Question
Has to be on g++ compiler Create a program in two modules for a Label Maker application: The Two modules are used as follows to
Has to be on g++ compiler
Create a program in two modules for a Label Maker application:
The Two modules are used as follows to print labels:
Sample main
// Workshop 4: // Version: 0.9 // Date: 2021/02/04 // Author: Fardad Soleimanloo // Description: // This file tests the DIY section of your workshop ///////////////////////////////////////////// #include#include "Label.h" #include "LabelMaker.h" using namespace sdds; using namespace std; int main() { int noOfLabels; // Create a lable for the program title // and couple of empty ones: Label theProgram("/-\\|/-\\|", "The Label Maker Program"), EmptyOne1, EmptyOne2("ABCDEFGH"); theProgram.printLabel() << endl << endl; // ask for number of labels to get created cout << "Number of labels to create: "; cin >> noOfLabels; cin.ignore(10000, ' '); // Create a LabelMaker for the number of // the labels requested LabelMaker lblMkr(noOfLabels); // Ask for the label texts lblMkr.readLabels(); // Print the labels lblMkr.printLabels(); return 0; }
DIY Execution sample
EmptyOne1 EmptyOne2 /-------------------------\ | | | The Label Maker Program | | | \-------------------------/ Number of labels to create: 3 Enter 3 labels: Enter label number 1 > Introduction to Programming Using C Enter label number 2 > Introduction to Object Oriented Programming Enter label number 3 > Object-Oriented Software Development Using C++ +-------------------------------------+ | | | Introduction to Programming Using C | | | +-------------------------------------+ +---------------------------------------------+ | | | Introduction to Object Oriented Programming | | | +---------------------------------------------+ +------------------------------------------------+ | | | Object-Oriented Software Development Using C++ | | | +------------------------------------------------+
The Label Module
The label class creates a label by drawing a frame around a one-line text with an unknown size (maximum of 70 chars,must be kept dynamically).
The frame is dictated by series of 8 characters in a Cstring. These characters indicate how the frame is to be drawn:
character 1: top left corner character. character 2: character to repeat for the top line. character 3: top right corner character character 4: character to repeat for the right line. character 5: bottom right corner character character 6: character to repeat for the bottom line. character 7: bottom left corner character character 8: character to repeat for the left line.
For example the following CString:"AbCdEfGh"
will generate the following frame around a text:
AbbbbbbbbbbbbbbbbbbbbbbbbbC h d h d h d GfffffffffffffffffffffffffE
Mandatory constructors and methods:
Label()
Creates an empty label and defaults the frame to"+-+|+-+|"
Label(const char* frameArg)
Creates an empty label and sets the frame to theframeArgargument.
Label(const char* frameArg, const char* content)
Creates a Label with theframeset toframeArgand the content of the Label set to the corresponding argument. Note that you must keep the content dynamically even though it should never be more than 70 characters.
destructor
Makes sure there is no memory leak when the label goes out of scope.
void readLabel();
Reads the label from console up to 70 characters and stores it in the Label as shown in theExecution sample
std::ostream& printLabel()const;
Prints the label by drawing the frame around the content ad shown in theExecution sample. Note that no newline is printed after the last line and cout is returned at the end.
The LabelMaker Module
The LabelMaker class creates a dynamic array of Labels and gets their content from the user one by one and prints them all at once.
Mandatory constructors and methods:
LabelMaker(int noOfLabels);
creates a dynamic array of Labels to the size ofnoOfLabels
void readLabels();
Gets the contents of the Labels as demonstrated in theExecution sample
void printLabels();
Prints the Labels as demonstrated in theExecution sample
~LabelMaker();
Deallocates the memory when LabelMaker goes out of scope.
Modify the tester program to test are the different circumstances/cases of the application if desired and note that the professor's tester may have many more samples than the tester program here.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
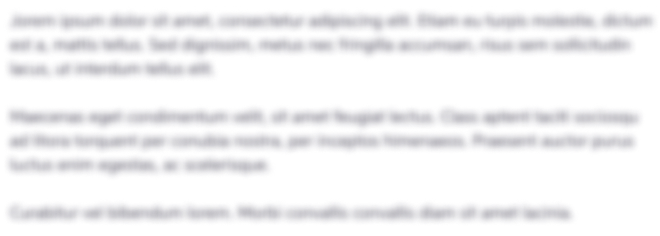
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started