey of a thousand sites begins with a single click. You can't teach a new mouse old clicks. Great groups from little icons grow. Speak softly and carry a cellular phone. C:\ is the root of all directories. Don't put all your hypes in one home page. Pentium wise; pen and paper foolish. The modem is the message. Too many clicks spoil the browse. The geek shall inherit the earth. A chat has nine lives. Don't byte off more than you can view. Fax is stranger than fiction. What boots up must come down. Windows will never cease. (ed. oh sure...) In Gates we trust. (ed. yeah right....) Virtual reality is its own reward. Modulation in all things. A user and his leisure time are soon parted. There's no place like http://www.home.com Know what to expect before you connect. Oh, what a tangled website we weave when first we practice. Speed thrills. Give a man a fish and you feed him for a day; teach him to use the Net and he won't bother you for weeks.
connection.java:
import java.net.*;
import java.io.*;
public class Connection implements Runnable
{
public Connection(Socket client) {
this.client = client;
}
public void run() {
try {
handler.process(client);
}
catch (IOException e) {
e.printStackTrace();
}
}
private Socket client;
private Handler handler = new Handler();
}
QuoteClient.java:
import java.net.*;
import java.io.*;
public class QuoteClient
{
public static final int DEFAULT_PORT = 6007;
public static void main(String[] args) throws IOException {
if (args.length != 1) {
System.err.println("Usage: java EchoClient ");
System.exit(0);
}
BufferedReader networkBin = null; // the reader from the network
PrintWriter networkPout = null; // the writer to the network
BufferedReader localBin = null; // the reader from the local keyboard
Socket sock = null; // the socket
try {
sock = new Socket(args[0], DEFAULT_PORT);
// set up the necessary communication channels
networkBin = new BufferedReader(new InputStreamReader(sock.getInputStream()));
localBin = new BufferedReader(new InputStreamReader(System.in));
/**
* a PrintWriter allows us to use println() with ordinary
* socket I/O. "true" indicates automatic flushing of the stream.
* The stream is flushed with an invocation of println()
*/
networkPout = new PrintWriter(sock.getOutputStream(),true);
/**
* Read from the keyboard and send it to the echo server.
* Quit reading when the client enters a period "."
*/
boolean done = false;
int count = 1;
while (!done) {
String line = localBin.readLine();
if (!line.equalsIgnoreCase("quote"))
done = true;
else {
networkPout.println(line);
System.out.println("Quote #" + count + ": " + networkBin.readLine());
count++;
}
}
}
catch (IOException ioe) {
System.err.println(ioe);
}
finally {
if (networkBin != null)
networkBin.close();
if (localBin != null)
localBin.close();
if (networkPout != null)
networkPout.close();
if (sock != null)
sock.close();
}
}
}
QuoteServer1.java:
import java.net.*;
import java.io.*;
public class QuoteServer1
{
public static final int DEFAULT_PORT = 6007;
public static void main(String[] args) throws IOException {
ServerSocket sock = null;
try {
// establish the socket
sock = new ServerSocket(DEFAULT_PORT);
while (true) {
/**
* now listen for connections
* and service the connection in a separate thread.
*/
Thread worker = new Thread(new Connection(sock.accept()));
worker.start();
}
}
catch (IOException ioe) { }
finally {
if (sock != null)
sock.close();
}
}
}
PARTI Given a client-server network program files (stored in both QuoteServerl and QuoteServer2 folders), the client will produce the results as shown below: General Output -Configuration:Step by Step Solution
There are 3 Steps involved in it
Step: 1
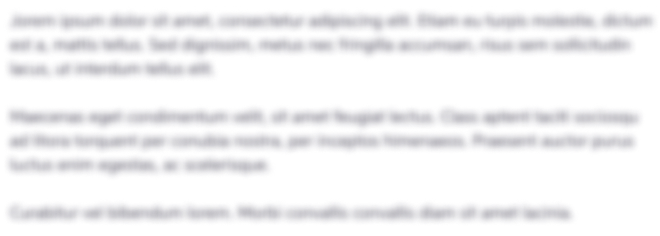
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started