Question
Header files : card.h: #ifndef CARD_H #define CARD_H #include using std::ostream; // Enum type that represents the card suits enum suit {clubs, hearts, spades, diamonds};
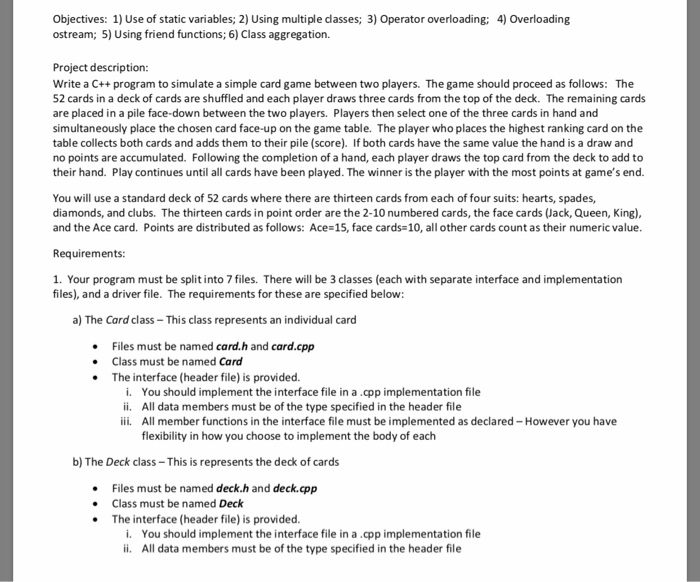
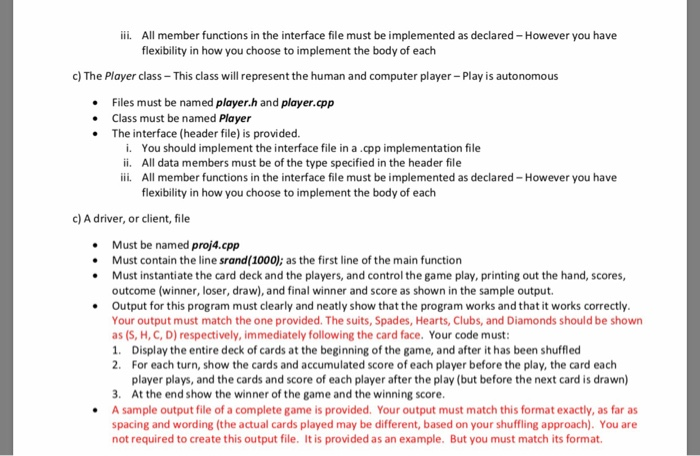
#ifndef CARD_H
#define CARD_H
#include
using std::ostream;
// Enum type that represents the card suits
enum suit {clubs, hearts, spades, diamonds};
class Card
{
public:
//default constructor - required
Card();
//constructor that takes a card's face (represented an integer) and suit
// card face example: Ace=0, 2=1, 3=2, ... Q=11, K=12 or some other ordering
Card (int face, suit st);
// overload the
friend ostream& operator
// compare and return true if *this has a lesser point value than cd, false otherwise
bool operator
// compare and return true if *this has a larger point value than cd, false otherwise
bool operator > (const Card& cd) const;
// compare and return true if *this has the same point value as cd, false otherwise
bool operator== (const Card& cd) const;
// return the point value of the card: Ace: 15, Faces: 10, Numbers: the number
int getPointValue() const;
private:
suit cardSuit; // card's suit
int cardFace; // card's face
int pointValue; // card's point value (derived from face)
};
#endif
player.h:
#ifndef PLAYER_H
#define PLAYER_H
#include
#include
#include "deck.h"
#include "card.h"
using std::ostream;
using std::string;
class Player
{
public:
static const int Max_Cards = 3; // # of cards a player can have in a hand
// constructor - player's name defaults to "unknown" if not supplied
Player(string name="unknown");
// Simulates player removing one card from hand and playing it - returns the card
// You may use whatever strategy you'd like here: choose a card randomly,
// choose the card with the largest value, choose the first card, or other approaches
Card playCard();
// draw top card from the deck
void drawCard(Deck& dk);
// add the point value of the card to the player's score
void addScore(Card acard);
// return the score the player has earned so far
int getScore() const;
// return the name of the player
string getName() const;
// return true if the player's hand is out of cards
bool emptyHand() const;
// overload the
friend std::ostream& operator
private:
string name; // the player's name
int score; // the player's score
Card hand[Max_Cards]; // array holding the cards currently in the player's hand
bool hasPlayed[Max_Cards]; // hasPlayed[i] indicates that hand[i] (i.e., ith card in player's hand)
// has been played
};
#endif
deck.h:
#ifndef DECK_H
#define DECK_H
#include
#include "card.h"
using std::ostream;
class Deck
{
public:
// default constructor
Deck();
// Remove the top card from the deck and return it.
Card dealCard();
// Shuffle the cards in the deck
void Shuffle();
// return true if there are no more cards in the deck, false otherwise
bool isEmpty();
//overload
friend ostream& operator
private:
static const int numCards = 52; // # of cards in a deck
Card theDeck[numCards]; // the array holding the cards
int topCard; // the index of the deck's top card
};
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
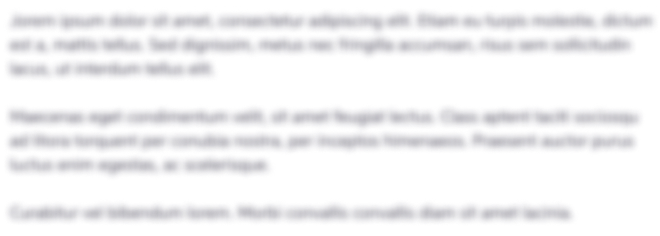
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started