Question
Hash Table java (ONLY EDIT WHERE YOUR CODE HERE IS WRITTEN) IHash has the interface to the hashing implementation, and HashTable is the file with
Hash Table java (ONLY EDIT WHERE "YOUR CODE HERE" IS WRITTEN)
IHash has the interface to the hashing implementation, and HashTable is the file with your implementation. Implement all of the methods in the interface.
Conceptually,
Each hashTable is a table (implemented as an array list) indexed by hashCode % size.
Each entry in the table contains a hashBucket.
You will need to decide which data structure you wish to use for the hashBuckets. Good candidates include an ArrayList or LinkedList.
WARNING | Create a working version of the assignment before trying to optimize |
HashIterator
This is the last part of the HashTable class and will take time to conceptualize.
---------------------------------------------------------------------------------------------
import java.util.*;
public class HashTable implements IHash {
// Method of hashing
HashFunction hasher;
// Hash table : an ArrayList of "buckets", which store the actual strings
ArrayList> hashTable;
/**
* Number of Elements
*/
int numberOfElements;
int size;
/**
* Initializes a new instance of HashTable.
*
* Initialize the instance variables.
* Note: when initializing the hashTable, make sure to allocate each entry in the HashTable
* to a new a HashBucket or null, your choice.
* @param size the size of the hashTable
* @param hasher the type of hashing function
*/
public HashTable(int size, HashFunction hasher) {
// YOUR CODE HERE
}
public boolean insert(String key) {
// YOUR CODE HERE
return false;
}
public boolean remove(String key) {
// YOUR CODE HERE
return false;
}
public String search(String key) {
// YOUR CODE HERE
return null;
}
public int size() {
// YOUR CODE HERE
return size;
}
public int size(int index) {
// YOUR CODE HERE
return 0;
}
// Return iterator for the entire hashTable,
// must iterate all hashBuckets that are not empty
public class HashIterator implements Iterator
// The current index into the hashTable
private int currentIndex;
// The current iterator for that hashBucket
private Iterator
HashIterator() {
// YOUR CODE HERE
}
public String next() {
// YOUR CODE HERE
return null;
}
public boolean hasNext() {
// YOUR CODE HERE
return false;
}
}
// Return an iterator for the hash table
public Iterator
// YOUR CODE HERE
return null;
}
/**
* Does not use the iterator above. Iterates over one bucket.
*
* @param index the index of bucket to iterate over
* @return an iterator for that bucket
*/
public Iterator
List
return bucket != null ? bucket.iterator() : null;
}
// Prints entire hash table.
// NOTE: This method is used extensively for testing.
public void print() {
Debug.printf("HashTable size: " + size());
for (int index = 0; index
List
if (list != null) {
Debug.printf("HashTable[%d]: %d entries", index, list.size());
for (String word : list) {
Debug.printf("String: %s (hash code %d)", word, hasher.hash(word));
}
}else {
Debug.printf("HashTable[%d]: %d entries", index, 0);
}
}
}
}
Constructor Detail HashTable public HashTable(int size, HashFunction hasher) Initializes a new instance of HashTable. Initialize the instance variables. Note: when initializing the hashTable, make sure to allocate each entry in the HashTable to a new a HashBucket or null, your choice. Parameters size -the size of the hashTable hasher- the type of hashing function Constructor Detail HashTable public HashTable(int size, HashFunction hasher) Initializes a new instance of HashTable. Initialize the instance variables. Note: when initializing the hashTable, make sure to allocate each entry in the HashTable to a new a HashBucket or null, your choice. Parameters size -the size of the hashTable hasher- the type of hashing functionStep by Step Solution
There are 3 Steps involved in it
Step: 1
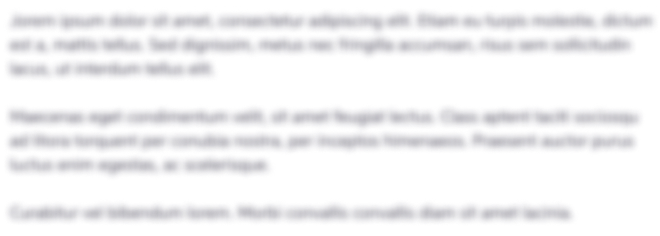
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started